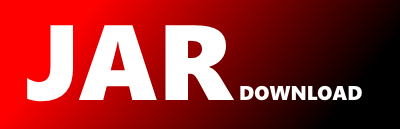
com.pulumi.googlenative.dialogflow.v3beta1.AgentArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.dialogflow.v3beta1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.dialogflow.v3beta1.inputs.GoogleCloudDialogflowCxV3beta1AdvancedSettingsArgs;
import com.pulumi.googlenative.dialogflow.v3beta1.inputs.GoogleCloudDialogflowCxV3beta1SpeechToTextSettingsArgs;
import com.pulumi.googlenative.dialogflow.v3beta1.inputs.GoogleCloudDialogflowCxV3beta1TextToSpeechSettingsArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AgentArgs extends com.pulumi.resources.ResourceArgs {
public static final AgentArgs Empty = new AgentArgs();
/**
* Hierarchical advanced settings for this agent. The settings exposed at the lower level overrides the settings exposed at the higher level.
*
*/
@Import(name="advancedSettings")
private @Nullable Output advancedSettings;
/**
* @return Hierarchical advanced settings for this agent. The settings exposed at the lower level overrides the settings exposed at the higher level.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy