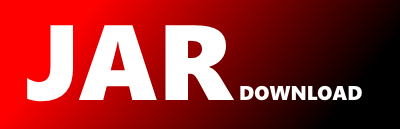
com.pulumi.googlenative.vmmigration.v1.CloneJob Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.vmmigration.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.googlenative.Utilities;
import com.pulumi.googlenative.vmmigration.v1.CloneJobArgs;
import com.pulumi.googlenative.vmmigration.v1.outputs.CloneStepResponse;
import com.pulumi.googlenative.vmmigration.v1.outputs.ComputeEngineTargetDetailsResponse;
import com.pulumi.googlenative.vmmigration.v1.outputs.StatusResponse;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Initiates a Clone of a specific migrating VM.
* Auto-naming is currently not supported for this resource.
* Note - this resource's API doesn't support deletion. When deleted, the resource will persist
* on Google Cloud even though it will be deleted from Pulumi state.
*
*/
@ResourceType(type="google-native:vmmigration/v1:CloneJob")
public class CloneJob extends com.pulumi.resources.CustomResource {
/**
* Required. The clone job identifier.
*
*/
@Export(name="cloneJobId", type=String.class, parameters={})
private Output cloneJobId;
/**
* @return Required. The clone job identifier.
*
*/
public Output cloneJobId() {
return this.cloneJobId;
}
/**
* Details of the target VM in Compute Engine.
*
*/
@Export(name="computeEngineTargetDetails", type=ComputeEngineTargetDetailsResponse.class, parameters={})
private Output computeEngineTargetDetails;
/**
* @return Details of the target VM in Compute Engine.
*
*/
public Output computeEngineTargetDetails() {
return this.computeEngineTargetDetails;
}
/**
* The time the clone job was created (as an API call, not when it was actually created in the target).
*
*/
@Export(name="createTime", type=String.class, parameters={})
private Output createTime;
/**
* @return The time the clone job was created (as an API call, not when it was actually created in the target).
*
*/
public Output createTime() {
return this.createTime;
}
/**
* The time the clone job was ended.
*
*/
@Export(name="endTime", type=String.class, parameters={})
private Output endTime;
/**
* @return The time the clone job was ended.
*
*/
public Output endTime() {
return this.endTime;
}
/**
* Provides details for the errors that led to the Clone Job's state.
*
*/
@Export(name="error", type=StatusResponse.class, parameters={})
private Output error;
/**
* @return Provides details for the errors that led to the Clone Job's state.
*
*/
public Output error() {
return this.error;
}
@Export(name="location", type=String.class, parameters={})
private Output location;
public Output location() {
return this.location;
}
@Export(name="migratingVmId", type=String.class, parameters={})
private Output migratingVmId;
public Output migratingVmId() {
return this.migratingVmId;
}
/**
* The name of the clone.
*
*/
@Export(name="name", type=String.class, parameters={})
private Output name;
/**
* @return The name of the clone.
*
*/
public Output name() {
return this.name;
}
@Export(name="project", type=String.class, parameters={})
private Output project;
public Output project() {
return this.project;
}
/**
* A request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. The server will guarantee that for at least 60 minutes since the first request. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*
*/
@Export(name="requestId", type=String.class, parameters={})
private Output* @Nullable */ String> requestId;
/**
* @return A request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. The server will guarantee that for at least 60 minutes since the first request. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*
*/
public Output> requestId() {
return Codegen.optional(this.requestId);
}
@Export(name="sourceId", type=String.class, parameters={})
private Output sourceId;
public Output sourceId() {
return this.sourceId;
}
/**
* State of the clone job.
*
*/
@Export(name="state", type=String.class, parameters={})
private Output state;
/**
* @return State of the clone job.
*
*/
public Output state() {
return this.state;
}
/**
* The time the state was last updated.
*
*/
@Export(name="stateTime", type=String.class, parameters={})
private Output stateTime;
/**
* @return The time the state was last updated.
*
*/
public Output stateTime() {
return this.stateTime;
}
/**
* The clone steps list representing its progress.
*
*/
@Export(name="steps", type=List.class, parameters={CloneStepResponse.class})
private Output> steps;
/**
* @return The clone steps list representing its progress.
*
*/
public Output> steps() {
return this.steps;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public CloneJob(String name) {
this(name, CloneJobArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public CloneJob(String name, CloneJobArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public CloneJob(String name, CloneJobArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:vmmigration/v1:CloneJob", name, args == null ? CloneJobArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private CloneJob(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("google-native:vmmigration/v1:CloneJob", name, null, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static CloneJob get(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new CloneJob(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy