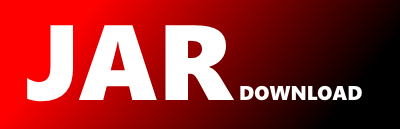
com.pulumi.googlenative.testing.v1.inputs.IosTestSetupArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.testing.v1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.testing.v1.inputs.FileReferenceArgs;
import com.pulumi.googlenative.testing.v1.inputs.IosDeviceFileArgs;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A description of how to set up an iOS device prior to running the test.
*
*/
public final class IosTestSetupArgs extends com.pulumi.resources.ResourceArgs {
public static final IosTestSetupArgs Empty = new IosTestSetupArgs();
/**
* iOS apps to install in addition to those being directly tested.
*
*/
@Import(name="additionalIpas")
private @Nullable Output> additionalIpas;
/**
* @return iOS apps to install in addition to those being directly tested.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy