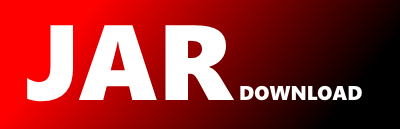
com.pulumi.googlenative.storage.v1.DefaultObjectAccessControl Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.storage.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.googlenative.Utilities;
import com.pulumi.googlenative.storage.v1.DefaultObjectAccessControlArgs;
import com.pulumi.googlenative.storage.v1.outputs.DefaultObjectAccessControlProjectTeamResponse;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Creates a new default object ACL entry on the specified bucket.
* Auto-naming is currently not supported for this resource.
*
*/
@ResourceType(type="google-native:storage/v1:DefaultObjectAccessControl")
public class DefaultObjectAccessControl extends com.pulumi.resources.CustomResource {
@Export(name="bucket", type=String.class, parameters={})
private Output bucket;
public Output bucket() {
return this.bucket;
}
/**
* The domain associated with the entity, if any.
*
*/
@Export(name="domain", type=String.class, parameters={})
private Output domain;
/**
* @return The domain associated with the entity, if any.
*
*/
public Output domain() {
return this.domain;
}
/**
* The email address associated with the entity, if any.
*
*/
@Export(name="email", type=String.class, parameters={})
private Output email;
/**
* @return The email address associated with the entity, if any.
*
*/
public Output email() {
return this.email;
}
/**
* The entity holding the permission, in one of the following forms:
* - user-userId
* - user-email
* - group-groupId
* - group-email
* - domain-domain
* - project-team-projectId
* - allUsers
* - allAuthenticatedUsers Examples:
* - The user [email protected] would be [email protected].
* - The group [email protected] would be [email protected].
* - To refer to all members of the Google Apps for Business domain example.com, the entity would be domain-example.com.
*
*/
@Export(name="entity", type=String.class, parameters={})
private Output entity;
/**
* @return The entity holding the permission, in one of the following forms:
* - user-userId
* - user-email
* - group-groupId
* - group-email
* - domain-domain
* - project-team-projectId
* - allUsers
* - allAuthenticatedUsers Examples:
* - The user [email protected] would be [email protected].
* - The group [email protected] would be [email protected].
* - To refer to all members of the Google Apps for Business domain example.com, the entity would be domain-example.com.
*
*/
public Output entity() {
return this.entity;
}
/**
* The ID for the entity, if any.
*
*/
@Export(name="entityId", type=String.class, parameters={})
private Output entityId;
/**
* @return The ID for the entity, if any.
*
*/
public Output entityId() {
return this.entityId;
}
/**
* HTTP 1.1 Entity tag for the access-control entry.
*
*/
@Export(name="etag", type=String.class, parameters={})
private Output etag;
/**
* @return HTTP 1.1 Entity tag for the access-control entry.
*
*/
public Output etag() {
return this.etag;
}
/**
* The content generation of the object, if applied to an object.
*
*/
@Export(name="generation", type=String.class, parameters={})
private Output generation;
/**
* @return The content generation of the object, if applied to an object.
*
*/
public Output generation() {
return this.generation;
}
/**
* The kind of item this is. For object access control entries, this is always storage#objectAccessControl.
*
*/
@Export(name="kind", type=String.class, parameters={})
private Output kind;
/**
* @return The kind of item this is. For object access control entries, this is always storage#objectAccessControl.
*
*/
public Output kind() {
return this.kind;
}
/**
* The name of the object, if applied to an object.
*
*/
@Export(name="object", type=String.class, parameters={})
private Output object;
/**
* @return The name of the object, if applied to an object.
*
*/
public Output object() {
return this.object;
}
/**
* The project team associated with the entity, if any.
*
*/
@Export(name="projectTeam", type=DefaultObjectAccessControlProjectTeamResponse.class, parameters={})
private Output projectTeam;
/**
* @return The project team associated with the entity, if any.
*
*/
public Output projectTeam() {
return this.projectTeam;
}
/**
* The access permission for the entity.
*
*/
@Export(name="role", type=String.class, parameters={})
private Output role;
/**
* @return The access permission for the entity.
*
*/
public Output role() {
return this.role;
}
/**
* The link to this access-control entry.
*
*/
@Export(name="selfLink", type=String.class, parameters={})
private Output selfLink;
/**
* @return The link to this access-control entry.
*
*/
public Output selfLink() {
return this.selfLink;
}
/**
* The project to be billed for this request. Required for Requester Pays buckets.
*
*/
@Export(name="userProject", type=String.class, parameters={})
private Output* @Nullable */ String> userProject;
/**
* @return The project to be billed for this request. Required for Requester Pays buckets.
*
*/
public Output> userProject() {
return Codegen.optional(this.userProject);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public DefaultObjectAccessControl(String name) {
this(name, DefaultObjectAccessControlArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public DefaultObjectAccessControl(String name, DefaultObjectAccessControlArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public DefaultObjectAccessControl(String name, DefaultObjectAccessControlArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:storage/v1:DefaultObjectAccessControl", name, args == null ? DefaultObjectAccessControlArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private DefaultObjectAccessControl(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:storage/v1:DefaultObjectAccessControl", name, null, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static DefaultObjectAccessControl get(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new DefaultObjectAccessControl(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy