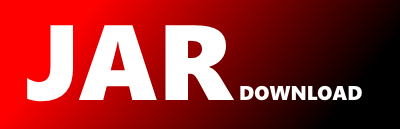
com.pulumi.googlenative.genomics.v1alpha2.PipelineArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.genomics.v1alpha2;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.genomics.v1alpha2.inputs.DockerExecutorArgs;
import com.pulumi.googlenative.genomics.v1alpha2.inputs.PipelineParameterArgs;
import com.pulumi.googlenative.genomics.v1alpha2.inputs.PipelineResourcesArgs;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PipelineArgs extends com.pulumi.resources.ResourceArgs {
public static final PipelineArgs Empty = new PipelineArgs();
/**
* User-specified description.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return User-specified description.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy