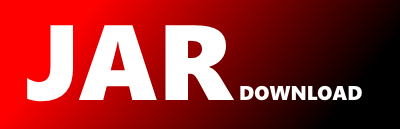
com.pulumi.googlenative.storage.v1.outputs.GetBucketIamPolicyResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.storage.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.storage.v1.outputs.BucketIamPolicyBindingsItemResponse;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetBucketIamPolicyResult {
/**
* @return An association between a role, which comes with a set of permissions, and members who may assume that role.
*
*/
private List bindings;
/**
* @return HTTP 1.1 Entity tag for the policy.
*
*/
private String etag;
/**
* @return The kind of item this is. For policies, this is always storage#policy. This field is ignored on input.
*
*/
private String kind;
/**
* @return The ID of the resource to which this policy belongs. Will be of the form projects/_/buckets/bucket for buckets, and projects/_/buckets/bucket/objects/object for objects. A specific generation may be specified by appending #generationNumber to the end of the object name, e.g. projects/_/buckets/my-bucket/objects/data.txt#17. The current generation can be denoted with #0. This field is ignored on input.
*
*/
private String resourceId;
/**
* @return The IAM policy format version.
*
*/
private Integer version;
private GetBucketIamPolicyResult() {}
/**
* @return An association between a role, which comes with a set of permissions, and members who may assume that role.
*
*/
public List bindings() {
return this.bindings;
}
/**
* @return HTTP 1.1 Entity tag for the policy.
*
*/
public String etag() {
return this.etag;
}
/**
* @return The kind of item this is. For policies, this is always storage#policy. This field is ignored on input.
*
*/
public String kind() {
return this.kind;
}
/**
* @return The ID of the resource to which this policy belongs. Will be of the form projects/_/buckets/bucket for buckets, and projects/_/buckets/bucket/objects/object for objects. A specific generation may be specified by appending #generationNumber to the end of the object name, e.g. projects/_/buckets/my-bucket/objects/data.txt#17. The current generation can be denoted with #0. This field is ignored on input.
*
*/
public String resourceId() {
return this.resourceId;
}
/**
* @return The IAM policy format version.
*
*/
public Integer version() {
return this.version;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBucketIamPolicyResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List bindings;
private String etag;
private String kind;
private String resourceId;
private Integer version;
public Builder() {}
public Builder(GetBucketIamPolicyResult defaults) {
Objects.requireNonNull(defaults);
this.bindings = defaults.bindings;
this.etag = defaults.etag;
this.kind = defaults.kind;
this.resourceId = defaults.resourceId;
this.version = defaults.version;
}
@CustomType.Setter
public Builder bindings(List bindings) {
this.bindings = Objects.requireNonNull(bindings);
return this;
}
public Builder bindings(BucketIamPolicyBindingsItemResponse... bindings) {
return bindings(List.of(bindings));
}
@CustomType.Setter
public Builder etag(String etag) {
this.etag = Objects.requireNonNull(etag);
return this;
}
@CustomType.Setter
public Builder kind(String kind) {
this.kind = Objects.requireNonNull(kind);
return this;
}
@CustomType.Setter
public Builder resourceId(String resourceId) {
this.resourceId = Objects.requireNonNull(resourceId);
return this;
}
@CustomType.Setter
public Builder version(Integer version) {
this.version = Objects.requireNonNull(version);
return this;
}
public GetBucketIamPolicyResult build() {
final var o = new GetBucketIamPolicyResult();
o.bindings = bindings;
o.etag = etag;
o.kind = kind;
o.resourceId = resourceId;
o.version = version;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy