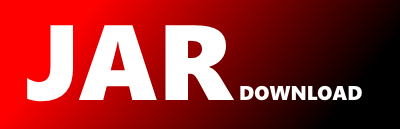
com.pulumi.googlenative.datafusion.v1.InstanceArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.datafusion.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.datafusion.v1.enums.InstanceType;
import com.pulumi.googlenative.datafusion.v1.inputs.CryptoKeyConfigArgs;
import com.pulumi.googlenative.datafusion.v1.inputs.EventPublishConfigArgs;
import com.pulumi.googlenative.datafusion.v1.inputs.NetworkConfigArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class InstanceArgs extends com.pulumi.resources.ResourceArgs {
public static final InstanceArgs Empty = new InstanceArgs();
/**
* The crypto key configuration. This field is used by the Customer-Managed Encryption Keys (CMEK) feature.
*
*/
@Import(name="cryptoKeyConfig")
private @Nullable Output cryptoKeyConfig;
/**
* @return The crypto key configuration. This field is used by the Customer-Managed Encryption Keys (CMEK) feature.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy