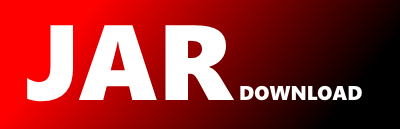
com.pulumi.googlenative.firebasehosting.v1beta1.Firebasehosting_v1beta1Functions Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.firebasehosting.v1beta1;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import com.pulumi.googlenative.Utilities;
import com.pulumi.googlenative.firebasehosting.v1beta1.inputs.GetChannelArgs;
import com.pulumi.googlenative.firebasehosting.v1beta1.inputs.GetChannelPlainArgs;
import com.pulumi.googlenative.firebasehosting.v1beta1.inputs.GetDomainArgs;
import com.pulumi.googlenative.firebasehosting.v1beta1.inputs.GetDomainPlainArgs;
import com.pulumi.googlenative.firebasehosting.v1beta1.inputs.GetReleaseArgs;
import com.pulumi.googlenative.firebasehosting.v1beta1.inputs.GetReleasePlainArgs;
import com.pulumi.googlenative.firebasehosting.v1beta1.inputs.GetSiteArgs;
import com.pulumi.googlenative.firebasehosting.v1beta1.inputs.GetSitePlainArgs;
import com.pulumi.googlenative.firebasehosting.v1beta1.inputs.GetVersionArgs;
import com.pulumi.googlenative.firebasehosting.v1beta1.inputs.GetVersionPlainArgs;
import com.pulumi.googlenative.firebasehosting.v1beta1.outputs.GetChannelResult;
import com.pulumi.googlenative.firebasehosting.v1beta1.outputs.GetDomainResult;
import com.pulumi.googlenative.firebasehosting.v1beta1.outputs.GetReleaseResult;
import com.pulumi.googlenative.firebasehosting.v1beta1.outputs.GetSiteResult;
import com.pulumi.googlenative.firebasehosting.v1beta1.outputs.GetVersionResult;
import java.util.concurrent.CompletableFuture;
public final class Firebasehosting_v1beta1Functions {
/**
* Retrieves information for the specified channel of the specified site.
*
*/
public static Output getChannel(GetChannelArgs args) {
return getChannel(args, InvokeOptions.Empty);
}
/**
* Retrieves information for the specified channel of the specified site.
*
*/
public static CompletableFuture getChannelPlain(GetChannelPlainArgs args) {
return getChannelPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves information for the specified channel of the specified site.
*
*/
public static Output getChannel(GetChannelArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:firebasehosting/v1beta1:getChannel", TypeShape.of(GetChannelResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves information for the specified channel of the specified site.
*
*/
public static CompletableFuture getChannelPlain(GetChannelPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:firebasehosting/v1beta1:getChannel", TypeShape.of(GetChannelResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a domain mapping on the specified site.
*
*/
public static Output getDomain(GetDomainArgs args) {
return getDomain(args, InvokeOptions.Empty);
}
/**
* Gets a domain mapping on the specified site.
*
*/
public static CompletableFuture getDomainPlain(GetDomainPlainArgs args) {
return getDomainPlain(args, InvokeOptions.Empty);
}
/**
* Gets a domain mapping on the specified site.
*
*/
public static Output getDomain(GetDomainArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:firebasehosting/v1beta1:getDomain", TypeShape.of(GetDomainResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a domain mapping on the specified site.
*
*/
public static CompletableFuture getDomainPlain(GetDomainPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:firebasehosting/v1beta1:getDomain", TypeShape.of(GetDomainResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified release for a site or channel. When used to get a release for a site, this can get releases for both the default `live` channel and any active preview channels for the specified site.
*
*/
public static Output getRelease(GetReleaseArgs args) {
return getRelease(args, InvokeOptions.Empty);
}
/**
* Gets the specified release for a site or channel. When used to get a release for a site, this can get releases for both the default `live` channel and any active preview channels for the specified site.
*
*/
public static CompletableFuture getReleasePlain(GetReleasePlainArgs args) {
return getReleasePlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified release for a site or channel. When used to get a release for a site, this can get releases for both the default `live` channel and any active preview channels for the specified site.
*
*/
public static Output getRelease(GetReleaseArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:firebasehosting/v1beta1:getRelease", TypeShape.of(GetReleaseResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified release for a site or channel. When used to get a release for a site, this can get releases for both the default `live` channel and any active preview channels for the specified site.
*
*/
public static CompletableFuture getReleasePlain(GetReleasePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:firebasehosting/v1beta1:getRelease", TypeShape.of(GetReleaseResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified Hosting Site.
*
*/
public static Output getSite(GetSiteArgs args) {
return getSite(args, InvokeOptions.Empty);
}
/**
* Gets the specified Hosting Site.
*
*/
public static CompletableFuture getSitePlain(GetSitePlainArgs args) {
return getSitePlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified Hosting Site.
*
*/
public static Output getSite(GetSiteArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:firebasehosting/v1beta1:getSite", TypeShape.of(GetSiteResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified Hosting Site.
*
*/
public static CompletableFuture getSitePlain(GetSitePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:firebasehosting/v1beta1:getSite", TypeShape.of(GetSiteResult.class), args, Utilities.withVersion(options));
}
/**
* Get the specified version that has been created for the specified site. This can include versions that were created for the default `live` channel or for any active preview channels for the specified site.
*
*/
public static Output getVersion(GetVersionArgs args) {
return getVersion(args, InvokeOptions.Empty);
}
/**
* Get the specified version that has been created for the specified site. This can include versions that were created for the default `live` channel or for any active preview channels for the specified site.
*
*/
public static CompletableFuture getVersionPlain(GetVersionPlainArgs args) {
return getVersionPlain(args, InvokeOptions.Empty);
}
/**
* Get the specified version that has been created for the specified site. This can include versions that were created for the default `live` channel or for any active preview channels for the specified site.
*
*/
public static Output getVersion(GetVersionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("google-native:firebasehosting/v1beta1:getVersion", TypeShape.of(GetVersionResult.class), args, Utilities.withVersion(options));
}
/**
* Get the specified version that has been created for the specified site. This can include versions that were created for the default `live` channel or for any active preview channels for the specified site.
*
*/
public static CompletableFuture getVersionPlain(GetVersionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("google-native:firebasehosting/v1beta1:getVersion", TypeShape.of(GetVersionResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy