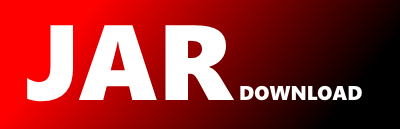
com.pulumi.googlenative.vmmigration.v1alpha1.inputs.AwsSourceDetailsArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.vmmigration.v1alpha1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.vmmigration.v1alpha1.inputs.AccessKeyCredentialsArgs;
import com.pulumi.googlenative.vmmigration.v1alpha1.inputs.TagArgs;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* AwsSourceDetails message describes a specific source details for the AWS source type.
*
*/
public final class AwsSourceDetailsArgs extends com.pulumi.resources.ResourceArgs {
public static final AwsSourceDetailsArgs Empty = new AwsSourceDetailsArgs();
/**
* AWS Credentials using access key id and secret.
*
*/
@Import(name="accessKeyCreds")
private @Nullable Output accessKeyCreds;
/**
* @return AWS Credentials using access key id and secret.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy