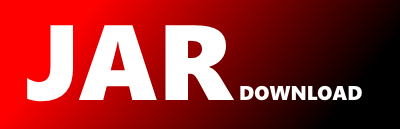
com.pulumi.googlenative.compute.alpha.outputs.GetExternalVpnGatewayResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.compute.alpha.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.compute.alpha.outputs.ExternalVpnGatewayInterfaceResponse;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetExternalVpnGatewayResult {
/**
* @return Creation timestamp in RFC3339 text format.
*
*/
private String creationTimestamp;
/**
* @return An optional description of this resource. Provide this property when you create the resource.
*
*/
private String description;
/**
* @return A list of interfaces for this external VPN gateway. If your peer-side gateway is an on-premises gateway and non-AWS cloud providers' gateway, at most two interfaces can be provided for an external VPN gateway. If your peer side is an AWS virtual private gateway, four interfaces should be provided for an external VPN gateway.
*
*/
private List interfaces;
/**
* @return Type of the resource. Always compute#externalVpnGateway for externalVpnGateways.
*
*/
private String kind;
/**
* @return A fingerprint for the labels being applied to this ExternalVpnGateway, which is essentially a hash of the labels set used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve an ExternalVpnGateway.
*
*/
private String labelFingerprint;
/**
* @return Labels for this resource. These can only be added or modified by the setLabels method. Each label key/value pair must comply with RFC1035. Label values may be empty.
*
*/
private Map labels;
/**
* @return Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*/
private String name;
/**
* @return Indicates the user-supplied redundancy type of this external VPN gateway.
*
*/
private String redundancyType;
/**
* @return Server-defined URL for the resource.
*
*/
private String selfLink;
private GetExternalVpnGatewayResult() {}
/**
* @return Creation timestamp in RFC3339 text format.
*
*/
public String creationTimestamp() {
return this.creationTimestamp;
}
/**
* @return An optional description of this resource. Provide this property when you create the resource.
*
*/
public String description() {
return this.description;
}
/**
* @return A list of interfaces for this external VPN gateway. If your peer-side gateway is an on-premises gateway and non-AWS cloud providers' gateway, at most two interfaces can be provided for an external VPN gateway. If your peer side is an AWS virtual private gateway, four interfaces should be provided for an external VPN gateway.
*
*/
public List interfaces() {
return this.interfaces;
}
/**
* @return Type of the resource. Always compute#externalVpnGateway for externalVpnGateways.
*
*/
public String kind() {
return this.kind;
}
/**
* @return A fingerprint for the labels being applied to this ExternalVpnGateway, which is essentially a hash of the labels set used for optimistic locking. The fingerprint is initially generated by Compute Engine and changes after every request to modify or update labels. You must always provide an up-to-date fingerprint hash in order to update or change labels, otherwise the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve an ExternalVpnGateway.
*
*/
public String labelFingerprint() {
return this.labelFingerprint;
}
/**
* @return Labels for this resource. These can only be added or modified by the setLabels method. Each label key/value pair must comply with RFC1035. Label values may be empty.
*
*/
public Map labels() {
return this.labels;
}
/**
* @return Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*/
public String name() {
return this.name;
}
/**
* @return Indicates the user-supplied redundancy type of this external VPN gateway.
*
*/
public String redundancyType() {
return this.redundancyType;
}
/**
* @return Server-defined URL for the resource.
*
*/
public String selfLink() {
return this.selfLink;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetExternalVpnGatewayResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String creationTimestamp;
private String description;
private List interfaces;
private String kind;
private String labelFingerprint;
private Map labels;
private String name;
private String redundancyType;
private String selfLink;
public Builder() {}
public Builder(GetExternalVpnGatewayResult defaults) {
Objects.requireNonNull(defaults);
this.creationTimestamp = defaults.creationTimestamp;
this.description = defaults.description;
this.interfaces = defaults.interfaces;
this.kind = defaults.kind;
this.labelFingerprint = defaults.labelFingerprint;
this.labels = defaults.labels;
this.name = defaults.name;
this.redundancyType = defaults.redundancyType;
this.selfLink = defaults.selfLink;
}
@CustomType.Setter
public Builder creationTimestamp(String creationTimestamp) {
this.creationTimestamp = Objects.requireNonNull(creationTimestamp);
return this;
}
@CustomType.Setter
public Builder description(String description) {
this.description = Objects.requireNonNull(description);
return this;
}
@CustomType.Setter
public Builder interfaces(List interfaces) {
this.interfaces = Objects.requireNonNull(interfaces);
return this;
}
public Builder interfaces(ExternalVpnGatewayInterfaceResponse... interfaces) {
return interfaces(List.of(interfaces));
}
@CustomType.Setter
public Builder kind(String kind) {
this.kind = Objects.requireNonNull(kind);
return this;
}
@CustomType.Setter
public Builder labelFingerprint(String labelFingerprint) {
this.labelFingerprint = Objects.requireNonNull(labelFingerprint);
return this;
}
@CustomType.Setter
public Builder labels(Map labels) {
this.labels = Objects.requireNonNull(labels);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder redundancyType(String redundancyType) {
this.redundancyType = Objects.requireNonNull(redundancyType);
return this;
}
@CustomType.Setter
public Builder selfLink(String selfLink) {
this.selfLink = Objects.requireNonNull(selfLink);
return this;
}
public GetExternalVpnGatewayResult build() {
final var o = new GetExternalVpnGatewayResult();
o.creationTimestamp = creationTimestamp;
o.description = description;
o.interfaces = interfaces;
o.kind = kind;
o.labelFingerprint = labelFingerprint;
o.labels = labels;
o.name = name;
o.redundancyType = redundancyType;
o.selfLink = selfLink;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy