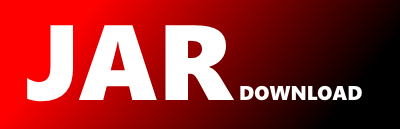
com.pulumi.googlenative.sqladmin.v1beta4.InstanceArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.sqladmin.v1beta4;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.sqladmin.v1beta4.enums.InstanceBackendType;
import com.pulumi.googlenative.sqladmin.v1beta4.enums.InstanceDatabaseVersion;
import com.pulumi.googlenative.sqladmin.v1beta4.enums.InstanceInstanceType;
import com.pulumi.googlenative.sqladmin.v1beta4.enums.InstanceState;
import com.pulumi.googlenative.sqladmin.v1beta4.enums.InstanceSuspensionReasonItem;
import com.pulumi.googlenative.sqladmin.v1beta4.inputs.DiskEncryptionConfigurationArgs;
import com.pulumi.googlenative.sqladmin.v1beta4.inputs.DiskEncryptionStatusArgs;
import com.pulumi.googlenative.sqladmin.v1beta4.inputs.InstanceFailoverReplicaArgs;
import com.pulumi.googlenative.sqladmin.v1beta4.inputs.IpMappingArgs;
import com.pulumi.googlenative.sqladmin.v1beta4.inputs.OnPremisesConfigurationArgs;
import com.pulumi.googlenative.sqladmin.v1beta4.inputs.ReplicaConfigurationArgs;
import com.pulumi.googlenative.sqladmin.v1beta4.inputs.SettingsArgs;
import com.pulumi.googlenative.sqladmin.v1beta4.inputs.SqlOutOfDiskReportArgs;
import com.pulumi.googlenative.sqladmin.v1beta4.inputs.SqlScheduledMaintenanceArgs;
import com.pulumi.googlenative.sqladmin.v1beta4.inputs.SslCertArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class InstanceArgs extends com.pulumi.resources.ResourceArgs {
public static final InstanceArgs Empty = new InstanceArgs();
/**
* The backend type. `SECOND_GEN`: Cloud SQL database instance. `EXTERNAL`: A database server that is not managed by Google. This property is read-only; use the `tier` property in the `settings` object to determine the database type.
*
*/
@Import(name="backendType")
private @Nullable Output backendType;
/**
* @return The backend type. `SECOND_GEN`: Cloud SQL database instance. `EXTERNAL`: A database server that is not managed by Google. This property is read-only; use the `tier` property in the `settings` object to determine the database type.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy