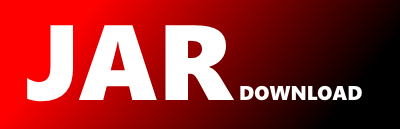
com.pulumi.googlenative.dataplex.v1.outputs.GoogleCloudDataplexV1DataQualityRuleResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.dataplex.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.dataplex.v1.outputs.GoogleCloudDataplexV1DataQualityRuleNonNullExpectationResponse;
import com.pulumi.googlenative.dataplex.v1.outputs.GoogleCloudDataplexV1DataQualityRuleRangeExpectationResponse;
import com.pulumi.googlenative.dataplex.v1.outputs.GoogleCloudDataplexV1DataQualityRuleRegexExpectationResponse;
import com.pulumi.googlenative.dataplex.v1.outputs.GoogleCloudDataplexV1DataQualityRuleRowConditionExpectationResponse;
import com.pulumi.googlenative.dataplex.v1.outputs.GoogleCloudDataplexV1DataQualityRuleSetExpectationResponse;
import com.pulumi.googlenative.dataplex.v1.outputs.GoogleCloudDataplexV1DataQualityRuleStatisticRangeExpectationResponse;
import com.pulumi.googlenative.dataplex.v1.outputs.GoogleCloudDataplexV1DataQualityRuleTableConditionExpectationResponse;
import com.pulumi.googlenative.dataplex.v1.outputs.GoogleCloudDataplexV1DataQualityRuleUniquenessExpectationResponse;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GoogleCloudDataplexV1DataQualityRuleResponse {
/**
* @return Optional. The unnested column which this rule is evaluated against.
*
*/
private String column;
/**
* @return The dimension a rule belongs to. Results are also aggregated at the dimension level. Supported dimensions are "COMPLETENESS", "ACCURACY", "CONSISTENCY", "VALIDITY", "UNIQUENESS", "INTEGRITY"
*
*/
private String dimension;
/**
* @return Optional. Rows with null values will automatically fail a rule, unless ignore_null is true. In that case, such null rows are trivially considered passing.Only applicable to ColumnMap rules.
*
*/
private Boolean ignoreNull;
/**
* @return ColumnMap rule which evaluates whether each column value is null.
*
*/
private GoogleCloudDataplexV1DataQualityRuleNonNullExpectationResponse nonNullExpectation;
/**
* @return ColumnMap rule which evaluates whether each column value lies between a specified range.
*
*/
private GoogleCloudDataplexV1DataQualityRuleRangeExpectationResponse rangeExpectation;
/**
* @return ColumnMap rule which evaluates whether each column value matches a specified regex.
*
*/
private GoogleCloudDataplexV1DataQualityRuleRegexExpectationResponse regexExpectation;
/**
* @return Table rule which evaluates whether each row passes the specified condition.
*
*/
private GoogleCloudDataplexV1DataQualityRuleRowConditionExpectationResponse rowConditionExpectation;
/**
* @return ColumnMap rule which evaluates whether each column value is contained by a specified set.
*
*/
private GoogleCloudDataplexV1DataQualityRuleSetExpectationResponse setExpectation;
/**
* @return ColumnAggregate rule which evaluates whether the column aggregate statistic lies between a specified range.
*
*/
private GoogleCloudDataplexV1DataQualityRuleStatisticRangeExpectationResponse statisticRangeExpectation;
/**
* @return Table rule which evaluates whether the provided expression is true.
*
*/
private GoogleCloudDataplexV1DataQualityRuleTableConditionExpectationResponse tableConditionExpectation;
/**
* @return Optional. The minimum ratio of passing_rows / total_rows required to pass this rule, with a range of 0.0, 1.0.0 indicates default value (i.e. 1.0).
*
*/
private Double threshold;
/**
* @return ColumnAggregate rule which evaluates whether the column has duplicates.
*
*/
private GoogleCloudDataplexV1DataQualityRuleUniquenessExpectationResponse uniquenessExpectation;
private GoogleCloudDataplexV1DataQualityRuleResponse() {}
/**
* @return Optional. The unnested column which this rule is evaluated against.
*
*/
public String column() {
return this.column;
}
/**
* @return The dimension a rule belongs to. Results are also aggregated at the dimension level. Supported dimensions are "COMPLETENESS", "ACCURACY", "CONSISTENCY", "VALIDITY", "UNIQUENESS", "INTEGRITY"
*
*/
public String dimension() {
return this.dimension;
}
/**
* @return Optional. Rows with null values will automatically fail a rule, unless ignore_null is true. In that case, such null rows are trivially considered passing.Only applicable to ColumnMap rules.
*
*/
public Boolean ignoreNull() {
return this.ignoreNull;
}
/**
* @return ColumnMap rule which evaluates whether each column value is null.
*
*/
public GoogleCloudDataplexV1DataQualityRuleNonNullExpectationResponse nonNullExpectation() {
return this.nonNullExpectation;
}
/**
* @return ColumnMap rule which evaluates whether each column value lies between a specified range.
*
*/
public GoogleCloudDataplexV1DataQualityRuleRangeExpectationResponse rangeExpectation() {
return this.rangeExpectation;
}
/**
* @return ColumnMap rule which evaluates whether each column value matches a specified regex.
*
*/
public GoogleCloudDataplexV1DataQualityRuleRegexExpectationResponse regexExpectation() {
return this.regexExpectation;
}
/**
* @return Table rule which evaluates whether each row passes the specified condition.
*
*/
public GoogleCloudDataplexV1DataQualityRuleRowConditionExpectationResponse rowConditionExpectation() {
return this.rowConditionExpectation;
}
/**
* @return ColumnMap rule which evaluates whether each column value is contained by a specified set.
*
*/
public GoogleCloudDataplexV1DataQualityRuleSetExpectationResponse setExpectation() {
return this.setExpectation;
}
/**
* @return ColumnAggregate rule which evaluates whether the column aggregate statistic lies between a specified range.
*
*/
public GoogleCloudDataplexV1DataQualityRuleStatisticRangeExpectationResponse statisticRangeExpectation() {
return this.statisticRangeExpectation;
}
/**
* @return Table rule which evaluates whether the provided expression is true.
*
*/
public GoogleCloudDataplexV1DataQualityRuleTableConditionExpectationResponse tableConditionExpectation() {
return this.tableConditionExpectation;
}
/**
* @return Optional. The minimum ratio of passing_rows / total_rows required to pass this rule, with a range of 0.0, 1.0.0 indicates default value (i.e. 1.0).
*
*/
public Double threshold() {
return this.threshold;
}
/**
* @return ColumnAggregate rule which evaluates whether the column has duplicates.
*
*/
public GoogleCloudDataplexV1DataQualityRuleUniquenessExpectationResponse uniquenessExpectation() {
return this.uniquenessExpectation;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GoogleCloudDataplexV1DataQualityRuleResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String column;
private String dimension;
private Boolean ignoreNull;
private GoogleCloudDataplexV1DataQualityRuleNonNullExpectationResponse nonNullExpectation;
private GoogleCloudDataplexV1DataQualityRuleRangeExpectationResponse rangeExpectation;
private GoogleCloudDataplexV1DataQualityRuleRegexExpectationResponse regexExpectation;
private GoogleCloudDataplexV1DataQualityRuleRowConditionExpectationResponse rowConditionExpectation;
private GoogleCloudDataplexV1DataQualityRuleSetExpectationResponse setExpectation;
private GoogleCloudDataplexV1DataQualityRuleStatisticRangeExpectationResponse statisticRangeExpectation;
private GoogleCloudDataplexV1DataQualityRuleTableConditionExpectationResponse tableConditionExpectation;
private Double threshold;
private GoogleCloudDataplexV1DataQualityRuleUniquenessExpectationResponse uniquenessExpectation;
public Builder() {}
public Builder(GoogleCloudDataplexV1DataQualityRuleResponse defaults) {
Objects.requireNonNull(defaults);
this.column = defaults.column;
this.dimension = defaults.dimension;
this.ignoreNull = defaults.ignoreNull;
this.nonNullExpectation = defaults.nonNullExpectation;
this.rangeExpectation = defaults.rangeExpectation;
this.regexExpectation = defaults.regexExpectation;
this.rowConditionExpectation = defaults.rowConditionExpectation;
this.setExpectation = defaults.setExpectation;
this.statisticRangeExpectation = defaults.statisticRangeExpectation;
this.tableConditionExpectation = defaults.tableConditionExpectation;
this.threshold = defaults.threshold;
this.uniquenessExpectation = defaults.uniquenessExpectation;
}
@CustomType.Setter
public Builder column(String column) {
this.column = Objects.requireNonNull(column);
return this;
}
@CustomType.Setter
public Builder dimension(String dimension) {
this.dimension = Objects.requireNonNull(dimension);
return this;
}
@CustomType.Setter
public Builder ignoreNull(Boolean ignoreNull) {
this.ignoreNull = Objects.requireNonNull(ignoreNull);
return this;
}
@CustomType.Setter
public Builder nonNullExpectation(GoogleCloudDataplexV1DataQualityRuleNonNullExpectationResponse nonNullExpectation) {
this.nonNullExpectation = Objects.requireNonNull(nonNullExpectation);
return this;
}
@CustomType.Setter
public Builder rangeExpectation(GoogleCloudDataplexV1DataQualityRuleRangeExpectationResponse rangeExpectation) {
this.rangeExpectation = Objects.requireNonNull(rangeExpectation);
return this;
}
@CustomType.Setter
public Builder regexExpectation(GoogleCloudDataplexV1DataQualityRuleRegexExpectationResponse regexExpectation) {
this.regexExpectation = Objects.requireNonNull(regexExpectation);
return this;
}
@CustomType.Setter
public Builder rowConditionExpectation(GoogleCloudDataplexV1DataQualityRuleRowConditionExpectationResponse rowConditionExpectation) {
this.rowConditionExpectation = Objects.requireNonNull(rowConditionExpectation);
return this;
}
@CustomType.Setter
public Builder setExpectation(GoogleCloudDataplexV1DataQualityRuleSetExpectationResponse setExpectation) {
this.setExpectation = Objects.requireNonNull(setExpectation);
return this;
}
@CustomType.Setter
public Builder statisticRangeExpectation(GoogleCloudDataplexV1DataQualityRuleStatisticRangeExpectationResponse statisticRangeExpectation) {
this.statisticRangeExpectation = Objects.requireNonNull(statisticRangeExpectation);
return this;
}
@CustomType.Setter
public Builder tableConditionExpectation(GoogleCloudDataplexV1DataQualityRuleTableConditionExpectationResponse tableConditionExpectation) {
this.tableConditionExpectation = Objects.requireNonNull(tableConditionExpectation);
return this;
}
@CustomType.Setter
public Builder threshold(Double threshold) {
this.threshold = Objects.requireNonNull(threshold);
return this;
}
@CustomType.Setter
public Builder uniquenessExpectation(GoogleCloudDataplexV1DataQualityRuleUniquenessExpectationResponse uniquenessExpectation) {
this.uniquenessExpectation = Objects.requireNonNull(uniquenessExpectation);
return this;
}
public GoogleCloudDataplexV1DataQualityRuleResponse build() {
final var o = new GoogleCloudDataplexV1DataQualityRuleResponse();
o.column = column;
o.dimension = dimension;
o.ignoreNull = ignoreNull;
o.nonNullExpectation = nonNullExpectation;
o.rangeExpectation = rangeExpectation;
o.regexExpectation = regexExpectation;
o.rowConditionExpectation = rowConditionExpectation;
o.setExpectation = setExpectation;
o.statisticRangeExpectation = statisticRangeExpectation;
o.tableConditionExpectation = tableConditionExpectation;
o.threshold = threshold;
o.uniquenessExpectation = uniquenessExpectation;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy