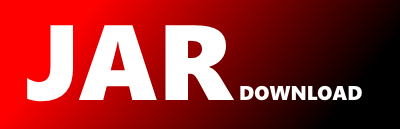
com.pulumi.googlenative.osconfig.v1alpha.inputs.OSPolicyResourceFileResourceArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.osconfig.v1alpha.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.osconfig.v1alpha.enums.OSPolicyResourceFileResourceState;
import com.pulumi.googlenative.osconfig.v1alpha.inputs.OSPolicyResourceFileArgs;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A resource that manages the state of a file.
*
*/
public final class OSPolicyResourceFileResourceArgs extends com.pulumi.resources.ResourceArgs {
public static final OSPolicyResourceFileResourceArgs Empty = new OSPolicyResourceFileResourceArgs();
/**
* A a file with this content. The size of the content is limited to 32KiB.
*
*/
@Import(name="content")
private @Nullable Output content;
/**
* @return A a file with this content. The size of the content is limited to 32KiB.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy