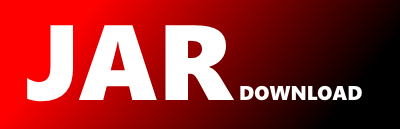
com.pulumi.googlenative.healthcare.v1beta1.AttributeDefinitionArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.healthcare.v1beta1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.healthcare.v1beta1.enums.AttributeDefinitionCategory;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AttributeDefinitionArgs extends com.pulumi.resources.ResourceArgs {
public static final AttributeDefinitionArgs Empty = new AttributeDefinitionArgs();
/**
* Possible values for the attribute. The number of allowed values must not exceed 500. An empty list is invalid. The list can only be expanded after creation.
*
*/
@Import(name="allowedValues", required=true)
private Output> allowedValues;
/**
* @return Possible values for the attribute. The number of allowed values must not exceed 500. An empty list is invalid. The list can only be expanded after creation.
*
*/
public Output> allowedValues() {
return this.allowedValues;
}
/**
* Required. The ID of the Attribute definition to create. The string must match the following regex: `_a-zA-Z{0,255}` and must not be a reserved keyword within the Common Expression Language as listed on https://github.com/google/cel-spec/blob/master/doc/langdef.md.
*
*/
@Import(name="attributeDefinitionId", required=true)
private Output attributeDefinitionId;
/**
* @return Required. The ID of the Attribute definition to create. The string must match the following regex: `_a-zA-Z{0,255}` and must not be a reserved keyword within the Common Expression Language as listed on https://github.com/google/cel-spec/blob/master/doc/langdef.md.
*
*/
public Output attributeDefinitionId() {
return this.attributeDefinitionId;
}
/**
* The category of the attribute. The value of this field cannot be changed after creation.
*
*/
@Import(name="category", required=true)
private Output category;
/**
* @return The category of the attribute. The value of this field cannot be changed after creation.
*
*/
public Output category() {
return this.category;
}
/**
* Optional. Default values of the attribute in Consents. If no default values are specified, it defaults to an empty value.
*
*/
@Import(name="consentDefaultValues")
private @Nullable Output> consentDefaultValues;
/**
* @return Optional. Default values of the attribute in Consents. If no default values are specified, it defaults to an empty value.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy