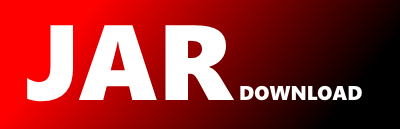
com.pulumi.googlenative.vmmigration.v1.CutoverJobArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.vmmigration.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CutoverJobArgs extends com.pulumi.resources.ResourceArgs {
public static final CutoverJobArgs Empty = new CutoverJobArgs();
/**
* Required. The cutover job identifier.
*
*/
@Import(name="cutoverJobId", required=true)
private Output cutoverJobId;
/**
* @return Required. The cutover job identifier.
*
*/
public Output cutoverJobId() {
return this.cutoverJobId;
}
@Import(name="location")
private @Nullable Output location;
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy