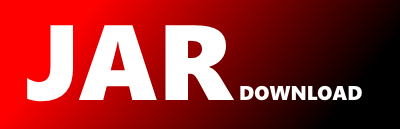
com.pulumi.googlenative.compute.beta.TargetSslProxy Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.compute.beta;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.googlenative.Utilities;
import com.pulumi.googlenative.compute.beta.TargetSslProxyArgs;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Creates a TargetSslProxy resource in the specified project using the data included in the request.
*
*/
@ResourceType(type="google-native:compute/beta:TargetSslProxy")
public class TargetSslProxy extends com.pulumi.resources.CustomResource {
/**
* URL of a certificate map that identifies a certificate map associated with the given target proxy. This field can only be set for global target proxies. If set, sslCertificates will be ignored. Accepted format is //certificatemanager.googleapis.com/projects/{project }/locations/{location}/certificateMaps/{resourceName}.
*
*/
@Export(name="certificateMap", type=String.class, parameters={})
private Output certificateMap;
/**
* @return URL of a certificate map that identifies a certificate map associated with the given target proxy. This field can only be set for global target proxies. If set, sslCertificates will be ignored. Accepted format is //certificatemanager.googleapis.com/projects/{project }/locations/{location}/certificateMaps/{resourceName}.
*
*/
public Output certificateMap() {
return this.certificateMap;
}
/**
* Creation timestamp in RFC3339 text format.
*
*/
@Export(name="creationTimestamp", type=String.class, parameters={})
private Output creationTimestamp;
/**
* @return Creation timestamp in RFC3339 text format.
*
*/
public Output creationTimestamp() {
return this.creationTimestamp;
}
/**
* An optional description of this resource. Provide this property when you create the resource.
*
*/
@Export(name="description", type=String.class, parameters={})
private Output description;
/**
* @return An optional description of this resource. Provide this property when you create the resource.
*
*/
public Output description() {
return this.description;
}
/**
* Type of the resource. Always compute#targetSslProxy for target SSL proxies.
*
*/
@Export(name="kind", type=String.class, parameters={})
private Output kind;
/**
* @return Type of the resource. Always compute#targetSslProxy for target SSL proxies.
*
*/
public Output kind() {
return this.kind;
}
/**
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*/
@Export(name="name", type=String.class, parameters={})
private Output name;
/**
* @return Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*/
public Output name() {
return this.name;
}
@Export(name="project", type=String.class, parameters={})
private Output project;
public Output project() {
return this.project;
}
/**
* Specifies the type of proxy header to append before sending data to the backend, either NONE or PROXY_V1. The default is NONE.
*
*/
@Export(name="proxyHeader", type=String.class, parameters={})
private Output proxyHeader;
/**
* @return Specifies the type of proxy header to append before sending data to the backend, either NONE or PROXY_V1. The default is NONE.
*
*/
public Output proxyHeader() {
return this.proxyHeader;
}
/**
* An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*
*/
@Export(name="requestId", type=String.class, parameters={})
private Output* @Nullable */ String> requestId;
/**
* @return An optional request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported ( 00000000-0000-0000-0000-000000000000).
*
*/
public Output> requestId() {
return Codegen.optional(this.requestId);
}
/**
* Server-defined URL for the resource.
*
*/
@Export(name="selfLink", type=String.class, parameters={})
private Output selfLink;
/**
* @return Server-defined URL for the resource.
*
*/
public Output selfLink() {
return this.selfLink;
}
/**
* URL to the BackendService resource.
*
*/
@Export(name="service", type=String.class, parameters={})
private Output service;
/**
* @return URL to the BackendService resource.
*
*/
public Output service() {
return this.service;
}
/**
* URLs to SslCertificate resources that are used to authenticate connections to Backends. At least one SSL certificate must be specified. Currently, you may specify up to 15 SSL certificates. sslCertificates do not apply when the load balancing scheme is set to INTERNAL_SELF_MANAGED.
*
*/
@Export(name="sslCertificates", type=List.class, parameters={String.class})
private Output> sslCertificates;
/**
* @return URLs to SslCertificate resources that are used to authenticate connections to Backends. At least one SSL certificate must be specified. Currently, you may specify up to 15 SSL certificates. sslCertificates do not apply when the load balancing scheme is set to INTERNAL_SELF_MANAGED.
*
*/
public Output> sslCertificates() {
return this.sslCertificates;
}
/**
* URL of SslPolicy resource that will be associated with the TargetSslProxy resource. If not set, the TargetSslProxy resource will not have any SSL policy configured.
*
*/
@Export(name="sslPolicy", type=String.class, parameters={})
private Output sslPolicy;
/**
* @return URL of SslPolicy resource that will be associated with the TargetSslProxy resource. If not set, the TargetSslProxy resource will not have any SSL policy configured.
*
*/
public Output sslPolicy() {
return this.sslPolicy;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public TargetSslProxy(String name) {
this(name, TargetSslProxyArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public TargetSslProxy(String name, @Nullable TargetSslProxyArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public TargetSslProxy(String name, @Nullable TargetSslProxyArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:compute/beta:TargetSslProxy", name, args == null ? TargetSslProxyArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private TargetSslProxy(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:compute/beta:TargetSslProxy", name, null, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static TargetSslProxy get(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new TargetSslProxy(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy