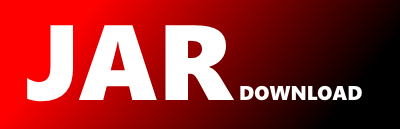
com.pulumi.googlenative.dialogflow.v2beta1.Dialogflow_v2beta1Functions Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.dialogflow.v2beta1;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import com.pulumi.googlenative.Utilities;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetContextArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetContextPlainArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetConversationArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetConversationPlainArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetConversationProfileArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetConversationProfilePlainArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetDocumentArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetDocumentPlainArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetEntityTypeArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetEntityTypePlainArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetEnvironmentArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetEnvironmentPlainArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetIntentArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetIntentPlainArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetKnowledgeBaseArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetKnowledgeBasePlainArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetParticipantArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetParticipantPlainArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetSessionEntityTypeArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetSessionEntityTypePlainArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetVersionArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.inputs.GetVersionPlainArgs;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GetContextResult;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GetConversationProfileResult;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GetConversationResult;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GetDocumentResult;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GetEntityTypeResult;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GetEnvironmentResult;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GetIntentResult;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GetKnowledgeBaseResult;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GetParticipantResult;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GetSessionEntityTypeResult;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GetVersionResult;
import java.util.concurrent.CompletableFuture;
public final class Dialogflow_v2beta1Functions {
/**
* Retrieves the specified context.
*
*/
public static Output getContext(GetContextArgs args) {
return getContext(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified context.
*
*/
public static CompletableFuture getContextPlain(GetContextPlainArgs args) {
return getContextPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified context.
*
*/
public static Output getContext(GetContextArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dialogflow/v2beta1:getContext", TypeShape.of(GetContextResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified context.
*
*/
public static CompletableFuture getContextPlain(GetContextPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dialogflow/v2beta1:getContext", TypeShape.of(GetContextResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specific conversation.
*
*/
public static Output getConversation(GetConversationArgs args) {
return getConversation(args, InvokeOptions.Empty);
}
/**
* Retrieves the specific conversation.
*
*/
public static CompletableFuture getConversationPlain(GetConversationPlainArgs args) {
return getConversationPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves the specific conversation.
*
*/
public static Output getConversation(GetConversationArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dialogflow/v2beta1:getConversation", TypeShape.of(GetConversationResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specific conversation.
*
*/
public static CompletableFuture getConversationPlain(GetConversationPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dialogflow/v2beta1:getConversation", TypeShape.of(GetConversationResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified conversation profile.
*
*/
public static Output getConversationProfile(GetConversationProfileArgs args) {
return getConversationProfile(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified conversation profile.
*
*/
public static CompletableFuture getConversationProfilePlain(GetConversationProfilePlainArgs args) {
return getConversationProfilePlain(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified conversation profile.
*
*/
public static Output getConversationProfile(GetConversationProfileArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dialogflow/v2beta1:getConversationProfile", TypeShape.of(GetConversationProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified conversation profile.
*
*/
public static CompletableFuture getConversationProfilePlain(GetConversationProfilePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dialogflow/v2beta1:getConversationProfile", TypeShape.of(GetConversationProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified document. Note: The `projects.agent.knowledgeBases.documents` resource is deprecated; only use `projects.knowledgeBases.documents`.
*
*/
public static Output getDocument(GetDocumentArgs args) {
return getDocument(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified document. Note: The `projects.agent.knowledgeBases.documents` resource is deprecated; only use `projects.knowledgeBases.documents`.
*
*/
public static CompletableFuture getDocumentPlain(GetDocumentPlainArgs args) {
return getDocumentPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified document. Note: The `projects.agent.knowledgeBases.documents` resource is deprecated; only use `projects.knowledgeBases.documents`.
*
*/
public static Output getDocument(GetDocumentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dialogflow/v2beta1:getDocument", TypeShape.of(GetDocumentResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified document. Note: The `projects.agent.knowledgeBases.documents` resource is deprecated; only use `projects.knowledgeBases.documents`.
*
*/
public static CompletableFuture getDocumentPlain(GetDocumentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dialogflow/v2beta1:getDocument", TypeShape.of(GetDocumentResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified entity type.
*
*/
public static Output getEntityType(GetEntityTypeArgs args) {
return getEntityType(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified entity type.
*
*/
public static CompletableFuture getEntityTypePlain(GetEntityTypePlainArgs args) {
return getEntityTypePlain(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified entity type.
*
*/
public static Output getEntityType(GetEntityTypeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dialogflow/v2beta1:getEntityType", TypeShape.of(GetEntityTypeResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified entity type.
*
*/
public static CompletableFuture getEntityTypePlain(GetEntityTypePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dialogflow/v2beta1:getEntityType", TypeShape.of(GetEntityTypeResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified agent environment.
*
*/
public static Output getEnvironment(GetEnvironmentArgs args) {
return getEnvironment(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified agent environment.
*
*/
public static CompletableFuture getEnvironmentPlain(GetEnvironmentPlainArgs args) {
return getEnvironmentPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified agent environment.
*
*/
public static Output getEnvironment(GetEnvironmentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dialogflow/v2beta1:getEnvironment", TypeShape.of(GetEnvironmentResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified agent environment.
*
*/
public static CompletableFuture getEnvironmentPlain(GetEnvironmentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dialogflow/v2beta1:getEnvironment", TypeShape.of(GetEnvironmentResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified intent.
*
*/
public static Output getIntent(GetIntentArgs args) {
return getIntent(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified intent.
*
*/
public static CompletableFuture getIntentPlain(GetIntentPlainArgs args) {
return getIntentPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified intent.
*
*/
public static Output getIntent(GetIntentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dialogflow/v2beta1:getIntent", TypeShape.of(GetIntentResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified intent.
*
*/
public static CompletableFuture getIntentPlain(GetIntentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dialogflow/v2beta1:getIntent", TypeShape.of(GetIntentResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified knowledge base. Note: The `projects.agent.knowledgeBases` resource is deprecated; only use `projects.knowledgeBases`.
*
*/
public static Output getKnowledgeBase(GetKnowledgeBaseArgs args) {
return getKnowledgeBase(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified knowledge base. Note: The `projects.agent.knowledgeBases` resource is deprecated; only use `projects.knowledgeBases`.
*
*/
public static CompletableFuture getKnowledgeBasePlain(GetKnowledgeBasePlainArgs args) {
return getKnowledgeBasePlain(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified knowledge base. Note: The `projects.agent.knowledgeBases` resource is deprecated; only use `projects.knowledgeBases`.
*
*/
public static Output getKnowledgeBase(GetKnowledgeBaseArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dialogflow/v2beta1:getKnowledgeBase", TypeShape.of(GetKnowledgeBaseResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified knowledge base. Note: The `projects.agent.knowledgeBases` resource is deprecated; only use `projects.knowledgeBases`.
*
*/
public static CompletableFuture getKnowledgeBasePlain(GetKnowledgeBasePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dialogflow/v2beta1:getKnowledgeBase", TypeShape.of(GetKnowledgeBaseResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves a conversation participant.
*
*/
public static Output getParticipant(GetParticipantArgs args) {
return getParticipant(args, InvokeOptions.Empty);
}
/**
* Retrieves a conversation participant.
*
*/
public static CompletableFuture getParticipantPlain(GetParticipantPlainArgs args) {
return getParticipantPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves a conversation participant.
*
*/
public static Output getParticipant(GetParticipantArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dialogflow/v2beta1:getParticipant", TypeShape.of(GetParticipantResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves a conversation participant.
*
*/
public static CompletableFuture getParticipantPlain(GetParticipantPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dialogflow/v2beta1:getParticipant", TypeShape.of(GetParticipantResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified session entity type. This method doesn't work with Google Assistant integration. Contact Dialogflow support if you need to use session entities with Google Assistant integration.
*
*/
public static Output getSessionEntityType(GetSessionEntityTypeArgs args) {
return getSessionEntityType(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified session entity type. This method doesn't work with Google Assistant integration. Contact Dialogflow support if you need to use session entities with Google Assistant integration.
*
*/
public static CompletableFuture getSessionEntityTypePlain(GetSessionEntityTypePlainArgs args) {
return getSessionEntityTypePlain(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified session entity type. This method doesn't work with Google Assistant integration. Contact Dialogflow support if you need to use session entities with Google Assistant integration.
*
*/
public static Output getSessionEntityType(GetSessionEntityTypeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dialogflow/v2beta1:getSessionEntityType", TypeShape.of(GetSessionEntityTypeResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified session entity type. This method doesn't work with Google Assistant integration. Contact Dialogflow support if you need to use session entities with Google Assistant integration.
*
*/
public static CompletableFuture getSessionEntityTypePlain(GetSessionEntityTypePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dialogflow/v2beta1:getSessionEntityType", TypeShape.of(GetSessionEntityTypeResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified agent version.
*
*/
public static Output getVersion(GetVersionArgs args) {
return getVersion(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified agent version.
*
*/
public static CompletableFuture getVersionPlain(GetVersionPlainArgs args) {
return getVersionPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves the specified agent version.
*
*/
public static Output getVersion(GetVersionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:dialogflow/v2beta1:getVersion", TypeShape.of(GetVersionResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves the specified agent version.
*
*/
public static CompletableFuture getVersionPlain(GetVersionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:dialogflow/v2beta1:getVersion", TypeShape.of(GetVersionResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy