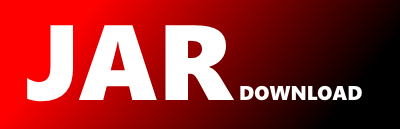
com.pulumi.googlenative.dialogflow.v3beta1.TestCaseArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.dialogflow.v3beta1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.dialogflow.v3beta1.inputs.GoogleCloudDialogflowCxV3beta1ConversationTurnArgs;
import com.pulumi.googlenative.dialogflow.v3beta1.inputs.GoogleCloudDialogflowCxV3beta1TestCaseResultArgs;
import com.pulumi.googlenative.dialogflow.v3beta1.inputs.GoogleCloudDialogflowCxV3beta1TestConfigArgs;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TestCaseArgs extends com.pulumi.resources.ResourceArgs {
public static final TestCaseArgs Empty = new TestCaseArgs();
@Import(name="agentId", required=true)
private Output agentId;
public Output agentId() {
return this.agentId;
}
/**
* The human-readable name of the test case, unique within the agent. Limit of 200 characters.
*
*/
@Import(name="displayName", required=true)
private Output displayName;
/**
* @return The human-readable name of the test case, unique within the agent. Limit of 200 characters.
*
*/
public Output displayName() {
return this.displayName;
}
/**
* The latest test result.
*
*/
@Import(name="lastTestResult")
private @Nullable Output lastTestResult;
/**
* @return The latest test result.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy