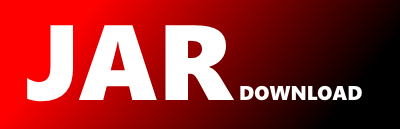
com.pulumi.googlenative.managedidentities.v1beta1.DomainBackupIamBinding Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.managedidentities.v1beta1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.googlenative.Utilities;
import com.pulumi.googlenative.iam.v1.outputs.Condition;
import com.pulumi.googlenative.managedidentities.v1beta1.DomainBackupIamBindingArgs;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
*/
@ResourceType(type="google-native:managedidentities/v1beta1:DomainBackupIamBinding")
public class DomainBackupIamBinding extends com.pulumi.resources.CustomResource {
/**
* An IAM Condition for a given binding. See https://cloud.google.com/iam/docs/conditions-overview for additional details.
*
*/
@Export(name="condition", type=Condition.class, parameters={})
private Output* @Nullable */ Condition> condition;
/**
* @return An IAM Condition for a given binding. See https://cloud.google.com/iam/docs/conditions-overview for additional details.
*
*/
public Output> condition() {
return Codegen.optional(this.condition);
}
/**
* The etag of the resource's IAM policy.
*
*/
@Export(name="etag", type=String.class, parameters={})
private Output etag;
/**
* @return The etag of the resource's IAM policy.
*
*/
public Output etag() {
return this.etag;
}
/**
* Specifies the principals requesting access for a Google Cloud resource. `members` can have the following values: * `allUsers`: A special identifier that represents anyone who is on the internet; with or without a Google account. * `allAuthenticatedUsers`: A special identifier that represents anyone who is authenticated with a Google account or a service account. Does not include identities that come from external identity providers (IdPs) through identity federation. * `user:{emailid}`: An email address that represents a specific Google account. For example, `[email protected]` . * `serviceAccount:{emailid}`: An email address that represents a Google service account. For example, `[email protected]`. * `serviceAccount:{projectid}.svc.id.goog[{namespace}/{kubernetes-sa}]`: An identifier for a [Kubernetes service account](https://cloud.google.com/kubernetes-engine/docs/how-to/kubernetes-service-accounts). For example, `my-project.svc.id.goog[my-namespace/my-kubernetes-sa]`. * `group:{emailid}`: An email address that represents a Google group. For example, `[email protected]`. * `domain:{domain}`: The G Suite domain (primary) that represents all the users of that domain. For example, `google.com` or `example.com`. * `deleted:user:{emailid}?uid={uniqueid}`: An email address (plus unique identifier) representing a user that has been recently deleted. For example, `[email protected]?uid=123456789012345678901`. If the user is recovered, this value reverts to `user:{emailid}` and the recovered user retains the role in the binding. * `deleted:serviceAccount:{emailid}?uid={uniqueid}`: An email address (plus unique identifier) representing a service account that has been recently deleted. For example, `[email protected]?uid=123456789012345678901`. If the service account is undeleted, this value reverts to `serviceAccount:{emailid}` and the undeleted service account retains the role in the binding. * `deleted:group:{emailid}?uid={uniqueid}`: An email address (plus unique identifier) representing a Google group that has been recently deleted. For example, `[email protected]?uid=123456789012345678901`. If the group is recovered, this value reverts to `group:{emailid}` and the recovered group retains the role in the binding.
*
*/
@Export(name="members", type=List.class, parameters={String.class})
private Output> members;
/**
* @return Specifies the principals requesting access for a Google Cloud resource. `members` can have the following values: * `allUsers`: A special identifier that represents anyone who is on the internet; with or without a Google account. * `allAuthenticatedUsers`: A special identifier that represents anyone who is authenticated with a Google account or a service account. Does not include identities that come from external identity providers (IdPs) through identity federation. * `user:{emailid}`: An email address that represents a specific Google account. For example, `[email protected]` . * `serviceAccount:{emailid}`: An email address that represents a Google service account. For example, `[email protected]`. * `serviceAccount:{projectid}.svc.id.goog[{namespace}/{kubernetes-sa}]`: An identifier for a [Kubernetes service account](https://cloud.google.com/kubernetes-engine/docs/how-to/kubernetes-service-accounts). For example, `my-project.svc.id.goog[my-namespace/my-kubernetes-sa]`. * `group:{emailid}`: An email address that represents a Google group. For example, `[email protected]`. * `domain:{domain}`: The G Suite domain (primary) that represents all the users of that domain. For example, `google.com` or `example.com`. * `deleted:user:{emailid}?uid={uniqueid}`: An email address (plus unique identifier) representing a user that has been recently deleted. For example, `[email protected]?uid=123456789012345678901`. If the user is recovered, this value reverts to `user:{emailid}` and the recovered user retains the role in the binding. * `deleted:serviceAccount:{emailid}?uid={uniqueid}`: An email address (plus unique identifier) representing a service account that has been recently deleted. For example, `[email protected]?uid=123456789012345678901`. If the service account is undeleted, this value reverts to `serviceAccount:{emailid}` and the undeleted service account retains the role in the binding. * `deleted:group:{emailid}?uid={uniqueid}`: An email address (plus unique identifier) representing a Google group that has been recently deleted. For example, `[email protected]?uid=123456789012345678901`. If the group is recovered, this value reverts to `group:{emailid}` and the recovered group retains the role in the binding.
*
*/
public Output> members() {
return this.members;
}
/**
* The name of the resource to manage IAM policies for.
*
*/
@Export(name="name", type=String.class, parameters={})
private Output name;
/**
* @return The name of the resource to manage IAM policies for.
*
*/
public Output name() {
return this.name;
}
/**
* The project in which the resource belongs. If it is not provided, a default will be supplied.
*
*/
@Export(name="project", type=String.class, parameters={})
private Output project;
/**
* @return The project in which the resource belongs. If it is not provided, a default will be supplied.
*
*/
public Output project() {
return this.project;
}
/**
* Role that is assigned to the list of `members`, or principals. For example, `roles/viewer`, `roles/editor`, or `roles/owner`.
*
*/
@Export(name="role", type=String.class, parameters={})
private Output role;
/**
* @return Role that is assigned to the list of `members`, or principals. For example, `roles/viewer`, `roles/editor`, or `roles/owner`.
*
*/
public Output role() {
return this.role;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public DomainBackupIamBinding(String name) {
this(name, DomainBackupIamBindingArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public DomainBackupIamBinding(String name, DomainBackupIamBindingArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public DomainBackupIamBinding(String name, DomainBackupIamBindingArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:managedidentities/v1beta1:DomainBackupIamBinding", name, args == null ? DomainBackupIamBindingArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private DomainBackupIamBinding(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:managedidentities/v1beta1:DomainBackupIamBinding", name, null, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static DomainBackupIamBinding get(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new DomainBackupIamBinding(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy