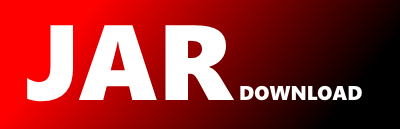
com.pulumi.googlenative.managedidentities.v1alpha1.outputs.TrustResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.managedidentities.v1alpha1.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class TrustResponse {
/**
* @return The time the instance was created.
*
*/
private String createTime;
/**
* @return The last heartbeat time when the trust was known to be connected.
*
*/
private String lastKnownTrustConnectedHeartbeatTime;
/**
* @return The trust authentication type which decides whether the trusted side has forest/domain wide access or selective access to approved set of resources.
*
*/
private Boolean selectiveAuthentication;
/**
* @return The current state of this trust.
*
*/
private String state;
/**
* @return Additional information about the current state of this trust, if available.
*
*/
private String stateDescription;
/**
* @return The target dns server ip addresses which can resolve the remote domain involved in trust.
*
*/
private List targetDnsIpAddresses;
/**
* @return The fully qualified target domain name which will be in trust with current domain.
*
*/
private String targetDomainName;
/**
* @return The trust direction decides the current domain is trusted, trusting or both.
*
*/
private String trustDirection;
/**
* @return Input only, and will not be stored. The trust secret used for handshake with target domain.
*
*/
private String trustHandshakeSecret;
/**
* @return The type of trust represented by the trust resource.
*
*/
private String trustType;
/**
* @return Last update time.
*
*/
private String updateTime;
private TrustResponse() {}
/**
* @return The time the instance was created.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return The last heartbeat time when the trust was known to be connected.
*
*/
public String lastKnownTrustConnectedHeartbeatTime() {
return this.lastKnownTrustConnectedHeartbeatTime;
}
/**
* @return The trust authentication type which decides whether the trusted side has forest/domain wide access or selective access to approved set of resources.
*
*/
public Boolean selectiveAuthentication() {
return this.selectiveAuthentication;
}
/**
* @return The current state of this trust.
*
*/
public String state() {
return this.state;
}
/**
* @return Additional information about the current state of this trust, if available.
*
*/
public String stateDescription() {
return this.stateDescription;
}
/**
* @return The target dns server ip addresses which can resolve the remote domain involved in trust.
*
*/
public List targetDnsIpAddresses() {
return this.targetDnsIpAddresses;
}
/**
* @return The fully qualified target domain name which will be in trust with current domain.
*
*/
public String targetDomainName() {
return this.targetDomainName;
}
/**
* @return The trust direction decides the current domain is trusted, trusting or both.
*
*/
public String trustDirection() {
return this.trustDirection;
}
/**
* @return Input only, and will not be stored. The trust secret used for handshake with target domain.
*
*/
public String trustHandshakeSecret() {
return this.trustHandshakeSecret;
}
/**
* @return The type of trust represented by the trust resource.
*
*/
public String trustType() {
return this.trustType;
}
/**
* @return Last update time.
*
*/
public String updateTime() {
return this.updateTime;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(TrustResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String createTime;
private String lastKnownTrustConnectedHeartbeatTime;
private Boolean selectiveAuthentication;
private String state;
private String stateDescription;
private List targetDnsIpAddresses;
private String targetDomainName;
private String trustDirection;
private String trustHandshakeSecret;
private String trustType;
private String updateTime;
public Builder() {}
public Builder(TrustResponse defaults) {
Objects.requireNonNull(defaults);
this.createTime = defaults.createTime;
this.lastKnownTrustConnectedHeartbeatTime = defaults.lastKnownTrustConnectedHeartbeatTime;
this.selectiveAuthentication = defaults.selectiveAuthentication;
this.state = defaults.state;
this.stateDescription = defaults.stateDescription;
this.targetDnsIpAddresses = defaults.targetDnsIpAddresses;
this.targetDomainName = defaults.targetDomainName;
this.trustDirection = defaults.trustDirection;
this.trustHandshakeSecret = defaults.trustHandshakeSecret;
this.trustType = defaults.trustType;
this.updateTime = defaults.updateTime;
}
@CustomType.Setter
public Builder createTime(String createTime) {
this.createTime = Objects.requireNonNull(createTime);
return this;
}
@CustomType.Setter
public Builder lastKnownTrustConnectedHeartbeatTime(String lastKnownTrustConnectedHeartbeatTime) {
this.lastKnownTrustConnectedHeartbeatTime = Objects.requireNonNull(lastKnownTrustConnectedHeartbeatTime);
return this;
}
@CustomType.Setter
public Builder selectiveAuthentication(Boolean selectiveAuthentication) {
this.selectiveAuthentication = Objects.requireNonNull(selectiveAuthentication);
return this;
}
@CustomType.Setter
public Builder state(String state) {
this.state = Objects.requireNonNull(state);
return this;
}
@CustomType.Setter
public Builder stateDescription(String stateDescription) {
this.stateDescription = Objects.requireNonNull(stateDescription);
return this;
}
@CustomType.Setter
public Builder targetDnsIpAddresses(List targetDnsIpAddresses) {
this.targetDnsIpAddresses = Objects.requireNonNull(targetDnsIpAddresses);
return this;
}
public Builder targetDnsIpAddresses(String... targetDnsIpAddresses) {
return targetDnsIpAddresses(List.of(targetDnsIpAddresses));
}
@CustomType.Setter
public Builder targetDomainName(String targetDomainName) {
this.targetDomainName = Objects.requireNonNull(targetDomainName);
return this;
}
@CustomType.Setter
public Builder trustDirection(String trustDirection) {
this.trustDirection = Objects.requireNonNull(trustDirection);
return this;
}
@CustomType.Setter
public Builder trustHandshakeSecret(String trustHandshakeSecret) {
this.trustHandshakeSecret = Objects.requireNonNull(trustHandshakeSecret);
return this;
}
@CustomType.Setter
public Builder trustType(String trustType) {
this.trustType = Objects.requireNonNull(trustType);
return this;
}
@CustomType.Setter
public Builder updateTime(String updateTime) {
this.updateTime = Objects.requireNonNull(updateTime);
return this;
}
public TrustResponse build() {
final var o = new TrustResponse();
o.createTime = createTime;
o.lastKnownTrustConnectedHeartbeatTime = lastKnownTrustConnectedHeartbeatTime;
o.selectiveAuthentication = selectiveAuthentication;
o.state = state;
o.stateDescription = stateDescription;
o.targetDnsIpAddresses = targetDnsIpAddresses;
o.targetDomainName = targetDomainName;
o.trustDirection = trustDirection;
o.trustHandshakeSecret = trustHandshakeSecret;
o.trustType = trustType;
o.updateTime = updateTime;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy