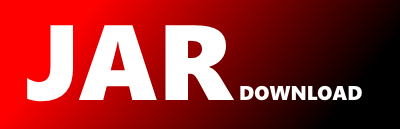
com.pulumi.googlenative.dialogflow.v2beta1.outputs.GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.dialogflow.v2beta1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigContextFilterSettingsResponse;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigDialogflowQuerySourceResponse;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigDocumentQuerySourceResponse;
import com.pulumi.googlenative.dialogflow.v2beta1.outputs.GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigKnowledgeBaseQuerySourceResponse;
import java.lang.Double;
import java.lang.Integer;
import java.util.Objects;
@CustomType
public final class GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigResponse {
/**
* @return Confidence threshold of query result. Agent Assist gives each suggestion a score in the range [0.0, 1.0], based on the relevance between the suggestion and the current conversation context. A score of 0.0 has no relevance, while a score of 1.0 has high relevance. Only suggestions with a score greater than or equal to the value of this field are included in the results. For a baseline model (the default), the recommended value is in the range [0.05, 0.1]. For a custom model, there is no recommended value. Tune this value by starting from a very low value and slowly increasing until you have desired results. If this field is not set, it is default to 0.0, which means that all suggestions are returned. Supported features: ARTICLE_SUGGESTION, FAQ, SMART_REPLY, SMART_COMPOSE, KNOWLEDGE_SEARCH, KNOWLEDGE_ASSIST.
*
*/
private Double confidenceThreshold;
/**
* @return Determines how recent conversation context is filtered when generating suggestions. If unspecified, no messages will be dropped.
*
*/
private GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigContextFilterSettingsResponse contextFilterSettings;
/**
* @return Query from Dialogflow agent. It is used by DIALOGFLOW_ASSIST.
*
*/
private GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigDialogflowQuerySourceResponse dialogflowQuerySource;
/**
* @return Query from knowledge base document. It is used by: SMART_REPLY, SMART_COMPOSE.
*
*/
private GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigDocumentQuerySourceResponse documentQuerySource;
/**
* @return Query from knowledgebase. It is used by: ARTICLE_SUGGESTION, FAQ.
*
*/
private GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigKnowledgeBaseQuerySourceResponse knowledgeBaseQuerySource;
/**
* @return Maximum number of results to return. Currently, if unset, defaults to 10. And the max number is 20.
*
*/
private Integer maxResults;
private GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigResponse() {}
/**
* @return Confidence threshold of query result. Agent Assist gives each suggestion a score in the range [0.0, 1.0], based on the relevance between the suggestion and the current conversation context. A score of 0.0 has no relevance, while a score of 1.0 has high relevance. Only suggestions with a score greater than or equal to the value of this field are included in the results. For a baseline model (the default), the recommended value is in the range [0.05, 0.1]. For a custom model, there is no recommended value. Tune this value by starting from a very low value and slowly increasing until you have desired results. If this field is not set, it is default to 0.0, which means that all suggestions are returned. Supported features: ARTICLE_SUGGESTION, FAQ, SMART_REPLY, SMART_COMPOSE, KNOWLEDGE_SEARCH, KNOWLEDGE_ASSIST.
*
*/
public Double confidenceThreshold() {
return this.confidenceThreshold;
}
/**
* @return Determines how recent conversation context is filtered when generating suggestions. If unspecified, no messages will be dropped.
*
*/
public GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigContextFilterSettingsResponse contextFilterSettings() {
return this.contextFilterSettings;
}
/**
* @return Query from Dialogflow agent. It is used by DIALOGFLOW_ASSIST.
*
*/
public GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigDialogflowQuerySourceResponse dialogflowQuerySource() {
return this.dialogflowQuerySource;
}
/**
* @return Query from knowledge base document. It is used by: SMART_REPLY, SMART_COMPOSE.
*
*/
public GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigDocumentQuerySourceResponse documentQuerySource() {
return this.documentQuerySource;
}
/**
* @return Query from knowledgebase. It is used by: ARTICLE_SUGGESTION, FAQ.
*
*/
public GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigKnowledgeBaseQuerySourceResponse knowledgeBaseQuerySource() {
return this.knowledgeBaseQuerySource;
}
/**
* @return Maximum number of results to return. Currently, if unset, defaults to 10. And the max number is 20.
*
*/
public Integer maxResults() {
return this.maxResults;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Double confidenceThreshold;
private GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigContextFilterSettingsResponse contextFilterSettings;
private GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigDialogflowQuerySourceResponse dialogflowQuerySource;
private GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigDocumentQuerySourceResponse documentQuerySource;
private GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigKnowledgeBaseQuerySourceResponse knowledgeBaseQuerySource;
private Integer maxResults;
public Builder() {}
public Builder(GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigResponse defaults) {
Objects.requireNonNull(defaults);
this.confidenceThreshold = defaults.confidenceThreshold;
this.contextFilterSettings = defaults.contextFilterSettings;
this.dialogflowQuerySource = defaults.dialogflowQuerySource;
this.documentQuerySource = defaults.documentQuerySource;
this.knowledgeBaseQuerySource = defaults.knowledgeBaseQuerySource;
this.maxResults = defaults.maxResults;
}
@CustomType.Setter
public Builder confidenceThreshold(Double confidenceThreshold) {
this.confidenceThreshold = Objects.requireNonNull(confidenceThreshold);
return this;
}
@CustomType.Setter
public Builder contextFilterSettings(GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigContextFilterSettingsResponse contextFilterSettings) {
this.contextFilterSettings = Objects.requireNonNull(contextFilterSettings);
return this;
}
@CustomType.Setter
public Builder dialogflowQuerySource(GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigDialogflowQuerySourceResponse dialogflowQuerySource) {
this.dialogflowQuerySource = Objects.requireNonNull(dialogflowQuerySource);
return this;
}
@CustomType.Setter
public Builder documentQuerySource(GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigDocumentQuerySourceResponse documentQuerySource) {
this.documentQuerySource = Objects.requireNonNull(documentQuerySource);
return this;
}
@CustomType.Setter
public Builder knowledgeBaseQuerySource(GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigKnowledgeBaseQuerySourceResponse knowledgeBaseQuerySource) {
this.knowledgeBaseQuerySource = Objects.requireNonNull(knowledgeBaseQuerySource);
return this;
}
@CustomType.Setter
public Builder maxResults(Integer maxResults) {
this.maxResults = Objects.requireNonNull(maxResults);
return this;
}
public GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigResponse build() {
final var o = new GoogleCloudDialogflowV2beta1HumanAgentAssistantConfigSuggestionQueryConfigResponse();
o.confidenceThreshold = confidenceThreshold;
o.contextFilterSettings = contextFilterSettings;
o.dialogflowQuerySource = dialogflowQuerySource;
o.documentQuerySource = documentQuerySource;
o.knowledgeBaseQuerySource = knowledgeBaseQuerySource;
o.maxResults = maxResults;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy