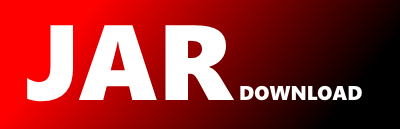
com.pulumi.googlenative.vmmigration.v1alpha1.outputs.ComputeEngineTargetDefaultsResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.vmmigration.v1alpha1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.googlenative.vmmigration.v1alpha1.outputs.AppliedLicenseResponse;
import com.pulumi.googlenative.vmmigration.v1alpha1.outputs.ComputeSchedulingResponse;
import com.pulumi.googlenative.vmmigration.v1alpha1.outputs.NetworkInterfaceResponse;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class ComputeEngineTargetDefaultsResponse {
/**
* @return Additional licenses to assign to the VM.
*
*/
private List additionalLicenses;
/**
* @return The OS license returned from the adaptation module report.
*
*/
private AppliedLicenseResponse appliedLicense;
/**
* @return The VM Boot Option, as set in the source vm.
*
*/
private String bootOption;
/**
* @return Compute instance scheduling information (if empty default is used).
*
*/
private ComputeSchedulingResponse computeScheduling;
/**
* @return The disk type to use in the VM.
*
*/
private String diskType;
/**
* @return The hostname to assign to the VM.
*
*/
private String hostname;
/**
* @return A map of labels to associate with the VM.
*
*/
private Map labels;
/**
* @return The license type to use in OS adaptation.
*
*/
private String licenseType;
/**
* @return The machine type to create the VM with.
*
*/
private String machineType;
/**
* @return The machine type series to create the VM with.
*
*/
private String machineTypeSeries;
/**
* @return The metadata key/value pairs to assign to the VM.
*
*/
private Map metadata;
/**
* @return List of NICs connected to this VM.
*
*/
private List networkInterfaces;
/**
* @return A map of network tags to associate with the VM.
*
*/
private List networkTags;
/**
* @return Defines whether the instance has Secure Boot enabled. This can be set to true only if the vm boot option is EFI.
*
*/
private Boolean secureBoot;
/**
* @return The service account to associate the VM with.
*
*/
private String serviceAccount;
/**
* @return The full path of the resource of type TargetProject which represents the Compute Engine project in which to create this VM.
*
*/
private String targetProject;
/**
* @return The name of the VM to create.
*
*/
private String vmName;
/**
* @return The zone in which to create the VM.
*
*/
private String zone;
private ComputeEngineTargetDefaultsResponse() {}
/**
* @return Additional licenses to assign to the VM.
*
*/
public List additionalLicenses() {
return this.additionalLicenses;
}
/**
* @return The OS license returned from the adaptation module report.
*
*/
public AppliedLicenseResponse appliedLicense() {
return this.appliedLicense;
}
/**
* @return The VM Boot Option, as set in the source vm.
*
*/
public String bootOption() {
return this.bootOption;
}
/**
* @return Compute instance scheduling information (if empty default is used).
*
*/
public ComputeSchedulingResponse computeScheduling() {
return this.computeScheduling;
}
/**
* @return The disk type to use in the VM.
*
*/
public String diskType() {
return this.diskType;
}
/**
* @return The hostname to assign to the VM.
*
*/
public String hostname() {
return this.hostname;
}
/**
* @return A map of labels to associate with the VM.
*
*/
public Map labels() {
return this.labels;
}
/**
* @return The license type to use in OS adaptation.
*
*/
public String licenseType() {
return this.licenseType;
}
/**
* @return The machine type to create the VM with.
*
*/
public String machineType() {
return this.machineType;
}
/**
* @return The machine type series to create the VM with.
*
*/
public String machineTypeSeries() {
return this.machineTypeSeries;
}
/**
* @return The metadata key/value pairs to assign to the VM.
*
*/
public Map metadata() {
return this.metadata;
}
/**
* @return List of NICs connected to this VM.
*
*/
public List networkInterfaces() {
return this.networkInterfaces;
}
/**
* @return A map of network tags to associate with the VM.
*
*/
public List networkTags() {
return this.networkTags;
}
/**
* @return Defines whether the instance has Secure Boot enabled. This can be set to true only if the vm boot option is EFI.
*
*/
public Boolean secureBoot() {
return this.secureBoot;
}
/**
* @return The service account to associate the VM with.
*
*/
public String serviceAccount() {
return this.serviceAccount;
}
/**
* @return The full path of the resource of type TargetProject which represents the Compute Engine project in which to create this VM.
*
*/
public String targetProject() {
return this.targetProject;
}
/**
* @return The name of the VM to create.
*
*/
public String vmName() {
return this.vmName;
}
/**
* @return The zone in which to create the VM.
*
*/
public String zone() {
return this.zone;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ComputeEngineTargetDefaultsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List additionalLicenses;
private AppliedLicenseResponse appliedLicense;
private String bootOption;
private ComputeSchedulingResponse computeScheduling;
private String diskType;
private String hostname;
private Map labels;
private String licenseType;
private String machineType;
private String machineTypeSeries;
private Map metadata;
private List networkInterfaces;
private List networkTags;
private Boolean secureBoot;
private String serviceAccount;
private String targetProject;
private String vmName;
private String zone;
public Builder() {}
public Builder(ComputeEngineTargetDefaultsResponse defaults) {
Objects.requireNonNull(defaults);
this.additionalLicenses = defaults.additionalLicenses;
this.appliedLicense = defaults.appliedLicense;
this.bootOption = defaults.bootOption;
this.computeScheduling = defaults.computeScheduling;
this.diskType = defaults.diskType;
this.hostname = defaults.hostname;
this.labels = defaults.labels;
this.licenseType = defaults.licenseType;
this.machineType = defaults.machineType;
this.machineTypeSeries = defaults.machineTypeSeries;
this.metadata = defaults.metadata;
this.networkInterfaces = defaults.networkInterfaces;
this.networkTags = defaults.networkTags;
this.secureBoot = defaults.secureBoot;
this.serviceAccount = defaults.serviceAccount;
this.targetProject = defaults.targetProject;
this.vmName = defaults.vmName;
this.zone = defaults.zone;
}
@CustomType.Setter
public Builder additionalLicenses(List additionalLicenses) {
this.additionalLicenses = Objects.requireNonNull(additionalLicenses);
return this;
}
public Builder additionalLicenses(String... additionalLicenses) {
return additionalLicenses(List.of(additionalLicenses));
}
@CustomType.Setter
public Builder appliedLicense(AppliedLicenseResponse appliedLicense) {
this.appliedLicense = Objects.requireNonNull(appliedLicense);
return this;
}
@CustomType.Setter
public Builder bootOption(String bootOption) {
this.bootOption = Objects.requireNonNull(bootOption);
return this;
}
@CustomType.Setter
public Builder computeScheduling(ComputeSchedulingResponse computeScheduling) {
this.computeScheduling = Objects.requireNonNull(computeScheduling);
return this;
}
@CustomType.Setter
public Builder diskType(String diskType) {
this.diskType = Objects.requireNonNull(diskType);
return this;
}
@CustomType.Setter
public Builder hostname(String hostname) {
this.hostname = Objects.requireNonNull(hostname);
return this;
}
@CustomType.Setter
public Builder labels(Map labels) {
this.labels = Objects.requireNonNull(labels);
return this;
}
@CustomType.Setter
public Builder licenseType(String licenseType) {
this.licenseType = Objects.requireNonNull(licenseType);
return this;
}
@CustomType.Setter
public Builder machineType(String machineType) {
this.machineType = Objects.requireNonNull(machineType);
return this;
}
@CustomType.Setter
public Builder machineTypeSeries(String machineTypeSeries) {
this.machineTypeSeries = Objects.requireNonNull(machineTypeSeries);
return this;
}
@CustomType.Setter
public Builder metadata(Map metadata) {
this.metadata = Objects.requireNonNull(metadata);
return this;
}
@CustomType.Setter
public Builder networkInterfaces(List networkInterfaces) {
this.networkInterfaces = Objects.requireNonNull(networkInterfaces);
return this;
}
public Builder networkInterfaces(NetworkInterfaceResponse... networkInterfaces) {
return networkInterfaces(List.of(networkInterfaces));
}
@CustomType.Setter
public Builder networkTags(List networkTags) {
this.networkTags = Objects.requireNonNull(networkTags);
return this;
}
public Builder networkTags(String... networkTags) {
return networkTags(List.of(networkTags));
}
@CustomType.Setter
public Builder secureBoot(Boolean secureBoot) {
this.secureBoot = Objects.requireNonNull(secureBoot);
return this;
}
@CustomType.Setter
public Builder serviceAccount(String serviceAccount) {
this.serviceAccount = Objects.requireNonNull(serviceAccount);
return this;
}
@CustomType.Setter
public Builder targetProject(String targetProject) {
this.targetProject = Objects.requireNonNull(targetProject);
return this;
}
@CustomType.Setter
public Builder vmName(String vmName) {
this.vmName = Objects.requireNonNull(vmName);
return this;
}
@CustomType.Setter
public Builder zone(String zone) {
this.zone = Objects.requireNonNull(zone);
return this;
}
public ComputeEngineTargetDefaultsResponse build() {
final var o = new ComputeEngineTargetDefaultsResponse();
o.additionalLicenses = additionalLicenses;
o.appliedLicense = appliedLicense;
o.bootOption = bootOption;
o.computeScheduling = computeScheduling;
o.diskType = diskType;
o.hostname = hostname;
o.labels = labels;
o.licenseType = licenseType;
o.machineType = machineType;
o.machineTypeSeries = machineTypeSeries;
o.metadata = metadata;
o.networkInterfaces = networkInterfaces;
o.networkTags = networkTags;
o.secureBoot = secureBoot;
o.serviceAccount = serviceAccount;
o.targetProject = targetProject;
o.vmName = vmName;
o.zone = zone;
return o;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy