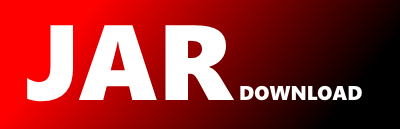
com.pulumi.googlenative.dlp.v2.inputs.GooglePrivacyDlpV2PrimitiveTransformationArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.dlp.v2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.dlp.v2.inputs.GooglePrivacyDlpV2BucketingConfigArgs;
import com.pulumi.googlenative.dlp.v2.inputs.GooglePrivacyDlpV2CharacterMaskConfigArgs;
import com.pulumi.googlenative.dlp.v2.inputs.GooglePrivacyDlpV2CryptoDeterministicConfigArgs;
import com.pulumi.googlenative.dlp.v2.inputs.GooglePrivacyDlpV2CryptoHashConfigArgs;
import com.pulumi.googlenative.dlp.v2.inputs.GooglePrivacyDlpV2CryptoReplaceFfxFpeConfigArgs;
import com.pulumi.googlenative.dlp.v2.inputs.GooglePrivacyDlpV2DateShiftConfigArgs;
import com.pulumi.googlenative.dlp.v2.inputs.GooglePrivacyDlpV2FixedSizeBucketingConfigArgs;
import com.pulumi.googlenative.dlp.v2.inputs.GooglePrivacyDlpV2RedactConfigArgs;
import com.pulumi.googlenative.dlp.v2.inputs.GooglePrivacyDlpV2ReplaceDictionaryConfigArgs;
import com.pulumi.googlenative.dlp.v2.inputs.GooglePrivacyDlpV2ReplaceValueConfigArgs;
import com.pulumi.googlenative.dlp.v2.inputs.GooglePrivacyDlpV2ReplaceWithInfoTypeConfigArgs;
import com.pulumi.googlenative.dlp.v2.inputs.GooglePrivacyDlpV2TimePartConfigArgs;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A rule for transforming a value.
*
*/
public final class GooglePrivacyDlpV2PrimitiveTransformationArgs extends com.pulumi.resources.ResourceArgs {
public static final GooglePrivacyDlpV2PrimitiveTransformationArgs Empty = new GooglePrivacyDlpV2PrimitiveTransformationArgs();
/**
* Bucketing
*
*/
@Import(name="bucketingConfig")
private @Nullable Output bucketingConfig;
/**
* @return Bucketing
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy