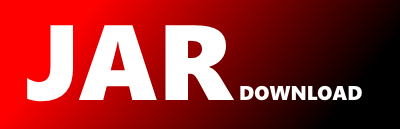
com.pulumi.googlenative.osconfig.v1beta.inputs.AssignmentArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.osconfig.v1beta.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.osconfig.v1beta.inputs.AssignmentGroupLabelArgs;
import com.pulumi.googlenative.osconfig.v1beta.inputs.AssignmentOsTypeArgs;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* An assignment represents the group or groups of VM instances that the policy applies to. If an assignment is empty, it applies to all VM instances. Otherwise, the targeted VM instances must meet all the criteria specified. So if both labels and zones are specified, the policy applies to VM instances with those labels and in those zones.
*
*/
public final class AssignmentArgs extends com.pulumi.resources.ResourceArgs {
public static final AssignmentArgs Empty = new AssignmentArgs();
/**
* Targets instances matching at least one of these label sets. This allows an assignment to target disparate groups, for example "env=prod or env=staging".
*
*/
@Import(name="groupLabels")
private @Nullable Output> groupLabels;
/**
* @return Targets instances matching at least one of these label sets. This allows an assignment to target disparate groups, for example "env=prod or env=staging".
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy