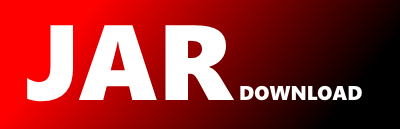
com.pulumi.googlenative.compute.beta.inputs.ResourcePolicyInstanceSchedulePolicyArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.compute.beta.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.compute.beta.inputs.ResourcePolicyInstanceSchedulePolicyScheduleArgs;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* An InstanceSchedulePolicy specifies when and how frequent certain operations are performed on the instance.
*
*/
public final class ResourcePolicyInstanceSchedulePolicyArgs extends com.pulumi.resources.ResourceArgs {
public static final ResourcePolicyInstanceSchedulePolicyArgs Empty = new ResourcePolicyInstanceSchedulePolicyArgs();
/**
* The expiration time of the schedule. The timestamp is an RFC3339 string.
*
*/
@Import(name="expirationTime")
private @Nullable Output expirationTime;
/**
* @return The expiration time of the schedule. The timestamp is an RFC3339 string.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy