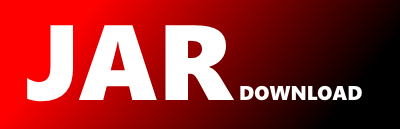
com.pulumi.googlenative.vmmigration.v1.outputs.GetCutoverJobResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.vmmigration.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.vmmigration.v1.outputs.ComputeEngineTargetDetailsResponse;
import com.pulumi.googlenative.vmmigration.v1.outputs.CutoverStepResponse;
import com.pulumi.googlenative.vmmigration.v1.outputs.StatusResponse;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetCutoverJobResult {
/**
* @return Details of the target VM in Compute Engine.
*
*/
private ComputeEngineTargetDetailsResponse computeEngineTargetDetails;
/**
* @return The time the cutover job was created (as an API call, not when it was actually created in the target).
*
*/
private String createTime;
/**
* @return The time the cutover job had finished.
*
*/
private String endTime;
/**
* @return Provides details for the errors that led to the Cutover Job's state.
*
*/
private StatusResponse error;
/**
* @return The name of the cutover job.
*
*/
private String name;
/**
* @return The current progress in percentage of the cutover job.
*
*/
private Integer progressPercent;
/**
* @return State of the cutover job.
*
*/
private String state;
/**
* @return A message providing possible extra details about the current state.
*
*/
private String stateMessage;
/**
* @return The time the state was last updated.
*
*/
private String stateTime;
/**
* @return The cutover steps list representing its progress.
*
*/
private List steps;
private GetCutoverJobResult() {}
/**
* @return Details of the target VM in Compute Engine.
*
*/
public ComputeEngineTargetDetailsResponse computeEngineTargetDetails() {
return this.computeEngineTargetDetails;
}
/**
* @return The time the cutover job was created (as an API call, not when it was actually created in the target).
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return The time the cutover job had finished.
*
*/
public String endTime() {
return this.endTime;
}
/**
* @return Provides details for the errors that led to the Cutover Job's state.
*
*/
public StatusResponse error() {
return this.error;
}
/**
* @return The name of the cutover job.
*
*/
public String name() {
return this.name;
}
/**
* @return The current progress in percentage of the cutover job.
*
*/
public Integer progressPercent() {
return this.progressPercent;
}
/**
* @return State of the cutover job.
*
*/
public String state() {
return this.state;
}
/**
* @return A message providing possible extra details about the current state.
*
*/
public String stateMessage() {
return this.stateMessage;
}
/**
* @return The time the state was last updated.
*
*/
public String stateTime() {
return this.stateTime;
}
/**
* @return The cutover steps list representing its progress.
*
*/
public List steps() {
return this.steps;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetCutoverJobResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private ComputeEngineTargetDetailsResponse computeEngineTargetDetails;
private String createTime;
private String endTime;
private StatusResponse error;
private String name;
private Integer progressPercent;
private String state;
private String stateMessage;
private String stateTime;
private List steps;
public Builder() {}
public Builder(GetCutoverJobResult defaults) {
Objects.requireNonNull(defaults);
this.computeEngineTargetDetails = defaults.computeEngineTargetDetails;
this.createTime = defaults.createTime;
this.endTime = defaults.endTime;
this.error = defaults.error;
this.name = defaults.name;
this.progressPercent = defaults.progressPercent;
this.state = defaults.state;
this.stateMessage = defaults.stateMessage;
this.stateTime = defaults.stateTime;
this.steps = defaults.steps;
}
@CustomType.Setter
public Builder computeEngineTargetDetails(ComputeEngineTargetDetailsResponse computeEngineTargetDetails) {
this.computeEngineTargetDetails = Objects.requireNonNull(computeEngineTargetDetails);
return this;
}
@CustomType.Setter
public Builder createTime(String createTime) {
this.createTime = Objects.requireNonNull(createTime);
return this;
}
@CustomType.Setter
public Builder endTime(String endTime) {
this.endTime = Objects.requireNonNull(endTime);
return this;
}
@CustomType.Setter
public Builder error(StatusResponse error) {
this.error = Objects.requireNonNull(error);
return this;
}
@CustomType.Setter
public Builder name(String name) {
this.name = Objects.requireNonNull(name);
return this;
}
@CustomType.Setter
public Builder progressPercent(Integer progressPercent) {
this.progressPercent = Objects.requireNonNull(progressPercent);
return this;
}
@CustomType.Setter
public Builder state(String state) {
this.state = Objects.requireNonNull(state);
return this;
}
@CustomType.Setter
public Builder stateMessage(String stateMessage) {
this.stateMessage = Objects.requireNonNull(stateMessage);
return this;
}
@CustomType.Setter
public Builder stateTime(String stateTime) {
this.stateTime = Objects.requireNonNull(stateTime);
return this;
}
@CustomType.Setter
public Builder steps(List steps) {
this.steps = Objects.requireNonNull(steps);
return this;
}
public Builder steps(CutoverStepResponse... steps) {
return steps(List.of(steps));
}
public GetCutoverJobResult build() {
final var o = new GetCutoverJobResult();
o.computeEngineTargetDetails = computeEngineTargetDetails;
o.createTime = createTime;
o.endTime = endTime;
o.error = error;
o.name = name;
o.progressPercent = progressPercent;
o.state = state;
o.stateMessage = stateMessage;
o.stateTime = stateTime;
o.steps = steps;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy