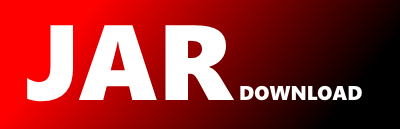
com.pulumi.googlenative.datalabeling.v1beta1.inputs.GoogleCloudDatalabelingV1beta1HumanAnnotationConfigArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.datalabeling.v1beta1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Configuration for how human labeling task should be done.
*
*/
public final class GoogleCloudDatalabelingV1beta1HumanAnnotationConfigArgs extends com.pulumi.resources.ResourceArgs {
public static final GoogleCloudDatalabelingV1beta1HumanAnnotationConfigArgs Empty = new GoogleCloudDatalabelingV1beta1HumanAnnotationConfigArgs();
/**
* Optional. A human-readable description for AnnotatedDataset. The description can be up to 10000 characters long.
*
*/
@Import(name="annotatedDatasetDescription")
private @Nullable Output annotatedDatasetDescription;
/**
* @return Optional. A human-readable description for AnnotatedDataset. The description can be up to 10000 characters long.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy