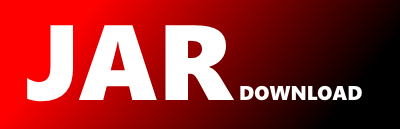
com.pulumi.googlenative.dataplex.v1.outputs.GetContentResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.dataplex.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.dataplex.v1.outputs.GoogleCloudDataplexV1ContentNotebookResponse;
import com.pulumi.googlenative.dataplex.v1.outputs.GoogleCloudDataplexV1ContentSqlScriptResponse;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetContentResult {
/**
* @return Content creation time.
*
*/
private String createTime;
/**
* @return Content data in string format.
*
*/
private String dataText;
/**
* @return Optional. Description of the content.
*
*/
private String description;
/**
* @return Optional. User defined labels for the content.
*
*/
private Map labels;
/**
* @return The relative resource name of the content, of the form: projects/{project_id}/locations/{location_id}/lakes/{lake_id}/content/{content_id}
*
*/
private String name;
/**
* @return Notebook related configurations.
*
*/
private GoogleCloudDataplexV1ContentNotebookResponse notebook;
/**
* @return The path for the Content file, represented as directory structure. Unique within a lake. Limited to alphanumerics, hyphens, underscores, dots and slashes.
*
*/
private String path;
/**
* @return Sql Script related configurations.
*
*/
private GoogleCloudDataplexV1ContentSqlScriptResponse sqlScript;
/**
* @return System generated globally unique ID for the content. This ID will be different if the content is deleted and re-created with the same name.
*
*/
private String uid;
/**
* @return The time when the content was last updated.
*
*/
private String updateTime;
private GetContentResult() {}
/**
* @return Content creation time.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return Content data in string format.
*
*/
public String dataText() {
return this.dataText;
}
/**
* @return Optional. Description of the content.
*
*/
public String description() {
return this.description;
}
/**
* @return Optional. User defined labels for the content.
*
*/
public Map labels() {
return this.labels;
}
/**
* @return The relative resource name of the content, of the form: projects/{project_id}/locations/{location_id}/lakes/{lake_id}/content/{content_id}
*
*/
public String name() {
return this.name;
}
/**
* @return Notebook related configurations.
*
*/
public GoogleCloudDataplexV1ContentNotebookResponse notebook() {
return this.notebook;
}
/**
* @return The path for the Content file, represented as directory structure. Unique within a lake. Limited to alphanumerics, hyphens, underscores, dots and slashes.
*
*/
public String path() {
return this.path;
}
/**
* @return Sql Script related configurations.
*
*/
public GoogleCloudDataplexV1ContentSqlScriptResponse sqlScript() {
return this.sqlScript;
}
/**
* @return System generated globally unique ID for the content. This ID will be different if the content is deleted and re-created with the same name.
*
*/
public String uid() {
return this.uid;
}
/**
* @return The time when the content was last updated.
*
*/
public String updateTime() {
return this.updateTime;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetContentResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String createTime;
private String dataText;
private String description;
private Map labels;
private String name;
private GoogleCloudDataplexV1ContentNotebookResponse notebook;
private String path;
private GoogleCloudDataplexV1ContentSqlScriptResponse sqlScript;
private String uid;
private String updateTime;
public Builder() {}
public Builder(GetContentResult defaults) {
Objects.requireNonNull(defaults);
this.createTime = defaults.createTime;
this.dataText = defaults.dataText;
this.description = defaults.description;
this.labels = defaults.labels;
this.name = defaults.name;
this.notebook = defaults.notebook;
this.path = defaults.path;
this.sqlScript = defaults.sqlScript;
this.uid = defaults.uid;
this.updateTime = defaults.updateTime;
}
@CustomType.Setter
public Builder createTime(String createTime) {
this.createTime = Objects.requireNonNull(createTime);
return this;
}
@CustomType.Setter
public Builder dataText(String dataText) {
this.dataText = Objects.requireNonNull(dataText);
return this;
}
@CustomType.Setter
public Builder description(String description) {
this.description = Objects.requireNonNull(description);
return this;
}
@CustomType.Setter
public Builder labels(Map labels) {
this.labels = Objects.requireNonNull(labels);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder notebook(GoogleCloudDataplexV1ContentNotebookResponse notebook) {
this.notebook = Objects.requireNonNull(notebook);
return this;
}
@CustomType.Setter
public Builder path(String path) {
this.path = Objects.requireNonNull(path);
return this;
}
@CustomType.Setter
public Builder sqlScript(GoogleCloudDataplexV1ContentSqlScriptResponse sqlScript) {
this.sqlScript = Objects.requireNonNull(sqlScript);
return this;
}
@CustomType.Setter
public Builder uid(String uid) {
this.uid = Objects.requireNonNull(uid);
return this;
}
@CustomType.Setter
public Builder updateTime(String updateTime) {
this.updateTime = Objects.requireNonNull(updateTime);
return this;
}
public GetContentResult build() {
final var o = new GetContentResult();
o.createTime = createTime;
o.dataText = dataText;
o.description = description;
o.labels = labels;
o.name = name;
o.notebook = notebook;
o.path = path;
o.sqlScript = sqlScript;
o.uid = uid;
o.updateTime = updateTime;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy