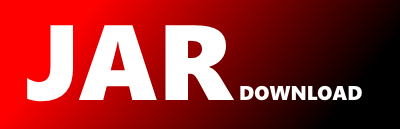
com.pulumi.googlenative.dataproc.v1beta2.Dataproc_v1beta2Functions Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.dataproc.v1beta2;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import com.pulumi.googlenative.Utilities;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetAutoscalingPolicyArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetAutoscalingPolicyIamPolicyArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetAutoscalingPolicyIamPolicyPlainArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetAutoscalingPolicyPlainArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetClusterArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetClusterPlainArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetJobArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetJobPlainArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetRegionAutoscalingPolicyIamPolicyArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetRegionAutoscalingPolicyIamPolicyPlainArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetRegionClusterIamPolicyArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetRegionClusterIamPolicyPlainArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetRegionJobIamPolicyArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetRegionJobIamPolicyPlainArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetRegionOperationIamPolicyArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetRegionOperationIamPolicyPlainArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetRegionWorkflowTemplateIamPolicyArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetRegionWorkflowTemplateIamPolicyPlainArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetWorkflowTemplateArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetWorkflowTemplateIamPolicyArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetWorkflowTemplateIamPolicyPlainArgs;
import com.pulumi.googlenative.dataproc.v1beta2.inputs.GetWorkflowTemplatePlainArgs;
import com.pulumi.googlenative.dataproc.v1beta2.outputs.GetAutoscalingPolicyIamPolicyResult;
import com.pulumi.googlenative.dataproc.v1beta2.outputs.GetAutoscalingPolicyResult;
import com.pulumi.googlenative.dataproc.v1beta2.outputs.GetClusterResult;
import com.pulumi.googlenative.dataproc.v1beta2.outputs.GetJobResult;
import com.pulumi.googlenative.dataproc.v1beta2.outputs.GetRegionAutoscalingPolicyIamPolicyResult;
import com.pulumi.googlenative.dataproc.v1beta2.outputs.GetRegionClusterIamPolicyResult;
import com.pulumi.googlenative.dataproc.v1beta2.outputs.GetRegionJobIamPolicyResult;
import com.pulumi.googlenative.dataproc.v1beta2.outputs.GetRegionOperationIamPolicyResult;
import com.pulumi.googlenative.dataproc.v1beta2.outputs.GetRegionWorkflowTemplateIamPolicyResult;
import com.pulumi.googlenative.dataproc.v1beta2.outputs.GetWorkflowTemplateIamPolicyResult;
import com.pulumi.googlenative.dataproc.v1beta2.outputs.GetWorkflowTemplateResult;
import java.util.concurrent.CompletableFuture;
public final class Dataproc_v1beta2Functions {
/**
* Retrieves autoscaling policy.
*
*/
public static Output getAutoscalingPolicy(GetAutoscalingPolicyArgs args) {
return getAutoscalingPolicy(args, InvokeOptions.Empty);
}
/**
* Retrieves autoscaling policy.
*
*/
public static CompletableFuture getAutoscalingPolicyPlain(GetAutoscalingPolicyPlainArgs args) {
return getAutoscalingPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves autoscaling policy.
*
*/
public static Output getAutoscalingPolicy(GetAutoscalingPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dataproc/v1beta2:getAutoscalingPolicy", TypeShape.of(GetAutoscalingPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves autoscaling policy.
*
*/
public static CompletableFuture getAutoscalingPolicyPlain(GetAutoscalingPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dataproc/v1beta2:getAutoscalingPolicy", TypeShape.of(GetAutoscalingPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getAutoscalingPolicyIamPolicy(GetAutoscalingPolicyIamPolicyArgs args) {
return getAutoscalingPolicyIamPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getAutoscalingPolicyIamPolicyPlain(GetAutoscalingPolicyIamPolicyPlainArgs args) {
return getAutoscalingPolicyIamPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getAutoscalingPolicyIamPolicy(GetAutoscalingPolicyIamPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dataproc/v1beta2:getAutoscalingPolicyIamPolicy", TypeShape.of(GetAutoscalingPolicyIamPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getAutoscalingPolicyIamPolicyPlain(GetAutoscalingPolicyIamPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dataproc/v1beta2:getAutoscalingPolicyIamPolicy", TypeShape.of(GetAutoscalingPolicyIamPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the resource representation for a cluster in a project.
*
*/
public static Output getCluster(GetClusterArgs args) {
return getCluster(args, InvokeOptions.Empty);
}
/**
* Gets the resource representation for a cluster in a project.
*
*/
public static CompletableFuture getClusterPlain(GetClusterPlainArgs args) {
return getClusterPlain(args, InvokeOptions.Empty);
}
/**
* Gets the resource representation for a cluster in a project.
*
*/
public static Output getCluster(GetClusterArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dataproc/v1beta2:getCluster", TypeShape.of(GetClusterResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the resource representation for a cluster in a project.
*
*/
public static CompletableFuture getClusterPlain(GetClusterPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dataproc/v1beta2:getCluster", TypeShape.of(GetClusterResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the resource representation for a job in a project.
*
*/
public static Output getJob(GetJobArgs args) {
return getJob(args, InvokeOptions.Empty);
}
/**
* Gets the resource representation for a job in a project.
*
*/
public static CompletableFuture getJobPlain(GetJobPlainArgs args) {
return getJobPlain(args, InvokeOptions.Empty);
}
/**
* Gets the resource representation for a job in a project.
*
*/
public static Output getJob(GetJobArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dataproc/v1beta2:getJob", TypeShape.of(GetJobResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the resource representation for a job in a project.
*
*/
public static CompletableFuture getJobPlain(GetJobPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dataproc/v1beta2:getJob", TypeShape.of(GetJobResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getRegionAutoscalingPolicyIamPolicy(GetRegionAutoscalingPolicyIamPolicyArgs args) {
return getRegionAutoscalingPolicyIamPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getRegionAutoscalingPolicyIamPolicyPlain(GetRegionAutoscalingPolicyIamPolicyPlainArgs args) {
return getRegionAutoscalingPolicyIamPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getRegionAutoscalingPolicyIamPolicy(GetRegionAutoscalingPolicyIamPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dataproc/v1beta2:getRegionAutoscalingPolicyIamPolicy", TypeShape.of(GetRegionAutoscalingPolicyIamPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getRegionAutoscalingPolicyIamPolicyPlain(GetRegionAutoscalingPolicyIamPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dataproc/v1beta2:getRegionAutoscalingPolicyIamPolicy", TypeShape.of(GetRegionAutoscalingPolicyIamPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getRegionClusterIamPolicy(GetRegionClusterIamPolicyArgs args) {
return getRegionClusterIamPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getRegionClusterIamPolicyPlain(GetRegionClusterIamPolicyPlainArgs args) {
return getRegionClusterIamPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getRegionClusterIamPolicy(GetRegionClusterIamPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dataproc/v1beta2:getRegionClusterIamPolicy", TypeShape.of(GetRegionClusterIamPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getRegionClusterIamPolicyPlain(GetRegionClusterIamPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dataproc/v1beta2:getRegionClusterIamPolicy", TypeShape.of(GetRegionClusterIamPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getRegionJobIamPolicy(GetRegionJobIamPolicyArgs args) {
return getRegionJobIamPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getRegionJobIamPolicyPlain(GetRegionJobIamPolicyPlainArgs args) {
return getRegionJobIamPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getRegionJobIamPolicy(GetRegionJobIamPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dataproc/v1beta2:getRegionJobIamPolicy", TypeShape.of(GetRegionJobIamPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getRegionJobIamPolicyPlain(GetRegionJobIamPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dataproc/v1beta2:getRegionJobIamPolicy", TypeShape.of(GetRegionJobIamPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getRegionOperationIamPolicy(GetRegionOperationIamPolicyArgs args) {
return getRegionOperationIamPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getRegionOperationIamPolicyPlain(GetRegionOperationIamPolicyPlainArgs args) {
return getRegionOperationIamPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getRegionOperationIamPolicy(GetRegionOperationIamPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dataproc/v1beta2:getRegionOperationIamPolicy", TypeShape.of(GetRegionOperationIamPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getRegionOperationIamPolicyPlain(GetRegionOperationIamPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dataproc/v1beta2:getRegionOperationIamPolicy", TypeShape.of(GetRegionOperationIamPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getRegionWorkflowTemplateIamPolicy(GetRegionWorkflowTemplateIamPolicyArgs args) {
return getRegionWorkflowTemplateIamPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getRegionWorkflowTemplateIamPolicyPlain(GetRegionWorkflowTemplateIamPolicyPlainArgs args) {
return getRegionWorkflowTemplateIamPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getRegionWorkflowTemplateIamPolicy(GetRegionWorkflowTemplateIamPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dataproc/v1beta2:getRegionWorkflowTemplateIamPolicy", TypeShape.of(GetRegionWorkflowTemplateIamPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getRegionWorkflowTemplateIamPolicyPlain(GetRegionWorkflowTemplateIamPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dataproc/v1beta2:getRegionWorkflowTemplateIamPolicy", TypeShape.of(GetRegionWorkflowTemplateIamPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the latest workflow template.Can retrieve previously instantiated template by specifying optional version parameter.
*
*/
public static Output getWorkflowTemplate(GetWorkflowTemplateArgs args) {
return getWorkflowTemplate(args, InvokeOptions.Empty);
}
/**
* Retrieves the latest workflow template.Can retrieve previously instantiated template by specifying optional version parameter.
*
*/
public static CompletableFuture getWorkflowTemplatePlain(GetWorkflowTemplatePlainArgs args) {
return getWorkflowTemplatePlain(args, InvokeOptions.Empty);
}
/**
* Retrieves the latest workflow template.Can retrieve previously instantiated template by specifying optional version parameter.
*
*/
public static Output getWorkflowTemplate(GetWorkflowTemplateArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dataproc/v1beta2:getWorkflowTemplate", TypeShape.of(GetWorkflowTemplateResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the latest workflow template.Can retrieve previously instantiated template by specifying optional version parameter.
*
*/
public static CompletableFuture getWorkflowTemplatePlain(GetWorkflowTemplatePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dataproc/v1beta2:getWorkflowTemplate", TypeShape.of(GetWorkflowTemplateResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getWorkflowTemplateIamPolicy(GetWorkflowTemplateIamPolicyArgs args) {
return getWorkflowTemplateIamPolicy(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getWorkflowTemplateIamPolicyPlain(GetWorkflowTemplateIamPolicyPlainArgs args) {
return getWorkflowTemplateIamPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static Output getWorkflowTemplateIamPolicy(GetWorkflowTemplateIamPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dataproc/v1beta2:getWorkflowTemplateIamPolicy", TypeShape.of(GetWorkflowTemplateIamPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and does not have a policy set.
*
*/
public static CompletableFuture getWorkflowTemplateIamPolicyPlain(GetWorkflowTemplateIamPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dataproc/v1beta2:getWorkflowTemplateIamPolicy", TypeShape.of(GetWorkflowTemplateIamPolicyResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy