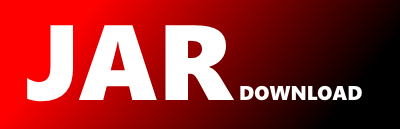
com.pulumi.googlenative.dialogflow.v3beta1.inputs.GoogleCloudDialogflowCxV3beta1WebhookGenericWebServiceArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.dialogflow.v3beta1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.dialogflow.v3beta1.enums.GoogleCloudDialogflowCxV3beta1WebhookGenericWebServiceHttpMethod;
import com.pulumi.googlenative.dialogflow.v3beta1.enums.GoogleCloudDialogflowCxV3beta1WebhookGenericWebServiceWebhookType;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Represents configuration for a generic web service.
*
*/
public final class GoogleCloudDialogflowCxV3beta1WebhookGenericWebServiceArgs extends com.pulumi.resources.ResourceArgs {
public static final GoogleCloudDialogflowCxV3beta1WebhookGenericWebServiceArgs Empty = new GoogleCloudDialogflowCxV3beta1WebhookGenericWebServiceArgs();
/**
* Optional. Specifies a list of allowed custom CA certificates (in DER format) for HTTPS verification. This overrides the default SSL trust store. If this is empty or unspecified, Dialogflow will use Google's default trust store to verify certificates. N.B. Make sure the HTTPS server certificates are signed with "subject alt name". For instance a certificate can be self-signed using the following command, ```openssl x509 -req -days 200 -in example.com.csr \ -signkey example.com.key \ -out example.com.crt \ -extfile <(printf "\nsubjectAltName='DNS:www.example.com'")```
*
*/
@Import(name="allowedCaCerts")
private @Nullable Output> allowedCaCerts;
/**
* @return Optional. Specifies a list of allowed custom CA certificates (in DER format) for HTTPS verification. This overrides the default SSL trust store. If this is empty or unspecified, Dialogflow will use Google's default trust store to verify certificates. N.B. Make sure the HTTPS server certificates are signed with "subject alt name". For instance a certificate can be self-signed using the following command, ```openssl x509 -req -days 200 -in example.com.csr \ -signkey example.com.key \ -out example.com.crt \ -extfile <(printf "\nsubjectAltName='DNS:www.example.com'")```
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy