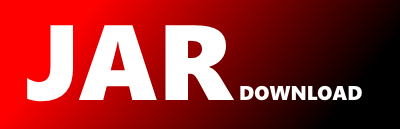
com.pulumi.googlenative.metastore.v1.outputs.ServiceResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.metastore.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.metastore.v1.outputs.EncryptionConfigResponse;
import com.pulumi.googlenative.metastore.v1.outputs.HiveMetastoreConfigResponse;
import com.pulumi.googlenative.metastore.v1.outputs.MaintenanceWindowResponse;
import com.pulumi.googlenative.metastore.v1.outputs.MetadataManagementActivityResponse;
import com.pulumi.googlenative.metastore.v1.outputs.NetworkConfigResponse;
import com.pulumi.googlenative.metastore.v1.outputs.ScalingConfigResponse;
import com.pulumi.googlenative.metastore.v1.outputs.TelemetryConfigResponse;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class ServiceResponse {
/**
* @return A Cloud Storage URI (starting with gs://) that specifies where artifacts related to the metastore service are stored.
*
*/
private String artifactGcsUri;
/**
* @return The time when the metastore service was created.
*
*/
private String createTime;
/**
* @return Immutable. The database type that the Metastore service stores its data.
*
*/
private String databaseType;
/**
* @return Immutable. Information used to configure the Dataproc Metastore service to encrypt customer data at rest. Cannot be updated.
*
*/
private EncryptionConfigResponse encryptionConfig;
/**
* @return The URI of the endpoint used to access the metastore service.
*
*/
private String endpointUri;
/**
* @return Configuration information specific to running Hive metastore software as the metastore service.
*
*/
private HiveMetastoreConfigResponse hiveMetastoreConfig;
/**
* @return User-defined labels for the metastore service.
*
*/
private Map labels;
/**
* @return The one hour maintenance window of the metastore service. This specifies when the service can be restarted for maintenance purposes in UTC time. Maintenance window is not needed for services with the SPANNER database type.
*
*/
private MaintenanceWindowResponse maintenanceWindow;
/**
* @return The metadata management activities of the metastore service.
*
*/
private MetadataManagementActivityResponse metadataManagementActivity;
/**
* @return Immutable. The relative resource name of the metastore service, in the following format:projects/{project_number}/locations/{location_id}/services/{service_id}.
*
*/
private String name;
/**
* @return Immutable. The relative resource name of the VPC network on which the instance can be accessed. It is specified in the following form:projects/{project_number}/global/networks/{network_id}.
*
*/
private String network;
/**
* @return The configuration specifying the network settings for the Dataproc Metastore service.
*
*/
private NetworkConfigResponse networkConfig;
/**
* @return The TCP port at which the metastore service is reached. Default: 9083.
*
*/
private Integer port;
/**
* @return Immutable. The release channel of the service. If unspecified, defaults to STABLE.
*
*/
private String releaseChannel;
/**
* @return Scaling configuration of the metastore service.
*
*/
private ScalingConfigResponse scalingConfig;
/**
* @return The current state of the metastore service.
*
*/
private String state;
/**
* @return Additional information about the current state of the metastore service, if available.
*
*/
private String stateMessage;
/**
* @return The configuration specifying telemetry settings for the Dataproc Metastore service. If unspecified defaults to JSON.
*
*/
private TelemetryConfigResponse telemetryConfig;
/**
* @return The tier of the service.
*
*/
private String tier;
/**
* @return The globally unique resource identifier of the metastore service.
*
*/
private String uid;
/**
* @return The time when the metastore service was last updated.
*
*/
private String updateTime;
private ServiceResponse() {}
/**
* @return A Cloud Storage URI (starting with gs://) that specifies where artifacts related to the metastore service are stored.
*
*/
public String artifactGcsUri() {
return this.artifactGcsUri;
}
/**
* @return The time when the metastore service was created.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return Immutable. The database type that the Metastore service stores its data.
*
*/
public String databaseType() {
return this.databaseType;
}
/**
* @return Immutable. Information used to configure the Dataproc Metastore service to encrypt customer data at rest. Cannot be updated.
*
*/
public EncryptionConfigResponse encryptionConfig() {
return this.encryptionConfig;
}
/**
* @return The URI of the endpoint used to access the metastore service.
*
*/
public String endpointUri() {
return this.endpointUri;
}
/**
* @return Configuration information specific to running Hive metastore software as the metastore service.
*
*/
public HiveMetastoreConfigResponse hiveMetastoreConfig() {
return this.hiveMetastoreConfig;
}
/**
* @return User-defined labels for the metastore service.
*
*/
public Map labels() {
return this.labels;
}
/**
* @return The one hour maintenance window of the metastore service. This specifies when the service can be restarted for maintenance purposes in UTC time. Maintenance window is not needed for services with the SPANNER database type.
*
*/
public MaintenanceWindowResponse maintenanceWindow() {
return this.maintenanceWindow;
}
/**
* @return The metadata management activities of the metastore service.
*
*/
public MetadataManagementActivityResponse metadataManagementActivity() {
return this.metadataManagementActivity;
}
/**
* @return Immutable. The relative resource name of the metastore service, in the following format:projects/{project_number}/locations/{location_id}/services/{service_id}.
*
*/
public String name() {
return this.name;
}
/**
* @return Immutable. The relative resource name of the VPC network on which the instance can be accessed. It is specified in the following form:projects/{project_number}/global/networks/{network_id}.
*
*/
public String network() {
return this.network;
}
/**
* @return The configuration specifying the network settings for the Dataproc Metastore service.
*
*/
public NetworkConfigResponse networkConfig() {
return this.networkConfig;
}
/**
* @return The TCP port at which the metastore service is reached. Default: 9083.
*
*/
public Integer port() {
return this.port;
}
/**
* @return Immutable. The release channel of the service. If unspecified, defaults to STABLE.
*
*/
public String releaseChannel() {
return this.releaseChannel;
}
/**
* @return Scaling configuration of the metastore service.
*
*/
public ScalingConfigResponse scalingConfig() {
return this.scalingConfig;
}
/**
* @return The current state of the metastore service.
*
*/
public String state() {
return this.state;
}
/**
* @return Additional information about the current state of the metastore service, if available.
*
*/
public String stateMessage() {
return this.stateMessage;
}
/**
* @return The configuration specifying telemetry settings for the Dataproc Metastore service. If unspecified defaults to JSON.
*
*/
public TelemetryConfigResponse telemetryConfig() {
return this.telemetryConfig;
}
/**
* @return The tier of the service.
*
*/
public String tier() {
return this.tier;
}
/**
* @return The globally unique resource identifier of the metastore service.
*
*/
public String uid() {
return this.uid;
}
/**
* @return The time when the metastore service was last updated.
*
*/
public String updateTime() {
return this.updateTime;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ServiceResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String artifactGcsUri;
private String createTime;
private String databaseType;
private EncryptionConfigResponse encryptionConfig;
private String endpointUri;
private HiveMetastoreConfigResponse hiveMetastoreConfig;
private Map labels;
private MaintenanceWindowResponse maintenanceWindow;
private MetadataManagementActivityResponse metadataManagementActivity;
private String name;
private String network;
private NetworkConfigResponse networkConfig;
private Integer port;
private String releaseChannel;
private ScalingConfigResponse scalingConfig;
private String state;
private String stateMessage;
private TelemetryConfigResponse telemetryConfig;
private String tier;
private String uid;
private String updateTime;
public Builder() {}
public Builder(ServiceResponse defaults) {
Objects.requireNonNull(defaults);
this.artifactGcsUri = defaults.artifactGcsUri;
this.createTime = defaults.createTime;
this.databaseType = defaults.databaseType;
this.encryptionConfig = defaults.encryptionConfig;
this.endpointUri = defaults.endpointUri;
this.hiveMetastoreConfig = defaults.hiveMetastoreConfig;
this.labels = defaults.labels;
this.maintenanceWindow = defaults.maintenanceWindow;
this.metadataManagementActivity = defaults.metadataManagementActivity;
this.name = defaults.name;
this.network = defaults.network;
this.networkConfig = defaults.networkConfig;
this.port = defaults.port;
this.releaseChannel = defaults.releaseChannel;
this.scalingConfig = defaults.scalingConfig;
this.state = defaults.state;
this.stateMessage = defaults.stateMessage;
this.telemetryConfig = defaults.telemetryConfig;
this.tier = defaults.tier;
this.uid = defaults.uid;
this.updateTime = defaults.updateTime;
}
@CustomType.Setter
public Builder artifactGcsUri(String artifactGcsUri) {
this.artifactGcsUri = Objects.requireNonNull(artifactGcsUri);
return this;
}
@CustomType.Setter
public Builder createTime(String createTime) {
this.createTime = Objects.requireNonNull(createTime);
return this;
}
@CustomType.Setter
public Builder databaseType(String databaseType) {
this.databaseType = Objects.requireNonNull(databaseType);
return this;
}
@CustomType.Setter
public Builder encryptionConfig(EncryptionConfigResponse encryptionConfig) {
this.encryptionConfig = Objects.requireNonNull(encryptionConfig);
return this;
}
@CustomType.Setter
public Builder endpointUri(String endpointUri) {
this.endpointUri = Objects.requireNonNull(endpointUri);
return this;
}
@CustomType.Setter
public Builder hiveMetastoreConfig(HiveMetastoreConfigResponse hiveMetastoreConfig) {
this.hiveMetastoreConfig = Objects.requireNonNull(hiveMetastoreConfig);
return this;
}
@CustomType.Setter
public Builder labels(Map labels) {
this.labels = Objects.requireNonNull(labels);
return this;
}
@CustomType.Setter
public Builder maintenanceWindow(MaintenanceWindowResponse maintenanceWindow) {
this.maintenanceWindow = Objects.requireNonNull(maintenanceWindow);
return this;
}
@CustomType.Setter
public Builder metadataManagementActivity(MetadataManagementActivityResponse metadataManagementActivity) {
this.metadataManagementActivity = Objects.requireNonNull(metadataManagementActivity);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder network(String network) {
this.network = Objects.requireNonNull(network);
return this;
}
@CustomType.Setter
public Builder networkConfig(NetworkConfigResponse networkConfig) {
this.networkConfig = Objects.requireNonNull(networkConfig);
return this;
}
@CustomType.Setter
public Builder port(Integer port) {
this.port = Objects.requireNonNull(port);
return this;
}
@CustomType.Setter
public Builder releaseChannel(String releaseChannel) {
this.releaseChannel = Objects.requireNonNull(releaseChannel);
return this;
}
@CustomType.Setter
public Builder scalingConfig(ScalingConfigResponse scalingConfig) {
this.scalingConfig = Objects.requireNonNull(scalingConfig);
return this;
}
@CustomType.Setter
public Builder state(String state) {
this.state = Objects.requireNonNull(state);
return this;
}
@CustomType.Setter
public Builder stateMessage(String stateMessage) {
this.stateMessage = Objects.requireNonNull(stateMessage);
return this;
}
@CustomType.Setter
public Builder telemetryConfig(TelemetryConfigResponse telemetryConfig) {
this.telemetryConfig = Objects.requireNonNull(telemetryConfig);
return this;
}
@CustomType.Setter
public Builder tier(String tier) {
this.tier = Objects.requireNonNull(tier);
return this;
}
@CustomType.Setter
public Builder uid(String uid) {
this.uid = Objects.requireNonNull(uid);
return this;
}
@CustomType.Setter
public Builder updateTime(String updateTime) {
this.updateTime = Objects.requireNonNull(updateTime);
return this;
}
public ServiceResponse build() {
final var o = new ServiceResponse();
o.artifactGcsUri = artifactGcsUri;
o.createTime = createTime;
o.databaseType = databaseType;
o.encryptionConfig = encryptionConfig;
o.endpointUri = endpointUri;
o.hiveMetastoreConfig = hiveMetastoreConfig;
o.labels = labels;
o.maintenanceWindow = maintenanceWindow;
o.metadataManagementActivity = metadataManagementActivity;
o.name = name;
o.network = network;
o.networkConfig = networkConfig;
o.port = port;
o.releaseChannel = releaseChannel;
o.scalingConfig = scalingConfig;
o.state = state;
o.stateMessage = stateMessage;
o.telemetryConfig = telemetryConfig;
o.tier = tier;
o.uid = uid;
o.updateTime = updateTime;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy