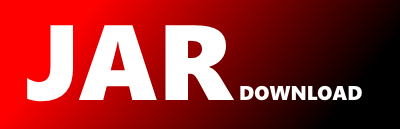
com.pulumi.googlenative.speech.v1.CustomClassArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.speech.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.speech.v1.inputs.ClassItemArgs;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CustomClassArgs extends com.pulumi.resources.ResourceArgs {
public static final CustomClassArgs Empty = new CustomClassArgs();
/**
* The ID to use for the custom class, which will become the final component of the custom class' resource name. This value should restrict to letters, numbers, and hyphens, with the first character a letter, the last a letter or a number, and be 4-63 characters.
*
*/
@Import(name="customClassId", required=true)
private Output customClassId;
/**
* @return The ID to use for the custom class, which will become the final component of the custom class' resource name. This value should restrict to letters, numbers, and hyphens, with the first character a letter, the last a letter or a number, and be 4-63 characters.
*
*/
public Output customClassId() {
return this.customClassId;
}
/**
* A collection of class items.
*
*/
@Import(name="items")
private @Nullable Output> items;
/**
* @return A collection of class items.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy