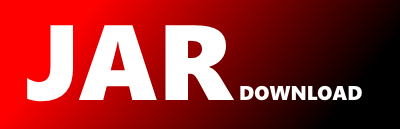
com.pulumi.googlenative.vmmigration.v1.inputs.ComputeEngineTargetDefaultsArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.vmmigration.v1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.vmmigration.v1.enums.ComputeEngineTargetDefaultsDiskType;
import com.pulumi.googlenative.vmmigration.v1.enums.ComputeEngineTargetDefaultsLicenseType;
import com.pulumi.googlenative.vmmigration.v1.inputs.ComputeSchedulingArgs;
import com.pulumi.googlenative.vmmigration.v1.inputs.NetworkInterfaceArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ComputeEngineTargetDefaults is a collection of details for creating a VM in a target Compute Engine project.
*
*/
public final class ComputeEngineTargetDefaultsArgs extends com.pulumi.resources.ResourceArgs {
public static final ComputeEngineTargetDefaultsArgs Empty = new ComputeEngineTargetDefaultsArgs();
/**
* Additional licenses to assign to the VM.
*
*/
@Import(name="additionalLicenses")
private @Nullable Output> additionalLicenses;
/**
* @return Additional licenses to assign to the VM.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy