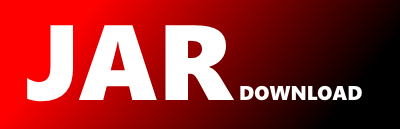
com.pulumi.googlenative.dataflow.v1b3.outputs.WorkerSettingsResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.dataflow.v1b3.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class WorkerSettingsResponse {
/**
* @return The base URL for accessing Google Cloud APIs. When workers access Google Cloud APIs, they logically do so via relative URLs. If this field is specified, it supplies the base URL to use for resolving these relative URLs. The normative algorithm used is defined by RFC 1808, "Relative Uniform Resource Locators". If not specified, the default value is "http://www.googleapis.com/"
*
*/
private String baseUrl;
/**
* @return Whether to send work progress updates to the service.
*
*/
private Boolean reportingEnabled;
/**
* @return The Cloud Dataflow service path relative to the root URL, for example, "dataflow/v1b3/projects".
*
*/
private String servicePath;
/**
* @return The Shuffle service path relative to the root URL, for example, "shuffle/v1beta1".
*
*/
private String shuffleServicePath;
/**
* @return The prefix of the resources the system should use for temporary storage. The supported resource type is: Google Cloud Storage: storage.googleapis.com/{bucket}/{object} bucket.storage.googleapis.com/{object}
*
*/
private String tempStoragePrefix;
/**
* @return The ID of the worker running this pipeline.
*
*/
private String workerId;
private WorkerSettingsResponse() {}
/**
* @return The base URL for accessing Google Cloud APIs. When workers access Google Cloud APIs, they logically do so via relative URLs. If this field is specified, it supplies the base URL to use for resolving these relative URLs. The normative algorithm used is defined by RFC 1808, "Relative Uniform Resource Locators". If not specified, the default value is "http://www.googleapis.com/"
*
*/
public String baseUrl() {
return this.baseUrl;
}
/**
* @return Whether to send work progress updates to the service.
*
*/
public Boolean reportingEnabled() {
return this.reportingEnabled;
}
/**
* @return The Cloud Dataflow service path relative to the root URL, for example, "dataflow/v1b3/projects".
*
*/
public String servicePath() {
return this.servicePath;
}
/**
* @return The Shuffle service path relative to the root URL, for example, "shuffle/v1beta1".
*
*/
public String shuffleServicePath() {
return this.shuffleServicePath;
}
/**
* @return The prefix of the resources the system should use for temporary storage. The supported resource type is: Google Cloud Storage: storage.googleapis.com/{bucket}/{object} bucket.storage.googleapis.com/{object}
*
*/
public String tempStoragePrefix() {
return this.tempStoragePrefix;
}
/**
* @return The ID of the worker running this pipeline.
*
*/
public String workerId() {
return this.workerId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(WorkerSettingsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String baseUrl;
private Boolean reportingEnabled;
private String servicePath;
private String shuffleServicePath;
private String tempStoragePrefix;
private String workerId;
public Builder() {}
public Builder(WorkerSettingsResponse defaults) {
Objects.requireNonNull(defaults);
this.baseUrl = defaults.baseUrl;
this.reportingEnabled = defaults.reportingEnabled;
this.servicePath = defaults.servicePath;
this.shuffleServicePath = defaults.shuffleServicePath;
this.tempStoragePrefix = defaults.tempStoragePrefix;
this.workerId = defaults.workerId;
}
@CustomType.Setter
public Builder baseUrl(String baseUrl) {
this.baseUrl = Objects.requireNonNull(baseUrl);
return this;
}
@CustomType.Setter
public Builder reportingEnabled(Boolean reportingEnabled) {
this.reportingEnabled = Objects.requireNonNull(reportingEnabled);
return this;
}
@CustomType.Setter
public Builder servicePath(String servicePath) {
this.servicePath = Objects.requireNonNull(servicePath);
return this;
}
@CustomType.Setter
public Builder shuffleServicePath(String shuffleServicePath) {
this.shuffleServicePath = Objects.requireNonNull(shuffleServicePath);
return this;
}
@CustomType.Setter
public Builder tempStoragePrefix(String tempStoragePrefix) {
this.tempStoragePrefix = Objects.requireNonNull(tempStoragePrefix);
return this;
}
@CustomType.Setter
public Builder workerId(String workerId) {
this.workerId = Objects.requireNonNull(workerId);
return this;
}
public WorkerSettingsResponse build() {
final var o = new WorkerSettingsResponse();
o.baseUrl = baseUrl;
o.reportingEnabled = reportingEnabled;
o.servicePath = servicePath;
o.shuffleServicePath = shuffleServicePath;
o.tempStoragePrefix = tempStoragePrefix;
o.workerId = workerId;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy