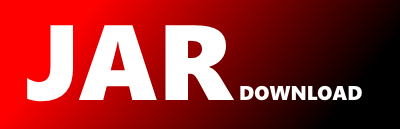
com.pulumi.googlenative.networkservices.v1.TcpRouteArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.networkservices.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.networkservices.v1.inputs.TcpRouteRouteRuleArgs;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TcpRouteArgs extends com.pulumi.resources.ResourceArgs {
public static final TcpRouteArgs Empty = new TcpRouteArgs();
/**
* Optional. A free-text description of the resource. Max length 1024 characters.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return Optional. A free-text description of the resource. Max length 1024 characters.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy