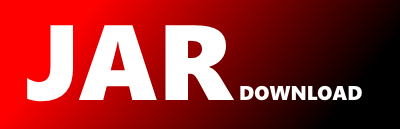
com.pulumi.googlenative.dataplex.v1.outputs.GoogleCloudDataplexV1TaskExecutionSpecResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.dataplex.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GoogleCloudDataplexV1TaskExecutionSpecResponse {
/**
* @return Optional. The arguments to pass to the task. The args can use placeholders of the format ${placeholder} as part of key/value string. These will be interpolated before passing the args to the driver. Currently supported placeholders: - ${task_id} - ${job_time} To pass positional args, set the key as TASK_ARGS. The value should be a comma-separated string of all the positional arguments. To use a delimiter other than comma, refer to https://cloud.google.com/sdk/gcloud/reference/topic/escaping. In case of other keys being present in the args, then TASK_ARGS will be passed as the last argument.
*
*/
private Map args;
/**
* @return Optional. The Cloud KMS key to use for encryption, of the form: projects/{project_number}/locations/{location_id}/keyRings/{key-ring-name}/cryptoKeys/{key-name}.
*
*/
private String kmsKey;
/**
* @return Optional. The maximum duration after which the job execution is expired.
*
*/
private String maxJobExecutionLifetime;
/**
* @return Optional. The project in which jobs are run. By default, the project containing the Lake is used. If a project is provided, the ExecutionSpec.service_account must belong to this project.
*
*/
private String project;
/**
* @return Service account to use to execute a task. If not provided, the default Compute service account for the project is used.
*
*/
private String serviceAccount;
private GoogleCloudDataplexV1TaskExecutionSpecResponse() {}
/**
* @return Optional. The arguments to pass to the task. The args can use placeholders of the format ${placeholder} as part of key/value string. These will be interpolated before passing the args to the driver. Currently supported placeholders: - ${task_id} - ${job_time} To pass positional args, set the key as TASK_ARGS. The value should be a comma-separated string of all the positional arguments. To use a delimiter other than comma, refer to https://cloud.google.com/sdk/gcloud/reference/topic/escaping. In case of other keys being present in the args, then TASK_ARGS will be passed as the last argument.
*
*/
public Map args() {
return this.args;
}
/**
* @return Optional. The Cloud KMS key to use for encryption, of the form: projects/{project_number}/locations/{location_id}/keyRings/{key-ring-name}/cryptoKeys/{key-name}.
*
*/
public String kmsKey() {
return this.kmsKey;
}
/**
* @return Optional. The maximum duration after which the job execution is expired.
*
*/
public String maxJobExecutionLifetime() {
return this.maxJobExecutionLifetime;
}
/**
* @return Optional. The project in which jobs are run. By default, the project containing the Lake is used. If a project is provided, the ExecutionSpec.service_account must belong to this project.
*
*/
public String project() {
return this.project;
}
/**
* @return Service account to use to execute a task. If not provided, the default Compute service account for the project is used.
*
*/
public String serviceAccount() {
return this.serviceAccount;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GoogleCloudDataplexV1TaskExecutionSpecResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Map args;
private String kmsKey;
private String maxJobExecutionLifetime;
private String project;
private String serviceAccount;
public Builder() {}
public Builder(GoogleCloudDataplexV1TaskExecutionSpecResponse defaults) {
Objects.requireNonNull(defaults);
this.args = defaults.args;
this.kmsKey = defaults.kmsKey;
this.maxJobExecutionLifetime = defaults.maxJobExecutionLifetime;
this.project = defaults.project;
this.serviceAccount = defaults.serviceAccount;
}
@CustomType.Setter
public Builder args(Map args) {
this.args = Objects.requireNonNull(args);
return this;
}
@CustomType.Setter
public Builder kmsKey(String kmsKey) {
this.kmsKey = Objects.requireNonNull(kmsKey);
return this;
}
@CustomType.Setter
public Builder maxJobExecutionLifetime(String maxJobExecutionLifetime) {
this.maxJobExecutionLifetime = Objects.requireNonNull(maxJobExecutionLifetime);
return this;
}
@CustomType.Setter
public Builder project(String project) {
this.project = Objects.requireNonNull(project);
return this;
}
@CustomType.Setter
public Builder serviceAccount(String serviceAccount) {
this.serviceAccount = Objects.requireNonNull(serviceAccount);
return this;
}
public GoogleCloudDataplexV1TaskExecutionSpecResponse build() {
final var o = new GoogleCloudDataplexV1TaskExecutionSpecResponse();
o.args = args;
o.kmsKey = kmsKey;
o.maxJobExecutionLifetime = maxJobExecutionLifetime;
o.project = project;
o.serviceAccount = serviceAccount;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy