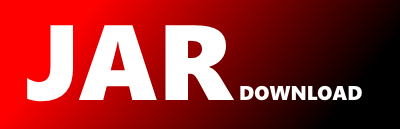
com.pulumi.googlenative.gkebackup.v1.outputs.GetBackupResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.gkebackup.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.gkebackup.v1.outputs.ClusterMetadataResponse;
import com.pulumi.googlenative.gkebackup.v1.outputs.EncryptionKeyResponse;
import com.pulumi.googlenative.gkebackup.v1.outputs.NamespacedNamesResponse;
import com.pulumi.googlenative.gkebackup.v1.outputs.NamespacesResponse;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetBackupResult {
/**
* @return If True, all namespaces were included in the Backup.
*
*/
private Boolean allNamespaces;
/**
* @return Information about the GKE cluster from which this Backup was created.
*
*/
private ClusterMetadataResponse clusterMetadata;
/**
* @return Completion time of the Backup
*
*/
private String completeTime;
/**
* @return The size of the config backup in bytes.
*
*/
private String configBackupSizeBytes;
/**
* @return Whether or not the Backup contains Kubernetes Secrets. Controlled by the parent BackupPlan's include_secrets value.
*
*/
private Boolean containsSecrets;
/**
* @return Whether or not the Backup contains volume data. Controlled by the parent BackupPlan's include_volume_data value.
*
*/
private Boolean containsVolumeData;
/**
* @return The timestamp when this Backup resource was created.
*
*/
private String createTime;
/**
* @return Minimum age for this Backup (in days). If this field is set to a non-zero value, the Backup will be "locked" against deletion (either manual or automatic deletion) for the number of days provided (measured from the creation time of the Backup). MUST be an integer value between 0-90 (inclusive). Defaults to parent BackupPlan's backup_delete_lock_days setting and may only be increased (either at creation time or in a subsequent update).
*
*/
private Integer deleteLockDays;
/**
* @return The time at which an existing delete lock will expire for this backup (calculated from create_time + delete_lock_days).
*
*/
private String deleteLockExpireTime;
/**
* @return User specified descriptive string for this Backup.
*
*/
private String description;
/**
* @return The customer managed encryption key that was used to encrypt the Backup's artifacts. Inherited from the parent BackupPlan's encryption_key value.
*
*/
private EncryptionKeyResponse encryptionKey;
/**
* @return `etag` is used for optimistic concurrency control as a way to help prevent simultaneous updates of a backup from overwriting each other. It is strongly suggested that systems make use of the `etag` in the read-modify-write cycle to perform backup updates in order to avoid race conditions: An `etag` is returned in the response to `GetBackup`, and systems are expected to put that etag in the request to `UpdateBackup` or `DeleteBackup` to ensure that their change will be applied to the same version of the resource.
*
*/
private String etag;
/**
* @return A set of custom labels supplied by user.
*
*/
private Map labels;
/**
* @return This flag indicates whether this Backup resource was created manually by a user or via a schedule in the BackupPlan. A value of True means that the Backup was created manually.
*
*/
private Boolean manual;
/**
* @return The fully qualified name of the Backup. `projects/*{@literal /}locations/*{@literal /}backupPlans/*{@literal /}backups/*`
*
*/
private String name;
/**
* @return The total number of Kubernetes Pods contained in the Backup.
*
*/
private Integer podCount;
/**
* @return The total number of Kubernetes resources included in the Backup.
*
*/
private Integer resourceCount;
/**
* @return The age (in days) after which this Backup will be automatically deleted. Must be an integer value >= 0: - If 0, no automatic deletion will occur for this Backup. - If not 0, this must be >= delete_lock_days and <= 365. Once a Backup is created, this value may only be increased. Defaults to the parent BackupPlan's backup_retain_days value.
*
*/
private Integer retainDays;
/**
* @return The time at which this Backup will be automatically deleted (calculated from create_time + retain_days).
*
*/
private String retainExpireTime;
/**
* @return If set, the list of ProtectedApplications whose resources were included in the Backup.
*
*/
private NamespacedNamesResponse selectedApplications;
/**
* @return If set, the list of namespaces that were included in the Backup.
*
*/
private NamespacesResponse selectedNamespaces;
/**
* @return The total size of the Backup in bytes = config backup size + sum(volume backup sizes)
*
*/
private String sizeBytes;
/**
* @return Current state of the Backup
*
*/
private String state;
/**
* @return Human-readable description of why the backup is in the current `state`.
*
*/
private String stateReason;
/**
* @return Server generated global unique identifier of [UUID4](https://en.wikipedia.org/wiki/Universally_unique_identifier)
*
*/
private String uid;
/**
* @return The timestamp when this Backup resource was last updated.
*
*/
private String updateTime;
/**
* @return The total number of volume backups contained in the Backup.
*
*/
private Integer volumeCount;
private GetBackupResult() {}
/**
* @return If True, all namespaces were included in the Backup.
*
*/
public Boolean allNamespaces() {
return this.allNamespaces;
}
/**
* @return Information about the GKE cluster from which this Backup was created.
*
*/
public ClusterMetadataResponse clusterMetadata() {
return this.clusterMetadata;
}
/**
* @return Completion time of the Backup
*
*/
public String completeTime() {
return this.completeTime;
}
/**
* @return The size of the config backup in bytes.
*
*/
public String configBackupSizeBytes() {
return this.configBackupSizeBytes;
}
/**
* @return Whether or not the Backup contains Kubernetes Secrets. Controlled by the parent BackupPlan's include_secrets value.
*
*/
public Boolean containsSecrets() {
return this.containsSecrets;
}
/**
* @return Whether or not the Backup contains volume data. Controlled by the parent BackupPlan's include_volume_data value.
*
*/
public Boolean containsVolumeData() {
return this.containsVolumeData;
}
/**
* @return The timestamp when this Backup resource was created.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return Minimum age for this Backup (in days). If this field is set to a non-zero value, the Backup will be "locked" against deletion (either manual or automatic deletion) for the number of days provided (measured from the creation time of the Backup). MUST be an integer value between 0-90 (inclusive). Defaults to parent BackupPlan's backup_delete_lock_days setting and may only be increased (either at creation time or in a subsequent update).
*
*/
public Integer deleteLockDays() {
return this.deleteLockDays;
}
/**
* @return The time at which an existing delete lock will expire for this backup (calculated from create_time + delete_lock_days).
*
*/
public String deleteLockExpireTime() {
return this.deleteLockExpireTime;
}
/**
* @return User specified descriptive string for this Backup.
*
*/
public String description() {
return this.description;
}
/**
* @return The customer managed encryption key that was used to encrypt the Backup's artifacts. Inherited from the parent BackupPlan's encryption_key value.
*
*/
public EncryptionKeyResponse encryptionKey() {
return this.encryptionKey;
}
/**
* @return `etag` is used for optimistic concurrency control as a way to help prevent simultaneous updates of a backup from overwriting each other. It is strongly suggested that systems make use of the `etag` in the read-modify-write cycle to perform backup updates in order to avoid race conditions: An `etag` is returned in the response to `GetBackup`, and systems are expected to put that etag in the request to `UpdateBackup` or `DeleteBackup` to ensure that their change will be applied to the same version of the resource.
*
*/
public String etag() {
return this.etag;
}
/**
* @return A set of custom labels supplied by user.
*
*/
public Map labels() {
return this.labels;
}
/**
* @return This flag indicates whether this Backup resource was created manually by a user or via a schedule in the BackupPlan. A value of True means that the Backup was created manually.
*
*/
public Boolean manual() {
return this.manual;
}
/**
* @return The fully qualified name of the Backup. `projects/*{@literal /}locations/*{@literal /}backupPlans/*{@literal /}backups/*`
*
*/
public String name() {
return this.name;
}
/**
* @return The total number of Kubernetes Pods contained in the Backup.
*
*/
public Integer podCount() {
return this.podCount;
}
/**
* @return The total number of Kubernetes resources included in the Backup.
*
*/
public Integer resourceCount() {
return this.resourceCount;
}
/**
* @return The age (in days) after which this Backup will be automatically deleted. Must be an integer value >= 0: - If 0, no automatic deletion will occur for this Backup. - If not 0, this must be >= delete_lock_days and <= 365. Once a Backup is created, this value may only be increased. Defaults to the parent BackupPlan's backup_retain_days value.
*
*/
public Integer retainDays() {
return this.retainDays;
}
/**
* @return The time at which this Backup will be automatically deleted (calculated from create_time + retain_days).
*
*/
public String retainExpireTime() {
return this.retainExpireTime;
}
/**
* @return If set, the list of ProtectedApplications whose resources were included in the Backup.
*
*/
public NamespacedNamesResponse selectedApplications() {
return this.selectedApplications;
}
/**
* @return If set, the list of namespaces that were included in the Backup.
*
*/
public NamespacesResponse selectedNamespaces() {
return this.selectedNamespaces;
}
/**
* @return The total size of the Backup in bytes = config backup size + sum(volume backup sizes)
*
*/
public String sizeBytes() {
return this.sizeBytes;
}
/**
* @return Current state of the Backup
*
*/
public String state() {
return this.state;
}
/**
* @return Human-readable description of why the backup is in the current `state`.
*
*/
public String stateReason() {
return this.stateReason;
}
/**
* @return Server generated global unique identifier of [UUID4](https://en.wikipedia.org/wiki/Universally_unique_identifier)
*
*/
public String uid() {
return this.uid;
}
/**
* @return The timestamp when this Backup resource was last updated.
*
*/
public String updateTime() {
return this.updateTime;
}
/**
* @return The total number of volume backups contained in the Backup.
*
*/
public Integer volumeCount() {
return this.volumeCount;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBackupResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Boolean allNamespaces;
private ClusterMetadataResponse clusterMetadata;
private String completeTime;
private String configBackupSizeBytes;
private Boolean containsSecrets;
private Boolean containsVolumeData;
private String createTime;
private Integer deleteLockDays;
private String deleteLockExpireTime;
private String description;
private EncryptionKeyResponse encryptionKey;
private String etag;
private Map labels;
private Boolean manual;
private String name;
private Integer podCount;
private Integer resourceCount;
private Integer retainDays;
private String retainExpireTime;
private NamespacedNamesResponse selectedApplications;
private NamespacesResponse selectedNamespaces;
private String sizeBytes;
private String state;
private String stateReason;
private String uid;
private String updateTime;
private Integer volumeCount;
public Builder() {}
public Builder(GetBackupResult defaults) {
Objects.requireNonNull(defaults);
this.allNamespaces = defaults.allNamespaces;
this.clusterMetadata = defaults.clusterMetadata;
this.completeTime = defaults.completeTime;
this.configBackupSizeBytes = defaults.configBackupSizeBytes;
this.containsSecrets = defaults.containsSecrets;
this.containsVolumeData = defaults.containsVolumeData;
this.createTime = defaults.createTime;
this.deleteLockDays = defaults.deleteLockDays;
this.deleteLockExpireTime = defaults.deleteLockExpireTime;
this.description = defaults.description;
this.encryptionKey = defaults.encryptionKey;
this.etag = defaults.etag;
this.labels = defaults.labels;
this.manual = defaults.manual;
this.name = defaults.name;
this.podCount = defaults.podCount;
this.resourceCount = defaults.resourceCount;
this.retainDays = defaults.retainDays;
this.retainExpireTime = defaults.retainExpireTime;
this.selectedApplications = defaults.selectedApplications;
this.selectedNamespaces = defaults.selectedNamespaces;
this.sizeBytes = defaults.sizeBytes;
this.state = defaults.state;
this.stateReason = defaults.stateReason;
this.uid = defaults.uid;
this.updateTime = defaults.updateTime;
this.volumeCount = defaults.volumeCount;
}
@CustomType.Setter
public Builder allNamespaces(Boolean allNamespaces) {
this.allNamespaces = Objects.requireNonNull(allNamespaces);
return this;
}
@CustomType.Setter
public Builder clusterMetadata(ClusterMetadataResponse clusterMetadata) {
this.clusterMetadata = Objects.requireNonNull(clusterMetadata);
return this;
}
@CustomType.Setter
public Builder completeTime(String completeTime) {
this.completeTime = Objects.requireNonNull(completeTime);
return this;
}
@CustomType.Setter
public Builder configBackupSizeBytes(String configBackupSizeBytes) {
this.configBackupSizeBytes = Objects.requireNonNull(configBackupSizeBytes);
return this;
}
@CustomType.Setter
public Builder containsSecrets(Boolean containsSecrets) {
this.containsSecrets = Objects.requireNonNull(containsSecrets);
return this;
}
@CustomType.Setter
public Builder containsVolumeData(Boolean containsVolumeData) {
this.containsVolumeData = Objects.requireNonNull(containsVolumeData);
return this;
}
@CustomType.Setter
public Builder createTime(String createTime) {
this.createTime = Objects.requireNonNull(createTime);
return this;
}
@CustomType.Setter
public Builder deleteLockDays(Integer deleteLockDays) {
this.deleteLockDays = Objects.requireNonNull(deleteLockDays);
return this;
}
@CustomType.Setter
public Builder deleteLockExpireTime(String deleteLockExpireTime) {
this.deleteLockExpireTime = Objects.requireNonNull(deleteLockExpireTime);
return this;
}
@CustomType.Setter
public Builder description(String description) {
this.description = Objects.requireNonNull(description);
return this;
}
@CustomType.Setter
public Builder encryptionKey(EncryptionKeyResponse encryptionKey) {
this.encryptionKey = Objects.requireNonNull(encryptionKey);
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
this.etag = Objects.requireNonNull(etag);
return this;
}
@CustomType.Setter
public Builder labels(Map labels) {
this.labels = Objects.requireNonNull(labels);
return this;
}
@CustomType.Setter
public Builder manual(Boolean manual) {
this.manual = Objects.requireNonNull(manual);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder podCount(Integer podCount) {
this.podCount = Objects.requireNonNull(podCount);
return this;
}
@CustomType.Setter
public Builder resourceCount(Integer resourceCount) {
this.resourceCount = Objects.requireNonNull(resourceCount);
return this;
}
@CustomType.Setter
public Builder retainDays(Integer retainDays) {
this.retainDays = Objects.requireNonNull(retainDays);
return this;
}
@CustomType.Setter
public Builder retainExpireTime(String retainExpireTime) {
this.retainExpireTime = Objects.requireNonNull(retainExpireTime);
return this;
}
@CustomType.Setter
public Builder selectedApplications(NamespacedNamesResponse selectedApplications) {
this.selectedApplications = Objects.requireNonNull(selectedApplications);
return this;
}
@CustomType.Setter
public Builder selectedNamespaces(NamespacesResponse selectedNamespaces) {
this.selectedNamespaces = Objects.requireNonNull(selectedNamespaces);
return this;
}
@CustomType.Setter
public Builder sizeBytes(String sizeBytes) {
this.sizeBytes = Objects.requireNonNull(sizeBytes);
return this;
}
@CustomType.Setter
public Builder state(String state) {
this.state = Objects.requireNonNull(state);
return this;
}
@CustomType.Setter
public Builder stateReason(String stateReason) {
this.stateReason = Objects.requireNonNull(stateReason);
return this;
}
@CustomType.Setter
public Builder uid(String uid) {
this.uid = Objects.requireNonNull(uid);
return this;
}
@CustomType.Setter
public Builder updateTime(String updateTime) {
this.updateTime = Objects.requireNonNull(updateTime);
return this;
}
@CustomType.Setter
public Builder volumeCount(Integer volumeCount) {
this.volumeCount = Objects.requireNonNull(volumeCount);
return this;
}
public GetBackupResult build() {
final var o = new GetBackupResult();
o.allNamespaces = allNamespaces;
o.clusterMetadata = clusterMetadata;
o.completeTime = completeTime;
o.configBackupSizeBytes = configBackupSizeBytes;
o.containsSecrets = containsSecrets;
o.containsVolumeData = containsVolumeData;
o.createTime = createTime;
o.deleteLockDays = deleteLockDays;
o.deleteLockExpireTime = deleteLockExpireTime;
o.description = description;
o.encryptionKey = encryptionKey;
o.etag = etag;
o.labels = labels;
o.manual = manual;
o.name = name;
o.podCount = podCount;
o.resourceCount = resourceCount;
o.retainDays = retainDays;
o.retainExpireTime = retainExpireTime;
o.selectedApplications = selectedApplications;
o.selectedNamespaces = selectedNamespaces;
o.sizeBytes = sizeBytes;
o.state = state;
o.stateReason = stateReason;
o.uid = uid;
o.updateTime = updateTime;
o.volumeCount = volumeCount;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy