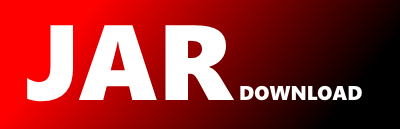
com.pulumi.googlenative.redis.v1.inputs.TimeOfDayArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.redis.v1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Represents a time of day. The date and time zone are either not significant or are specified elsewhere. An API may choose to allow leap seconds. Related types are google.type.Date and `google.protobuf.Timestamp`.
*
*/
public final class TimeOfDayArgs extends com.pulumi.resources.ResourceArgs {
public static final TimeOfDayArgs Empty = new TimeOfDayArgs();
/**
* Hours of day in 24 hour format. Should be from 0 to 23. An API may choose to allow the value "24:00:00" for scenarios like business closing time.
*
*/
@Import(name="hours")
private @Nullable Output hours;
/**
* @return Hours of day in 24 hour format. Should be from 0 to 23. An API may choose to allow the value "24:00:00" for scenarios like business closing time.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy