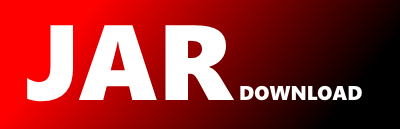
com.pulumi.googlenative.compute.v1.outputs.InstanceGroupManagerActionsSummaryResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.compute.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.util.Objects;
@CustomType
public final class InstanceGroupManagerActionsSummaryResponse {
/**
* @return The total number of instances in the managed instance group that are scheduled to be abandoned. Abandoning an instance removes it from the managed instance group without deleting it.
*
*/
private Integer abandoning;
/**
* @return The number of instances in the managed instance group that are scheduled to be created or are currently being created. If the group fails to create any of these instances, it tries again until it creates the instance successfully. If you have disabled creation retries, this field will not be populated; instead, the creatingWithoutRetries field will be populated.
*
*/
private Integer creating;
/**
* @return The number of instances that the managed instance group will attempt to create. The group attempts to create each instance only once. If the group fails to create any of these instances, it decreases the group's targetSize value accordingly.
*
*/
private Integer creatingWithoutRetries;
/**
* @return The number of instances in the managed instance group that are scheduled to be deleted or are currently being deleted.
*
*/
private Integer deleting;
/**
* @return The number of instances in the managed instance group that are running and have no scheduled actions.
*
*/
private Integer none;
/**
* @return The number of instances in the managed instance group that are scheduled to be recreated or are currently being being recreated. Recreating an instance deletes the existing root persistent disk and creates a new disk from the image that is defined in the instance template.
*
*/
private Integer recreating;
/**
* @return The number of instances in the managed instance group that are being reconfigured with properties that do not require a restart or a recreate action. For example, setting or removing target pools for the instance.
*
*/
private Integer refreshing;
/**
* @return The number of instances in the managed instance group that are scheduled to be restarted or are currently being restarted.
*
*/
private Integer restarting;
/**
* @return The number of instances in the managed instance group that are scheduled to be resumed or are currently being resumed.
*
*/
private Integer resuming;
/**
* @return The number of instances in the managed instance group that are scheduled to be started or are currently being started.
*
*/
private Integer starting;
/**
* @return The number of instances in the managed instance group that are scheduled to be stopped or are currently being stopped.
*
*/
private Integer stopping;
/**
* @return The number of instances in the managed instance group that are scheduled to be suspended or are currently being suspended.
*
*/
private Integer suspending;
/**
* @return The number of instances in the managed instance group that are being verified. See the managedInstances[].currentAction property in the listManagedInstances method documentation.
*
*/
private Integer verifying;
private InstanceGroupManagerActionsSummaryResponse() {}
/**
* @return The total number of instances in the managed instance group that are scheduled to be abandoned. Abandoning an instance removes it from the managed instance group without deleting it.
*
*/
public Integer abandoning() {
return this.abandoning;
}
/**
* @return The number of instances in the managed instance group that are scheduled to be created or are currently being created. If the group fails to create any of these instances, it tries again until it creates the instance successfully. If you have disabled creation retries, this field will not be populated; instead, the creatingWithoutRetries field will be populated.
*
*/
public Integer creating() {
return this.creating;
}
/**
* @return The number of instances that the managed instance group will attempt to create. The group attempts to create each instance only once. If the group fails to create any of these instances, it decreases the group's targetSize value accordingly.
*
*/
public Integer creatingWithoutRetries() {
return this.creatingWithoutRetries;
}
/**
* @return The number of instances in the managed instance group that are scheduled to be deleted or are currently being deleted.
*
*/
public Integer deleting() {
return this.deleting;
}
/**
* @return The number of instances in the managed instance group that are running and have no scheduled actions.
*
*/
public Integer none() {
return this.none;
}
/**
* @return The number of instances in the managed instance group that are scheduled to be recreated or are currently being being recreated. Recreating an instance deletes the existing root persistent disk and creates a new disk from the image that is defined in the instance template.
*
*/
public Integer recreating() {
return this.recreating;
}
/**
* @return The number of instances in the managed instance group that are being reconfigured with properties that do not require a restart or a recreate action. For example, setting or removing target pools for the instance.
*
*/
public Integer refreshing() {
return this.refreshing;
}
/**
* @return The number of instances in the managed instance group that are scheduled to be restarted or are currently being restarted.
*
*/
public Integer restarting() {
return this.restarting;
}
/**
* @return The number of instances in the managed instance group that are scheduled to be resumed or are currently being resumed.
*
*/
public Integer resuming() {
return this.resuming;
}
/**
* @return The number of instances in the managed instance group that are scheduled to be started or are currently being started.
*
*/
public Integer starting() {
return this.starting;
}
/**
* @return The number of instances in the managed instance group that are scheduled to be stopped or are currently being stopped.
*
*/
public Integer stopping() {
return this.stopping;
}
/**
* @return The number of instances in the managed instance group that are scheduled to be suspended or are currently being suspended.
*
*/
public Integer suspending() {
return this.suspending;
}
/**
* @return The number of instances in the managed instance group that are being verified. See the managedInstances[].currentAction property in the listManagedInstances method documentation.
*
*/
public Integer verifying() {
return this.verifying;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InstanceGroupManagerActionsSummaryResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Integer abandoning;
private Integer creating;
private Integer creatingWithoutRetries;
private Integer deleting;
private Integer none;
private Integer recreating;
private Integer refreshing;
private Integer restarting;
private Integer resuming;
private Integer starting;
private Integer stopping;
private Integer suspending;
private Integer verifying;
public Builder() {}
public Builder(InstanceGroupManagerActionsSummaryResponse defaults) {
Objects.requireNonNull(defaults);
this.abandoning = defaults.abandoning;
this.creating = defaults.creating;
this.creatingWithoutRetries = defaults.creatingWithoutRetries;
this.deleting = defaults.deleting;
this.none = defaults.none;
this.recreating = defaults.recreating;
this.refreshing = defaults.refreshing;
this.restarting = defaults.restarting;
this.resuming = defaults.resuming;
this.starting = defaults.starting;
this.stopping = defaults.stopping;
this.suspending = defaults.suspending;
this.verifying = defaults.verifying;
}
@CustomType.Setter
public Builder abandoning(Integer abandoning) {
this.abandoning = Objects.requireNonNull(abandoning);
return this;
}
@CustomType.Setter
public Builder creating(Integer creating) {
this.creating = Objects.requireNonNull(creating);
return this;
}
@CustomType.Setter
public Builder creatingWithoutRetries(Integer creatingWithoutRetries) {
this.creatingWithoutRetries = Objects.requireNonNull(creatingWithoutRetries);
return this;
}
@CustomType.Setter
public Builder deleting(Integer deleting) {
this.deleting = Objects.requireNonNull(deleting);
return this;
}
@CustomType.Setter
public Builder none(Integer none) {
this.none = Objects.requireNonNull(none);
return this;
}
@CustomType.Setter
public Builder recreating(Integer recreating) {
this.recreating = Objects.requireNonNull(recreating);
return this;
}
@CustomType.Setter
public Builder refreshing(Integer refreshing) {
this.refreshing = Objects.requireNonNull(refreshing);
return this;
}
@CustomType.Setter
public Builder restarting(Integer restarting) {
this.restarting = Objects.requireNonNull(restarting);
return this;
}
@CustomType.Setter
public Builder resuming(Integer resuming) {
this.resuming = Objects.requireNonNull(resuming);
return this;
}
@CustomType.Setter
public Builder starting(Integer starting) {
this.starting = Objects.requireNonNull(starting);
return this;
}
@CustomType.Setter
public Builder stopping(Integer stopping) {
this.stopping = Objects.requireNonNull(stopping);
return this;
}
@CustomType.Setter
public Builder suspending(Integer suspending) {
this.suspending = Objects.requireNonNull(suspending);
return this;
}
@CustomType.Setter
public Builder verifying(Integer verifying) {
this.verifying = Objects.requireNonNull(verifying);
return this;
}
public InstanceGroupManagerActionsSummaryResponse build() {
final var o = new InstanceGroupManagerActionsSummaryResponse();
o.abandoning = abandoning;
o.creating = creating;
o.creatingWithoutRetries = creatingWithoutRetries;
o.deleting = deleting;
o.none = none;
o.recreating = recreating;
o.refreshing = refreshing;
o.restarting = restarting;
o.resuming = resuming;
o.starting = starting;
o.stopping = stopping;
o.suspending = suspending;
o.verifying = verifying;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy