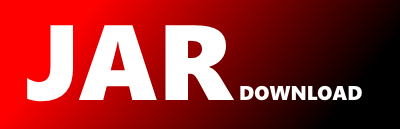
com.pulumi.googlenative.spanner.v1.outputs.GetBackupResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.spanner.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.spanner.v1.outputs.EncryptionInfoResponse;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetBackupResult {
/**
* @return The time the CreateBackup request is received. If the request does not specify `version_time`, the `version_time` of the backup will be equivalent to the `create_time`.
*
*/
private String createTime;
/**
* @return Required for the CreateBackup operation. Name of the database from which this backup was created. This needs to be in the same instance as the backup. Values are of the form `projects//instances//databases/`.
*
*/
private String database;
/**
* @return The database dialect information for the backup.
*
*/
private String databaseDialect;
/**
* @return The encryption information for the backup.
*
*/
private EncryptionInfoResponse encryptionInfo;
/**
* @return Required for the CreateBackup operation. The expiration time of the backup, with microseconds granularity that must be at least 6 hours and at most 366 days from the time the CreateBackup request is processed. Once the `expire_time` has passed, the backup is eligible to be automatically deleted by Cloud Spanner to free the resources used by the backup.
*
*/
private String expireTime;
/**
* @return The max allowed expiration time of the backup, with microseconds granularity. A backup's expiration time can be configured in multiple APIs: CreateBackup, UpdateBackup, CopyBackup. When updating or copying an existing backup, the expiration time specified must be less than `Backup.max_expire_time`.
*
*/
private String maxExpireTime;
/**
* @return Output only for the CreateBackup operation. Required for the UpdateBackup operation. A globally unique identifier for the backup which cannot be changed. Values are of the form `projects//instances//backups/a-z*[a-z0-9]` The final segment of the name must be between 2 and 60 characters in length. The backup is stored in the location(s) specified in the instance configuration of the instance containing the backup, identified by the prefix of the backup name of the form `projects//instances/`.
*
*/
private String name;
/**
* @return The names of the destination backups being created by copying this source backup. The backup names are of the form `projects//instances//backups/`. Referencing backups may exist in different instances. The existence of any referencing backup prevents the backup from being deleted. When the copy operation is done (either successfully completed or cancelled or the destination backup is deleted), the reference to the backup is removed.
*
*/
private List referencingBackups;
/**
* @return The names of the restored databases that reference the backup. The database names are of the form `projects//instances//databases/`. Referencing databases may exist in different instances. The existence of any referencing database prevents the backup from being deleted. When a restored database from the backup enters the `READY` state, the reference to the backup is removed.
*
*/
private List referencingDatabases;
/**
* @return Size of the backup in bytes.
*
*/
private String sizeBytes;
/**
* @return The current state of the backup.
*
*/
private String state;
/**
* @return The backup will contain an externally consistent copy of the database at the timestamp specified by `version_time`. If `version_time` is not specified, the system will set `version_time` to the `create_time` of the backup.
*
*/
private String versionTime;
private GetBackupResult() {}
/**
* @return The time the CreateBackup request is received. If the request does not specify `version_time`, the `version_time` of the backup will be equivalent to the `create_time`.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return Required for the CreateBackup operation. Name of the database from which this backup was created. This needs to be in the same instance as the backup. Values are of the form `projects//instances//databases/`.
*
*/
public String database() {
return this.database;
}
/**
* @return The database dialect information for the backup.
*
*/
public String databaseDialect() {
return this.databaseDialect;
}
/**
* @return The encryption information for the backup.
*
*/
public EncryptionInfoResponse encryptionInfo() {
return this.encryptionInfo;
}
/**
* @return Required for the CreateBackup operation. The expiration time of the backup, with microseconds granularity that must be at least 6 hours and at most 366 days from the time the CreateBackup request is processed. Once the `expire_time` has passed, the backup is eligible to be automatically deleted by Cloud Spanner to free the resources used by the backup.
*
*/
public String expireTime() {
return this.expireTime;
}
/**
* @return The max allowed expiration time of the backup, with microseconds granularity. A backup's expiration time can be configured in multiple APIs: CreateBackup, UpdateBackup, CopyBackup. When updating or copying an existing backup, the expiration time specified must be less than `Backup.max_expire_time`.
*
*/
public String maxExpireTime() {
return this.maxExpireTime;
}
/**
* @return Output only for the CreateBackup operation. Required for the UpdateBackup operation. A globally unique identifier for the backup which cannot be changed. Values are of the form `projects//instances//backups/a-z*[a-z0-9]` The final segment of the name must be between 2 and 60 characters in length. The backup is stored in the location(s) specified in the instance configuration of the instance containing the backup, identified by the prefix of the backup name of the form `projects//instances/`.
*
*/
public String name() {
return this.name;
}
/**
* @return The names of the destination backups being created by copying this source backup. The backup names are of the form `projects//instances//backups/`. Referencing backups may exist in different instances. The existence of any referencing backup prevents the backup from being deleted. When the copy operation is done (either successfully completed or cancelled or the destination backup is deleted), the reference to the backup is removed.
*
*/
public List referencingBackups() {
return this.referencingBackups;
}
/**
* @return The names of the restored databases that reference the backup. The database names are of the form `projects//instances//databases/`. Referencing databases may exist in different instances. The existence of any referencing database prevents the backup from being deleted. When a restored database from the backup enters the `READY` state, the reference to the backup is removed.
*
*/
public List referencingDatabases() {
return this.referencingDatabases;
}
/**
* @return Size of the backup in bytes.
*
*/
public String sizeBytes() {
return this.sizeBytes;
}
/**
* @return The current state of the backup.
*
*/
public String state() {
return this.state;
}
/**
* @return The backup will contain an externally consistent copy of the database at the timestamp specified by `version_time`. If `version_time` is not specified, the system will set `version_time` to the `create_time` of the backup.
*
*/
public String versionTime() {
return this.versionTime;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBackupResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String createTime;
private String database;
private String databaseDialect;
private EncryptionInfoResponse encryptionInfo;
private String expireTime;
private String maxExpireTime;
private String name;
private List referencingBackups;
private List referencingDatabases;
private String sizeBytes;
private String state;
private String versionTime;
public Builder() {}
public Builder(GetBackupResult defaults) {
Objects.requireNonNull(defaults);
this.createTime = defaults.createTime;
this.database = defaults.database;
this.databaseDialect = defaults.databaseDialect;
this.encryptionInfo = defaults.encryptionInfo;
this.expireTime = defaults.expireTime;
this.maxExpireTime = defaults.maxExpireTime;
this.name = defaults.name;
this.referencingBackups = defaults.referencingBackups;
this.referencingDatabases = defaults.referencingDatabases;
this.sizeBytes = defaults.sizeBytes;
this.state = defaults.state;
this.versionTime = defaults.versionTime;
}
@CustomType.Setter
public Builder createTime(String createTime) {
this.createTime = Objects.requireNonNull(createTime);
return this;
}
@CustomType.Setter
public Builder database(String database) {
this.database = Objects.requireNonNull(database);
return this;
}
@CustomType.Setter
public Builder databaseDialect(String databaseDialect) {
this.databaseDialect = Objects.requireNonNull(databaseDialect);
return this;
}
@CustomType.Setter
public Builder encryptionInfo(EncryptionInfoResponse encryptionInfo) {
this.encryptionInfo = Objects.requireNonNull(encryptionInfo);
return this;
}
@CustomType.Setter
public Builder expireTime(String expireTime) {
this.expireTime = Objects.requireNonNull(expireTime);
return this;
}
@CustomType.Setter
public Builder maxExpireTime(String maxExpireTime) {
this.maxExpireTime = Objects.requireNonNull(maxExpireTime);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder referencingBackups(List referencingBackups) {
this.referencingBackups = Objects.requireNonNull(referencingBackups);
return this;
}
public Builder referencingBackups(String... referencingBackups) {
return referencingBackups(List.of(referencingBackups));
}
@CustomType.Setter
public Builder referencingDatabases(List referencingDatabases) {
this.referencingDatabases = Objects.requireNonNull(referencingDatabases);
return this;
}
public Builder referencingDatabases(String... referencingDatabases) {
return referencingDatabases(List.of(referencingDatabases));
}
@CustomType.Setter
public Builder sizeBytes(String sizeBytes) {
this.sizeBytes = Objects.requireNonNull(sizeBytes);
return this;
}
@CustomType.Setter
public Builder state(String state) {
this.state = Objects.requireNonNull(state);
return this;
}
@CustomType.Setter
public Builder versionTime(String versionTime) {
this.versionTime = Objects.requireNonNull(versionTime);
return this;
}
public GetBackupResult build() {
final var o = new GetBackupResult();
o.createTime = createTime;
o.database = database;
o.databaseDialect = databaseDialect;
o.encryptionInfo = encryptionInfo;
o.expireTime = expireTime;
o.maxExpireTime = maxExpireTime;
o.name = name;
o.referencingBackups = referencingBackups;
o.referencingDatabases = referencingDatabases;
o.sizeBytes = sizeBytes;
o.state = state;
o.versionTime = versionTime;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy