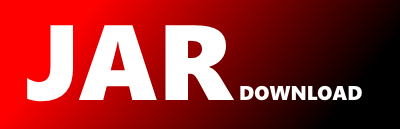
com.pulumi.googlenative.firebasehosting.v1beta1.outputs.DomainProvisioningResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.firebasehosting.v1beta1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.firebasehosting.v1beta1.outputs.CertDnsChallengeResponse;
import com.pulumi.googlenative.firebasehosting.v1beta1.outputs.CertHttpChallengeResponse;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class DomainProvisioningResponse {
/**
* @return The TXT records (for the certificate challenge) that were found at the last DNS fetch.
*
*/
private List certChallengeDiscoveredTxt;
/**
* @return The DNS challenge for generating a certificate.
*
*/
private CertDnsChallengeResponse certChallengeDns;
/**
* @return The HTTP challenge for generating a certificate.
*
*/
private CertHttpChallengeResponse certChallengeHttp;
/**
* @return The certificate provisioning status; updated when Firebase Hosting provisions an SSL certificate for the domain.
*
*/
private String certStatus;
/**
* @return The IPs found at the last DNS fetch.
*
*/
private List discoveredIps;
/**
* @return The time at which the last DNS fetch occurred.
*
*/
private String dnsFetchTime;
/**
* @return The DNS record match status as of the last DNS fetch.
*
*/
private String dnsStatus;
/**
* @return The list of IPs to which the domain is expected to resolve.
*
*/
private List expectedIps;
private DomainProvisioningResponse() {}
/**
* @return The TXT records (for the certificate challenge) that were found at the last DNS fetch.
*
*/
public List certChallengeDiscoveredTxt() {
return this.certChallengeDiscoveredTxt;
}
/**
* @return The DNS challenge for generating a certificate.
*
*/
public CertDnsChallengeResponse certChallengeDns() {
return this.certChallengeDns;
}
/**
* @return The HTTP challenge for generating a certificate.
*
*/
public CertHttpChallengeResponse certChallengeHttp() {
return this.certChallengeHttp;
}
/**
* @return The certificate provisioning status; updated when Firebase Hosting provisions an SSL certificate for the domain.
*
*/
public String certStatus() {
return this.certStatus;
}
/**
* @return The IPs found at the last DNS fetch.
*
*/
public List discoveredIps() {
return this.discoveredIps;
}
/**
* @return The time at which the last DNS fetch occurred.
*
*/
public String dnsFetchTime() {
return this.dnsFetchTime;
}
/**
* @return The DNS record match status as of the last DNS fetch.
*
*/
public String dnsStatus() {
return this.dnsStatus;
}
/**
* @return The list of IPs to which the domain is expected to resolve.
*
*/
public List expectedIps() {
return this.expectedIps;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DomainProvisioningResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List certChallengeDiscoveredTxt;
private CertDnsChallengeResponse certChallengeDns;
private CertHttpChallengeResponse certChallengeHttp;
private String certStatus;
private List discoveredIps;
private String dnsFetchTime;
private String dnsStatus;
private List expectedIps;
public Builder() {}
public Builder(DomainProvisioningResponse defaults) {
Objects.requireNonNull(defaults);
this.certChallengeDiscoveredTxt = defaults.certChallengeDiscoveredTxt;
this.certChallengeDns = defaults.certChallengeDns;
this.certChallengeHttp = defaults.certChallengeHttp;
this.certStatus = defaults.certStatus;
this.discoveredIps = defaults.discoveredIps;
this.dnsFetchTime = defaults.dnsFetchTime;
this.dnsStatus = defaults.dnsStatus;
this.expectedIps = defaults.expectedIps;
}
@CustomType.Setter
public Builder certChallengeDiscoveredTxt(List certChallengeDiscoveredTxt) {
this.certChallengeDiscoveredTxt = Objects.requireNonNull(certChallengeDiscoveredTxt);
return this;
}
public Builder certChallengeDiscoveredTxt(String... certChallengeDiscoveredTxt) {
return certChallengeDiscoveredTxt(List.of(certChallengeDiscoveredTxt));
}
@CustomType.Setter
public Builder certChallengeDns(CertDnsChallengeResponse certChallengeDns) {
this.certChallengeDns = Objects.requireNonNull(certChallengeDns);
return this;
}
@CustomType.Setter
public Builder certChallengeHttp(CertHttpChallengeResponse certChallengeHttp) {
this.certChallengeHttp = Objects.requireNonNull(certChallengeHttp);
return this;
}
@CustomType.Setter
public Builder certStatus(String certStatus) {
this.certStatus = Objects.requireNonNull(certStatus);
return this;
}
@CustomType.Setter
public Builder discoveredIps(List discoveredIps) {
this.discoveredIps = Objects.requireNonNull(discoveredIps);
return this;
}
public Builder discoveredIps(String... discoveredIps) {
return discoveredIps(List.of(discoveredIps));
}
@CustomType.Setter
public Builder dnsFetchTime(String dnsFetchTime) {
this.dnsFetchTime = Objects.requireNonNull(dnsFetchTime);
return this;
}
@CustomType.Setter
public Builder dnsStatus(String dnsStatus) {
this.dnsStatus = Objects.requireNonNull(dnsStatus);
return this;
}
@CustomType.Setter
public Builder expectedIps(List expectedIps) {
this.expectedIps = Objects.requireNonNull(expectedIps);
return this;
}
public Builder expectedIps(String... expectedIps) {
return expectedIps(List.of(expectedIps));
}
public DomainProvisioningResponse build() {
final var o = new DomainProvisioningResponse();
o.certChallengeDiscoveredTxt = certChallengeDiscoveredTxt;
o.certChallengeDns = certChallengeDns;
o.certChallengeHttp = certChallengeHttp;
o.certStatus = certStatus;
o.discoveredIps = discoveredIps;
o.dnsFetchTime = dnsFetchTime;
o.dnsStatus = dnsStatus;
o.expectedIps = expectedIps;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy