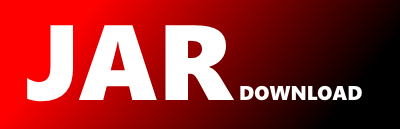
com.pulumi.googlenative.container.v1.NodePoolArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.container.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.container.v1.inputs.MaxPodsConstraintArgs;
import com.pulumi.googlenative.container.v1.inputs.NodeConfigArgs;
import com.pulumi.googlenative.container.v1.inputs.NodeManagementArgs;
import com.pulumi.googlenative.container.v1.inputs.NodeNetworkConfigArgs;
import com.pulumi.googlenative.container.v1.inputs.NodePoolAutoscalingArgs;
import com.pulumi.googlenative.container.v1.inputs.PlacementPolicyArgs;
import com.pulumi.googlenative.container.v1.inputs.StatusConditionArgs;
import com.pulumi.googlenative.container.v1.inputs.UpgradeSettingsArgs;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class NodePoolArgs extends com.pulumi.resources.ResourceArgs {
public static final NodePoolArgs Empty = new NodePoolArgs();
/**
* Autoscaler configuration for this NodePool. Autoscaler is enabled only if a valid configuration is present.
*
*/
@Import(name="autoscaling")
private @Nullable Output autoscaling;
/**
* @return Autoscaler configuration for this NodePool. Autoscaler is enabled only if a valid configuration is present.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy