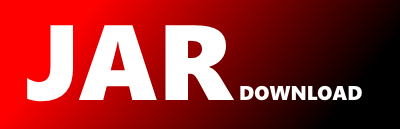
com.pulumi.googlenative.gkehub.v1alpha.FeatureIamBindingArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.gkehub.v1alpha;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.iam.v1.inputs.ConditionArgs;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class FeatureIamBindingArgs extends com.pulumi.resources.ResourceArgs {
public static final FeatureIamBindingArgs Empty = new FeatureIamBindingArgs();
/**
* An IAM Condition for a given binding.
*
*/
@Import(name="condition")
private @Nullable Output condition;
/**
* @return An IAM Condition for a given binding.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy