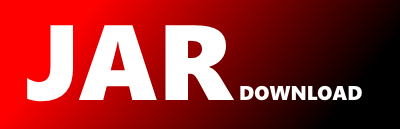
com.pulumi.googlenative.compute.alpha.inputs.ClientTlsSettingsArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.compute.alpha.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.compute.alpha.enums.ClientTlsSettingsMode;
import com.pulumi.googlenative.compute.alpha.inputs.TlsContextArgs;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* [Deprecated] The client side authentication settings for connection originating from the backend service. the backend service.
*
*/
public final class ClientTlsSettingsArgs extends com.pulumi.resources.ResourceArgs {
public static final ClientTlsSettingsArgs Empty = new ClientTlsSettingsArgs();
/**
* Configures the mechanism to obtain client-side security certificates and identity information. This field is only applicable when mode is set to MUTUAL.
*
*/
@Import(name="clientTlsContext")
private @Nullable Output clientTlsContext;
/**
* @return Configures the mechanism to obtain client-side security certificates and identity information. This field is only applicable when mode is set to MUTUAL.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy