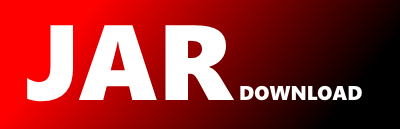
com.pulumi.googlenative.integrations.v1alpha.outputs.GoogleCloudIntegrationsV1alphaIntegrationAlertConfigResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.integrations.v1alpha.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.integrations.v1alpha.outputs.GoogleCloudIntegrationsV1alphaIntegrationAlertConfigThresholdValueResponse;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GoogleCloudIntegrationsV1alphaIntegrationAlertConfigResponse {
/**
* @return The period over which the metric value should be aggregated and evaluated. Format is , where integer should be a positive integer and unit should be one of (s,m,h,d,w) meaning (second, minute, hour, day, week). For an EXPECTED_MIN threshold, this aggregation_period must be lesser than 24 hours.
*
*/
private String aggregationPeriod;
/**
* @return For how many contiguous aggregation periods should the expected min or max be violated for the alert to be fired.
*
*/
private Integer alertThreshold;
/**
* @return Set to false by default. When set to true, the metrics are not aggregated or pushed to Monarch for this integration alert.
*
*/
private Boolean disableAlert;
/**
* @return Name of the alert. This will be displayed in the alert subject. If set, this name should be unique within the scope of the integration.
*
*/
private String displayName;
/**
* @return Should be specified only for *AVERAGE_DURATION and *PERCENTILE_DURATION metrics. This member should be used to specify what duration value the metrics should exceed for the alert to trigger.
*
*/
private String durationThreshold;
/**
* @return The type of metric.
*
*/
private String metricType;
/**
* @return For either events or tasks, depending on the type of alert, count only final attempts, not retries.
*
*/
private Boolean onlyFinalAttempt;
/**
* @return The threshold type, whether lower(expected_min) or upper(expected_max), for which this alert is being configured. If value falls below expected_min or exceeds expected_max, an alert will be fired.
*
*/
private String thresholdType;
/**
* @return The metric value, above or below which the alert should be triggered.
*
*/
private GoogleCloudIntegrationsV1alphaIntegrationAlertConfigThresholdValueResponse thresholdValue;
private GoogleCloudIntegrationsV1alphaIntegrationAlertConfigResponse() {}
/**
* @return The period over which the metric value should be aggregated and evaluated. Format is , where integer should be a positive integer and unit should be one of (s,m,h,d,w) meaning (second, minute, hour, day, week). For an EXPECTED_MIN threshold, this aggregation_period must be lesser than 24 hours.
*
*/
public String aggregationPeriod() {
return this.aggregationPeriod;
}
/**
* @return For how many contiguous aggregation periods should the expected min or max be violated for the alert to be fired.
*
*/
public Integer alertThreshold() {
return this.alertThreshold;
}
/**
* @return Set to false by default. When set to true, the metrics are not aggregated or pushed to Monarch for this integration alert.
*
*/
public Boolean disableAlert() {
return this.disableAlert;
}
/**
* @return Name of the alert. This will be displayed in the alert subject. If set, this name should be unique within the scope of the integration.
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return Should be specified only for *AVERAGE_DURATION and *PERCENTILE_DURATION metrics. This member should be used to specify what duration value the metrics should exceed for the alert to trigger.
*
*/
public String durationThreshold() {
return this.durationThreshold;
}
/**
* @return The type of metric.
*
*/
public String metricType() {
return this.metricType;
}
/**
* @return For either events or tasks, depending on the type of alert, count only final attempts, not retries.
*
*/
public Boolean onlyFinalAttempt() {
return this.onlyFinalAttempt;
}
/**
* @return The threshold type, whether lower(expected_min) or upper(expected_max), for which this alert is being configured. If value falls below expected_min or exceeds expected_max, an alert will be fired.
*
*/
public String thresholdType() {
return this.thresholdType;
}
/**
* @return The metric value, above or below which the alert should be triggered.
*
*/
public GoogleCloudIntegrationsV1alphaIntegrationAlertConfigThresholdValueResponse thresholdValue() {
return this.thresholdValue;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GoogleCloudIntegrationsV1alphaIntegrationAlertConfigResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String aggregationPeriod;
private Integer alertThreshold;
private Boolean disableAlert;
private String displayName;
private String durationThreshold;
private String metricType;
private Boolean onlyFinalAttempt;
private String thresholdType;
private GoogleCloudIntegrationsV1alphaIntegrationAlertConfigThresholdValueResponse thresholdValue;
public Builder() {}
public Builder(GoogleCloudIntegrationsV1alphaIntegrationAlertConfigResponse defaults) {
Objects.requireNonNull(defaults);
this.aggregationPeriod = defaults.aggregationPeriod;
this.alertThreshold = defaults.alertThreshold;
this.disableAlert = defaults.disableAlert;
this.displayName = defaults.displayName;
this.durationThreshold = defaults.durationThreshold;
this.metricType = defaults.metricType;
this.onlyFinalAttempt = defaults.onlyFinalAttempt;
this.thresholdType = defaults.thresholdType;
this.thresholdValue = defaults.thresholdValue;
}
@CustomType.Setter
public Builder aggregationPeriod(String aggregationPeriod) {
this.aggregationPeriod = Objects.requireNonNull(aggregationPeriod);
return this;
}
@CustomType.Setter
public Builder alertThreshold(Integer alertThreshold) {
this.alertThreshold = Objects.requireNonNull(alertThreshold);
return this;
}
@CustomType.Setter
public Builder disableAlert(Boolean disableAlert) {
this.disableAlert = Objects.requireNonNull(disableAlert);
return this;
}
@CustomType.Setter
public Builder displayName(String displayName) {
this.displayName = Objects.requireNonNull(displayName);
return this;
}
@CustomType.Setter
public Builder durationThreshold(String durationThreshold) {
this.durationThreshold = Objects.requireNonNull(durationThreshold);
return this;
}
@CustomType.Setter
public Builder metricType(String metricType) {
this.metricType = Objects.requireNonNull(metricType);
return this;
}
@CustomType.Setter
public Builder onlyFinalAttempt(Boolean onlyFinalAttempt) {
this.onlyFinalAttempt = Objects.requireNonNull(onlyFinalAttempt);
return this;
}
@CustomType.Setter
public Builder thresholdType(String thresholdType) {
this.thresholdType = Objects.requireNonNull(thresholdType);
return this;
}
@CustomType.Setter
public Builder thresholdValue(GoogleCloudIntegrationsV1alphaIntegrationAlertConfigThresholdValueResponse thresholdValue) {
this.thresholdValue = Objects.requireNonNull(thresholdValue);
return this;
}
public GoogleCloudIntegrationsV1alphaIntegrationAlertConfigResponse build() {
final var o = new GoogleCloudIntegrationsV1alphaIntegrationAlertConfigResponse();
o.aggregationPeriod = aggregationPeriod;
o.alertThreshold = alertThreshold;
o.disableAlert = disableAlert;
o.displayName = displayName;
o.durationThreshold = durationThreshold;
o.metricType = metricType;
o.onlyFinalAttempt = onlyFinalAttempt;
o.thresholdType = thresholdType;
o.thresholdValue = thresholdValue;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy