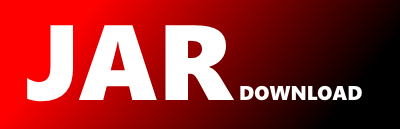
com.pulumi.googlenative.datastream.v1.outputs.PostgresqlColumnResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.datastream.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class PostgresqlColumnResponse {
/**
* @return Column name.
*
*/
private String column;
/**
* @return The PostgreSQL data type.
*
*/
private String dataType;
/**
* @return Column length.
*
*/
private Integer length;
/**
* @return Whether or not the column can accept a null value.
*
*/
private Boolean nullable;
/**
* @return The ordinal position of the column in the table.
*
*/
private Integer ordinalPosition;
/**
* @return Column precision.
*
*/
private Integer precision;
/**
* @return Whether or not the column represents a primary key.
*
*/
private Boolean primaryKey;
/**
* @return Column scale.
*
*/
private Integer scale;
private PostgresqlColumnResponse() {}
/**
* @return Column name.
*
*/
public String column() {
return this.column;
}
/**
* @return The PostgreSQL data type.
*
*/
public String dataType() {
return this.dataType;
}
/**
* @return Column length.
*
*/
public Integer length() {
return this.length;
}
/**
* @return Whether or not the column can accept a null value.
*
*/
public Boolean nullable() {
return this.nullable;
}
/**
* @return The ordinal position of the column in the table.
*
*/
public Integer ordinalPosition() {
return this.ordinalPosition;
}
/**
* @return Column precision.
*
*/
public Integer precision() {
return this.precision;
}
/**
* @return Whether or not the column represents a primary key.
*
*/
public Boolean primaryKey() {
return this.primaryKey;
}
/**
* @return Column scale.
*
*/
public Integer scale() {
return this.scale;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(PostgresqlColumnResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String column;
private String dataType;
private Integer length;
private Boolean nullable;
private Integer ordinalPosition;
private Integer precision;
private Boolean primaryKey;
private Integer scale;
public Builder() {}
public Builder(PostgresqlColumnResponse defaults) {
Objects.requireNonNull(defaults);
this.column = defaults.column;
this.dataType = defaults.dataType;
this.length = defaults.length;
this.nullable = defaults.nullable;
this.ordinalPosition = defaults.ordinalPosition;
this.precision = defaults.precision;
this.primaryKey = defaults.primaryKey;
this.scale = defaults.scale;
}
@CustomType.Setter
public Builder column(String column) {
this.column = Objects.requireNonNull(column);
return this;
}
@CustomType.Setter
public Builder dataType(String dataType) {
this.dataType = Objects.requireNonNull(dataType);
return this;
}
@CustomType.Setter
public Builder length(Integer length) {
this.length = Objects.requireNonNull(length);
return this;
}
@CustomType.Setter
public Builder nullable(Boolean nullable) {
this.nullable = Objects.requireNonNull(nullable);
return this;
}
@CustomType.Setter
public Builder ordinalPosition(Integer ordinalPosition) {
this.ordinalPosition = Objects.requireNonNull(ordinalPosition);
return this;
}
@CustomType.Setter
public Builder precision(Integer precision) {
this.precision = Objects.requireNonNull(precision);
return this;
}
@CustomType.Setter
public Builder primaryKey(Boolean primaryKey) {
this.primaryKey = Objects.requireNonNull(primaryKey);
return this;
}
@CustomType.Setter
public Builder scale(Integer scale) {
this.scale = Objects.requireNonNull(scale);
return this;
}
public PostgresqlColumnResponse build() {
final var o = new PostgresqlColumnResponse();
o.column = column;
o.dataType = dataType;
o.length = length;
o.nullable = nullable;
o.ordinalPosition = ordinalPosition;
o.precision = precision;
o.primaryKey = primaryKey;
o.scale = scale;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy