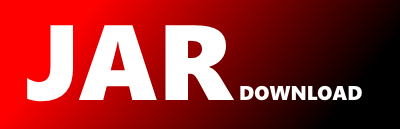
com.pulumi.googlenative.dataproc.v1.outputs.TrinoJobResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.dataproc.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.dataproc.v1.outputs.LoggingConfigResponse;
import com.pulumi.googlenative.dataproc.v1.outputs.QueryListResponse;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class TrinoJobResponse {
/**
* @return Optional. Trino client tags to attach to this query
*
*/
private List clientTags;
/**
* @return Optional. Whether to continue executing queries if a query fails. The default value is false. Setting to true can be useful when executing independent parallel queries.
*
*/
private Boolean continueOnFailure;
/**
* @return Optional. The runtime log config for job execution.
*
*/
private LoggingConfigResponse loggingConfig;
/**
* @return Optional. The format in which query output will be displayed. See the Trino documentation for supported output formats
*
*/
private String outputFormat;
/**
* @return Optional. A mapping of property names to values. Used to set Trino session properties (https://trino.io/docs/current/sql/set-session.html) Equivalent to using the --session flag in the Trino CLI
*
*/
private Map properties;
/**
* @return The HCFS URI of the script that contains SQL queries.
*
*/
private String queryFileUri;
/**
* @return A list of queries.
*
*/
private QueryListResponse queryList;
private TrinoJobResponse() {}
/**
* @return Optional. Trino client tags to attach to this query
*
*/
public List clientTags() {
return this.clientTags;
}
/**
* @return Optional. Whether to continue executing queries if a query fails. The default value is false. Setting to true can be useful when executing independent parallel queries.
*
*/
public Boolean continueOnFailure() {
return this.continueOnFailure;
}
/**
* @return Optional. The runtime log config for job execution.
*
*/
public LoggingConfigResponse loggingConfig() {
return this.loggingConfig;
}
/**
* @return Optional. The format in which query output will be displayed. See the Trino documentation for supported output formats
*
*/
public String outputFormat() {
return this.outputFormat;
}
/**
* @return Optional. A mapping of property names to values. Used to set Trino session properties (https://trino.io/docs/current/sql/set-session.html) Equivalent to using the --session flag in the Trino CLI
*
*/
public Map properties() {
return this.properties;
}
/**
* @return The HCFS URI of the script that contains SQL queries.
*
*/
public String queryFileUri() {
return this.queryFileUri;
}
/**
* @return A list of queries.
*
*/
public QueryListResponse queryList() {
return this.queryList;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(TrinoJobResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List clientTags;
private Boolean continueOnFailure;
private LoggingConfigResponse loggingConfig;
private String outputFormat;
private Map properties;
private String queryFileUri;
private QueryListResponse queryList;
public Builder() {}
public Builder(TrinoJobResponse defaults) {
Objects.requireNonNull(defaults);
this.clientTags = defaults.clientTags;
this.continueOnFailure = defaults.continueOnFailure;
this.loggingConfig = defaults.loggingConfig;
this.outputFormat = defaults.outputFormat;
this.properties = defaults.properties;
this.queryFileUri = defaults.queryFileUri;
this.queryList = defaults.queryList;
}
@CustomType.Setter
public Builder clientTags(List clientTags) {
this.clientTags = Objects.requireNonNull(clientTags);
return this;
}
public Builder clientTags(String... clientTags) {
return clientTags(List.of(clientTags));
}
@CustomType.Setter
public Builder continueOnFailure(Boolean continueOnFailure) {
this.continueOnFailure = Objects.requireNonNull(continueOnFailure);
return this;
}
@CustomType.Setter
public Builder loggingConfig(LoggingConfigResponse loggingConfig) {
this.loggingConfig = Objects.requireNonNull(loggingConfig);
return this;
}
@CustomType.Setter
public Builder outputFormat(String outputFormat) {
this.outputFormat = Objects.requireNonNull(outputFormat);
return this;
}
@CustomType.Setter
public Builder properties(Map properties) {
this.properties = Objects.requireNonNull(properties);
return this;
}
@CustomType.Setter
public Builder queryFileUri(String queryFileUri) {
this.queryFileUri = Objects.requireNonNull(queryFileUri);
return this;
}
@CustomType.Setter
public Builder queryList(QueryListResponse queryList) {
this.queryList = Objects.requireNonNull(queryList);
return this;
}
public TrinoJobResponse build() {
final var o = new TrinoJobResponse();
o.clientTags = clientTags;
o.continueOnFailure = continueOnFailure;
o.loggingConfig = loggingConfig;
o.outputFormat = outputFormat;
o.properties = properties;
o.queryFileUri = queryFileUri;
o.queryList = queryList;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy