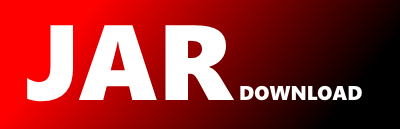
com.pulumi.googlenative.firestore.v1.DatabaseArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.firestore.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.firestore.v1.enums.DatabaseAppEngineIntegrationMode;
import com.pulumi.googlenative.firestore.v1.enums.DatabaseConcurrencyMode;
import com.pulumi.googlenative.firestore.v1.enums.DatabaseDeleteProtectionState;
import com.pulumi.googlenative.firestore.v1.enums.DatabaseType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DatabaseArgs extends com.pulumi.resources.ResourceArgs {
public static final DatabaseArgs Empty = new DatabaseArgs();
/**
* The App Engine integration mode to use for this database.
*
*/
@Import(name="appEngineIntegrationMode")
private @Nullable Output appEngineIntegrationMode;
/**
* @return The App Engine integration mode to use for this database.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy