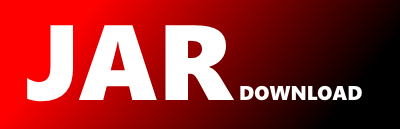
com.pulumi.googlenative.storage.v1.inputs.BucketAccessControlArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.storage.v1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.storage.v1.inputs.BucketAccessControlProjectTeamArgs;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* An access-control entry.
*
*/
public final class BucketAccessControlArgs extends com.pulumi.resources.ResourceArgs {
public static final BucketAccessControlArgs Empty = new BucketAccessControlArgs();
/**
* The name of the bucket.
*
*/
@Import(name="bucket")
private @Nullable Output bucket;
/**
* @return The name of the bucket.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy