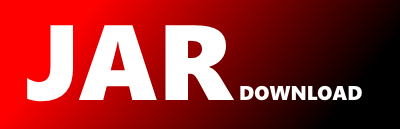
com.pulumi.googlenative.vmmigration.v1.outputs.AwsSourceDetailsResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.vmmigration.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.vmmigration.v1.outputs.AccessKeyCredentialsResponse;
import com.pulumi.googlenative.vmmigration.v1.outputs.StatusResponse;
import com.pulumi.googlenative.vmmigration.v1.outputs.TagResponse;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class AwsSourceDetailsResponse {
/**
* @return AWS Credentials using access key id and secret.
*
*/
private AccessKeyCredentialsResponse accessKeyCreds;
/**
* @return Immutable. The AWS region that the source VMs will be migrated from.
*
*/
private String awsRegion;
/**
* @return Provides details on the state of the Source in case of an error.
*
*/
private StatusResponse error;
/**
* @return AWS security group names to limit the scope of the source inventory.
*
*/
private List inventorySecurityGroupNames;
/**
* @return AWS resource tags to limit the scope of the source inventory.
*
*/
private List inventoryTagList;
/**
* @return User specified tags to add to every M2VM generated resource in AWS. These tags will be set in addition to the default tags that are set as part of the migration process. The tags must not begin with the reserved prefix `m2vm`.
*
*/
private Map migrationResourcesUserTags;
/**
* @return The source's public IP. All communication initiated by this source will originate from this IP.
*
*/
private String publicIp;
/**
* @return State of the source as determined by the health check.
*
*/
private String state;
private AwsSourceDetailsResponse() {}
/**
* @return AWS Credentials using access key id and secret.
*
*/
public AccessKeyCredentialsResponse accessKeyCreds() {
return this.accessKeyCreds;
}
/**
* @return Immutable. The AWS region that the source VMs will be migrated from.
*
*/
public String awsRegion() {
return this.awsRegion;
}
/**
* @return Provides details on the state of the Source in case of an error.
*
*/
public StatusResponse error() {
return this.error;
}
/**
* @return AWS security group names to limit the scope of the source inventory.
*
*/
public List inventorySecurityGroupNames() {
return this.inventorySecurityGroupNames;
}
/**
* @return AWS resource tags to limit the scope of the source inventory.
*
*/
public List inventoryTagList() {
return this.inventoryTagList;
}
/**
* @return User specified tags to add to every M2VM generated resource in AWS. These tags will be set in addition to the default tags that are set as part of the migration process. The tags must not begin with the reserved prefix `m2vm`.
*
*/
public Map migrationResourcesUserTags() {
return this.migrationResourcesUserTags;
}
/**
* @return The source's public IP. All communication initiated by this source will originate from this IP.
*
*/
public String publicIp() {
return this.publicIp;
}
/**
* @return State of the source as determined by the health check.
*
*/
public String state() {
return this.state;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AwsSourceDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private AccessKeyCredentialsResponse accessKeyCreds;
private String awsRegion;
private StatusResponse error;
private List inventorySecurityGroupNames;
private List inventoryTagList;
private Map migrationResourcesUserTags;
private String publicIp;
private String state;
public Builder() {}
public Builder(AwsSourceDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.accessKeyCreds = defaults.accessKeyCreds;
this.awsRegion = defaults.awsRegion;
this.error = defaults.error;
this.inventorySecurityGroupNames = defaults.inventorySecurityGroupNames;
this.inventoryTagList = defaults.inventoryTagList;
this.migrationResourcesUserTags = defaults.migrationResourcesUserTags;
this.publicIp = defaults.publicIp;
this.state = defaults.state;
}
@CustomType.Setter
public Builder accessKeyCreds(AccessKeyCredentialsResponse accessKeyCreds) {
this.accessKeyCreds = Objects.requireNonNull(accessKeyCreds);
return this;
}
@CustomType.Setter
public Builder awsRegion(String awsRegion) {
this.awsRegion = Objects.requireNonNull(awsRegion);
return this;
}
@CustomType.Setter
public Builder error(StatusResponse error) {
this.error = Objects.requireNonNull(error);
return this;
}
@CustomType.Setter
public Builder inventorySecurityGroupNames(List inventorySecurityGroupNames) {
this.inventorySecurityGroupNames = Objects.requireNonNull(inventorySecurityGroupNames);
return this;
}
public Builder inventorySecurityGroupNames(String... inventorySecurityGroupNames) {
return inventorySecurityGroupNames(List.of(inventorySecurityGroupNames));
}
@CustomType.Setter
public Builder inventoryTagList(List inventoryTagList) {
this.inventoryTagList = Objects.requireNonNull(inventoryTagList);
return this;
}
public Builder inventoryTagList(TagResponse... inventoryTagList) {
return inventoryTagList(List.of(inventoryTagList));
}
@CustomType.Setter
public Builder migrationResourcesUserTags(Map migrationResourcesUserTags) {
this.migrationResourcesUserTags = Objects.requireNonNull(migrationResourcesUserTags);
return this;
}
@CustomType.Setter
public Builder publicIp(String publicIp) {
this.publicIp = Objects.requireNonNull(publicIp);
return this;
}
@CustomType.Setter
public Builder state(String state) {
this.state = Objects.requireNonNull(state);
return this;
}
public AwsSourceDetailsResponse build() {
final var o = new AwsSourceDetailsResponse();
o.accessKeyCreds = accessKeyCreds;
o.awsRegion = awsRegion;
o.error = error;
o.inventorySecurityGroupNames = inventorySecurityGroupNames;
o.inventoryTagList = inventoryTagList;
o.migrationResourcesUserTags = migrationResourcesUserTags;
o.publicIp = publicIp;
o.state = state;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy